Sort Array In JavaScript - Complete Guide
In this tutorial, we are going to learn how to sort an array in JavaScript. We will learn how to sort an array in ascending, descending, or sort even objects.
- sort Method in JavaScript
- Sort array of numbers
- Sort array in descending order
- Sort array of strings
- Sort array of objects
Table Of Contents
sort Method In JavaScript
The sort() method is used to sort an array in ascending order by changing the positions of elements within the array itself. The method sorts the array and also returns the sorted array.
The sort()
is a built-in method of JavaScript. It converts the array element into a string and then compares their UTF-16 code point values.
Let's see an example of the sort method.
Example
// Array of strings
let lang = ["HTML", "CSS", "Javascript", "SQL", "Python", "Dart", "Basic"];
// Using sort method
lang.sort();
console.log(lang);
Try It
Working Of sort Method
The sort()
method converts the array element into a string and then compare their UTF-16 code point values and position them in ascending order.
Note: UTF-16 code point values are the same as ASCII code point values, but the Unicode standard defines the code point values of characters in the range U+0000 to U+FFFF
Suppose we have 2 numbers 5 and 1 in an array. According to the UTF-16 code point values, 5 is greater than 1. So, the sort()
method will move 5 after 1.
Example
let num = [5, 1];
// Using sort method
num.sort();
console.log(num); // [1, 5]
// similarly taking more than 2 numbers
let num2 = [5, 1, 3, 2, 4];
num2 = num2.sort();
console.log(num2); // [1, 2, 3, 4, 5]
Here comes the tricky part! What happens if we also have 2 or higher digit numbers in the array, like 12, 56, 263, etc?
Take a test array: arr = [5, 2, 12, 56, 263, 1]
and apply arr.sort()
You would be thinking that it will be sorted like:
arr = [1, 2, 5, 12, 56, 263]
❌
but it will be sorted like:
arr = [1, 12, 2, 263, 5, 56]
✅
Thinking how! 🤔
This happens because sort() method first compare the first digit of array element and sort according to that. If the first digit is the same, then it will compare the second digit and sort according to that. And so on.
Due to this reason, 12 is sorted before 2 because when you compare the first digit of both numbers then 1 comes before 2. So 12 is sorted before 2.
Applying the same logic you can understand why 263 is before 5 and 56.
Then what should you do to sort the array elements in increasing order of their number value?
To fix this you need to pass a compare function in the sort method.
Compare Function
The sort()
method accepts a function as an argument. It is called compare function.
array.sort(compareFunction)
The compare function determines the order of sorting the elements. It takes two arguments and returns a number. The number returned is used to sort the array.
function compareFunction(a, b) {
// ...
}
The 2 arguments accepted by the compare function are generally names as 'a' and 'b'.
At any moment of sorting the array, the compare function is called with two elements of the array where 'a' is a current element and 'b' is the next element in the array.
These 2 elements are compared and the following actions are taken:
- If compareFunction(a, b) returns less than 0, then
sort()
method sorts a at lower index than b. Simply a will come before b. - If compareFunction(a, b) returns equal to 0, then
sort()
method leave the element positions as it is. - If compareFunction(a, b) returns greater than 0, then
sort()
method sorts a at greater index than b. Simply a will come after b.
Let's see an example:
Example
let numbers = [5, 1, 30, 23, 145, 2];
// compare function
function compare(a, b) {
if (a > b) {
return 1;
} else if (a < b) {
return -1;
} else {
return 0;
}
}
// using sort method with compare function
numbers.sort(compare);
// Output
console.log(numbers);
Try It
Sort Array Of Numbers
Now that we know how to use compare function, let's see how to sort an array of numbers.
Use the sort()
method and pass a compare function to it.
It is not necessary to use an external function. You can also use an anonymous function.
Let's sort an array of numbers using the sort()
method.
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// compare function
function compare(a, b) {
if(a > b) {
return 1;
} else if(a < b) {
return -1;
} else {
return 0;
}
}
// sort method
arr.sort(compare);
//Output
console.log(arr);
Try It
# Shorten the compare function
If array elements are numbers and are not Infinity
then instead of returning on the base of 3 conditions as in the above example simply return a - b
which will be equivalent to the above 3 conditions.
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// compare function
function compare(a, b) {
return a - b;
}
// sort method
arr.sort(compare);
// Output
console.log(arr);
Try It
Example explained:
Let's check for the first 3 elements of the array. i.e [61, 8, 20]
compareFunction(61 ,8); // return 53, a > b compareFunction(61 ,20); // return 41, a > b compareFunction(8 ,20); // return -12, a < b
# Compare function as function expression
You can also define function expression within the sort
method.
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// sort method
// compare function as function expression
arr.sort(function (a, b) {
return a - b;
});
console.log(arr);
Try It
# Compare function as arrow function
Or you can define compare function using an arrow function.
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// arrow compare function
const compare = (a, b) => (a - b);
arr.sort(compare);
console.log(arr);
Try It
Or simply you can write. (arrow function as function expression)
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// sort method using arrow function
arr.sort((a, b) => a - b);
console.log(arr);
Sort Array In Descending Order
How do you think about sorting an array in descending order?
