GET ELEMENT IN JAVASCRIPT
How To Select Element In The DOM
In DOM introduction section we have seen how the DOM is structured as a tree of objects called nodes, and that node can be element, text or comment. We also get to know that we can modify DOM structure or can change any node but to modify something we must first select it.
In this section, we will come across multiple ways to select a node within the DOM.
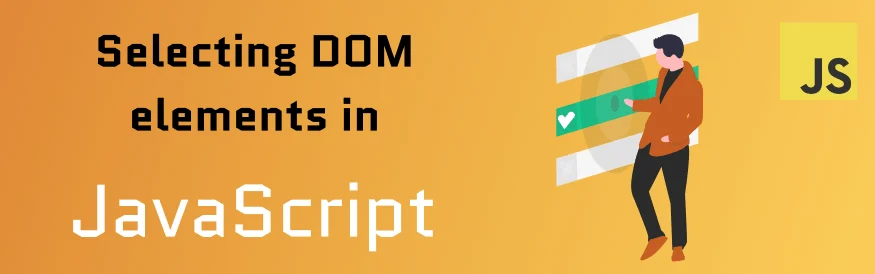
Here is the list of few methods in javascript which is used to select nodes within the DOM:
Let us now discuss each and every method in detail.
1. getElementById
The getElementById()
is the most popular and frequently used DOM method to select HTML elements since IDs are required to be unique so it is a powerful way to select a node within the document.
The getElementById()
method returns an element whose id is matched with the passed id value within the method.
Syntax:
const element = document.getElementById(ID);
To select the element, the "id" of the element is passed in the argument of the method.
If an element with a given id is present in the document
then it returns the element else it returns null.
// selecting element
const para = document.getElementById("id1");
function changeColor(myColor) {
para.style.color = myColor;
}
Try It
Output:
Change the color.
In the above example first, we select the element using getElementById
method, then we use change style of an element using javascript.
2. getElementsByClassName
The getElementsByClassName()
method is another way to select elements within the document. It returns an array of all the child elements which have given class name(s).
This method can be applied on a document or on any specific element of the document, when applied on any element like <div>
, <article>
, etc then only child element of the element having given class name is returned, while when applied on the document
then all the elements with the given class name within the document is returned.
const elements = document.getElementsByClassName(names); or const elements = rootElement.getElementsByClassName(names);
Since this method returns all the elements with specified class names in the form of an array, you can access those elements by its index value in the array.
getElementsByClassName Example
Getting all element with class name 'blue'.
// Selecting all elements with class name 'blue'
const elements = document.getElementsByClassName("blue");
function changeColor() {
// looping through all elements and changing color
for (let i = 0; i < elements.length; i++) {
elements[i].style.color = "red";
}
}
Try It
Getting all element with both classes 'big' and 'blue'.
// Selecting all elements with class name 'big' and 'blue'
const elements = document.getElementsByClassName("big blue");
function changeColor() {
// looping through all elements and changing color
for (let i = 0; i < elements.length; i++) {
elements[i].style.color = "red";
}
}
Try It
Getting all element that have class 'blue', inside an element that has the ID of 'color'.
// Selecting all elements with class name 'blue' which is inside 'color' id
const elements = document.getElementById("color").getElementsByClassName("blue");
console.log(elements);
function changeColor() {
// looping through all elements and changing color
for (let i = 0; i < elements.length; i++) {
elements[i].style.color = "red";
}
}
Try It
3. getElementsByTagName
The getElementsByTagName()
selects and returns all HTML elements with the given tag name in form of an array.
Note: The collection returned by the function is live HTMLCollection, means collection gets updated automatically with the DOM tree.
The method either can be used on the document itself or can be used on some element like <div>
, when applied on the document then all the element with given tag name is selected while when applied on some element then all its child element with a given name is selected but not itself.
const elements = document.getElementsByTagName(); or const elements = rootElement.getElementsByTagName();
If you want to make some change to all specific tags then it is useful.
// Selecting all elements with tag name
const elements = document.getElementsByTagName('div');
console.log(elements);
function changeColor() {
// looping through all elements and changing color
for (let i = 0; i < elements.length; i++) {
elements[i].style.color = "red";
}
}
Try It
4. querySelector
The querySelector()
method is another method to select unique element from the document. This method selects the element on the basic of a valid CSS selectors string.
querySelector()
method uses CSS selector string as argument to select the element. You can select all those elements that can be selected by CSS using CSS selector string.
const element = document.querySelector(selector);
- selector=".class1" - will select first element having this classname
- selector="#id1" - will select the first element with this id
- selector=".class1.class2" - will select first element having both the classes
- selector=".class1 .class2" - will select the first elements with class2 having a parent element with 'class1'
- selector="[input=text]" - will select the first elements with input type text
It returns the first element that matches the CSS selector string passes as an argument. If no matches are found then null
is returned.
Note: The CSS pseudo-elements will never return any elements
// Selecting element which matches CSS selector string
const element = document.querySelector('.demo');
function changeColor() {
element.style.color = "red";
}
Try It
Working with more complex CSS selector for with querySelector
method.
// Selecting element which matches CSS selector string
const element = document.querySelector('#sun .earth .moon p');
function changeColor() {
element.style.color = "red";
}
Try It
5. querySelectorAll
The querySelectorAll()
method is same as querySelector()
method. But instead of selecting only 1st element that matches the CSS selector querySelectorAll
selects all the element matching the string and return as a collection.
const elements = document.querySelectorAll();
Any valid CSS string can be used in this method.
If string matches then its return collection of HTML elements matching the string else return null.
// Selecting element which matches CSS selector string
const elements = document.querySelectorAll('#sun p');
console.log(elements);
function changeColor() {
for (let i = 0; i < elements.length; i++) {
elements[i].style.color = "red";
}
}
Try It