JAVASCRIPT DISPLAY OUTPUT
In this tutorial, we will use different methods is javascript property to display output/result on the screen or in console.
Different Ways To Display Output using Javascript
There are various methods to display output in javascript.
We are going to use following methods for this purpose:
- element.innerHTML
- document.write()
- alert()
- console.log()

1. element.innerHTML
You can use the innerHTML
property of an HTML element to insert text into the element.
Before applying the innerHTML
property, you need to select the element first.
Javascript has many methods to select an element from the HTML document. Some of these are:
- Accessing elements using id, using getElementById("id") method.
- Accessing elements using CSS selectors, using querySelector("class") method.
- Accessing elements using tag name, using getElementSByTagName("tag") method.
Once you have selected the element, you can use the innerHTML
property to insert text into the element.
Example
//getting access to "p" tag by "getElementById"
var element = document.getElementById("output");
//innerHTML is a property of "element"
element.innerHTML = "Hello, World!";
Try It
2. Output Using document.write()
document.write()
is a method using which you can write something on a webpage directly using javascript.
Note: document.write()
method overwrites all of the things inside an HTML document, So be careful while using it. Never use this method after the document is loaded (ex. using it in any event trigger) otherwise it will overwrite all.
3. Output Using document.alert()
document.alert()
or alert()
method is used to show some output or result. It creates a pop up showing the result.
When an alert pop up appears on the screen then any program after the alert()
method is not executed until the pop up is closed.
4. Output Using console.log
console.log
is used to show results in the console. console is used by developers for debugging purposes.
To open console press ctrl + shift + i or f12 then switch to console tab.
To show results in console write console.log(your_output_here)
. Using it you can output all kinds of object in console like number, string, array, etc.
Example
console.log("Hello, World!");
console.log(123);
console.log(document);
console.log("Hello World", 123); // multiple output can also be shown separating by comma
Try It
Output:
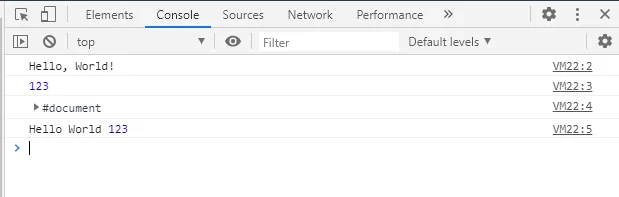