One way to do this is to sort the array in ascending order and then reverse the array using the reverse()
method.
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// sort method using arrow function
arr.sort((a, b) => a - b);
// reverse the sorted array
arr.reverse();
// Output
console.log(arr);
Try It
But we can do this with a simple modification in compare function.
Just change the return a - b
to return b - a
.
Example
let arr = [61, 8, 20, 5, 1, 11, 9, 3];
// sort method using arrow function
arr.sort((a, b) => b - a);
// Output
console.log(arr);
Try It
Sort Array Of String
Sorting string of array is very simple using JavaScript, just apply sort()
method on the array of string and by default, it will sort an array of a string in ascending order.
Example
let lang = ["HTML", "CSS", "Javascript", "SQL", "Python"];
// applying sort method
lang.sort();
console.log(lang);
Try It
# Reverse sort
To compare strings in reverse order use compare function. You need to change the logic of compare function and pass it to the sort
method as shown in the code below.
Example
let lang = ["HTML", "CSS", "Javascript", "SQL", "Python"];
// reverse sort
function compare(a, b) {
return b - a;
}
lang.sort(compare);
console.log(lang);
Try It
Sorting non-ASCII characters
To sort strings with non-ASCII characters, i.e. String from other languages é, è, a, ä, etc. Use String.localeCompare
.
This function can compare non-ASCII characters so they appear in the right order.
Example
// array of non-ASCII strings
let arr = ["zèbree", "communiqué", "éclair", "réservé", "écureuill"];
// compare function to sort non-ASCII characters
function compare(a, b) {
return a.localeCompare(b)
}
arr.sort(compare);
console.log(arr);
Try It
Javascript sort array of objects
To sort an array of objects you can not directly use the sort()
method. You will have to use compare function to decide the order of objects depending on different property values.
Create a compare function and compare the property values of two objects. This compare function will be passed to the sort()
method.
Here to sort an array of objects according to the age of an employee you can use a.age - b.age
to compare 2 objects. See the complete code below.
Example
let employee = [
{ name: "John", age: 35, joined: "Wed Feb 03 2011" },
{ name: "Mike", age: 28, joined: "Mon Feb 05 2001" },
{ name: "Herry", age: 32, joined: "Tue May 22 2012" },
{ name: "Sara", age: 24, joined: "Wed Aug 15 2018" },
{ name: "David", age: 33, joined: "Mon Oct 27 2008" }
]
function compare(a, b) {
return a.age > b.age;
}
employee.sort(compare);
console.log(employee);
// use console.table for better output look
Try It
Output using console.table
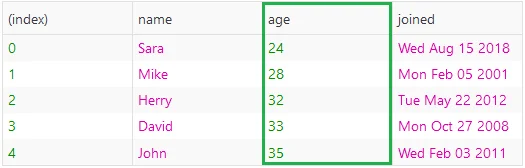
javascript sort array of objects by key (joining date)
Let's sort the object on the basis of the joining date property.
Since 'joining' in the object is a string value, first convert the value of the joining string value to a date object and then use this in compare function to return value.
Example
let employee = [
{ name: "John", age: 35, joined: "Wed Feb 03 2011" },
{ name: "Mike", age: 28, joined: "Mon Feb 05 2001" },
{ name: "Herry", age: 32, joined: "Tue May 22 2012" },
{ name: "Sara", age: 24, joined: "Wed Aug 15 2018" },
{ name: "David", age: 33, joined: "Mon Oct 27 2008" }
]
function compare(a, b) {
return new Date(a.joined) > new Date(b.joined);
}
employee.sort(compare);
console.log(employee);
// use console.table for better output look
Try It
Output using console.table
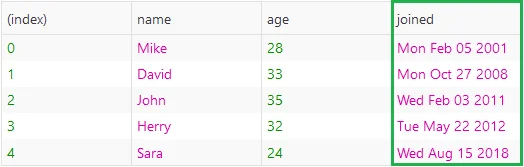
Sorting objects by string property
To sort the employee array by their name convert employee name to lowercase and pass it in compare function.
Example
let employee = [
{ name: "John", age: 35, joined: "Wed Feb 03 2011" },
{ name: "Mike", age: 28, joined: "Mon Feb 05 2001" },
{ name: "Herry", age: 32, joined: "Tue May 22 2012" },
{ name: "Sara", age: 24, joined: "Wed Aug 15 2018" },
{ name: "David", age: 33, joined: "Mon Oct 27 2008" }
];
// compare function
function compare(a, b) {
let nameA = a.name, nameB = b.name;
if (nameA > nameB) return 1;
if (nameA < nameB) return -1;
return 0;
}
// using sort method
employee.sort(compare);
// Output
console.table(employee);
Try It
Conclusion
JavaScript sort() method can be used to sort an array of numbers, strings, or objects. It can also be used to sort an array of objects by a property value. The compare function is used to decide the order of objects depending on different property values.
We have seen lots of examples that clarify our understanding of the sort() method.