JAVASCRIPT ASSIGNMENT OPERATORS
In this tutorial, you will learn about all the different assignment operators in javascript and how to use them in javascript.
Assignment Operators
In javascript, there are 16 different assignment operators that are used to assign value to the variable. It is shorthand of other operators which is recommended to use.
The assignment operators are used to assign value based on the right operand to its left operand.
The left operand must be a variable while the right operand may be a variable, number, boolean, string, expression, object, or combination of any other.
One of the most basic assignment operators is equal =, which is used to directly assign a value.
Example
let a = 10;
console.log(a);
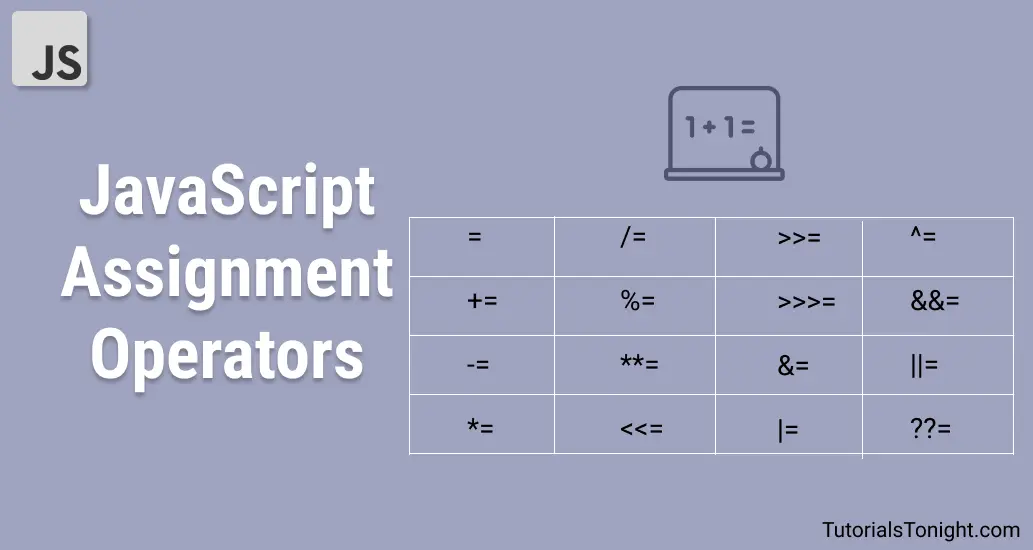
Assignment Operators List
Here is the list of all assignment operators in JavaScript:
In the following table if variable a
is not defined then assume it to be 10.
Operator | Description | Example | Equivalent to |
---|---|---|---|
= | Assignment operator | a = 10 | a = 10 |
+= | Addition assignment operator | a += 10 | a = a + 10 |
-= | Subtraction assignment operator | a -= 10 | a = a - 10 |
*= | Multiplication assignment operator | a *= 10 | a = a * 10 |
/= | Division assignment operator | a /= 10 | a = a / 10 |
%= | Remainder assignment operator | a %= 10 | a = a % 10 |
**= | Exponentiation assignment operator | a **= 2 | a = a ** 2 |
<<= | Left shift assignment | a <<= 1 | a = a << 1 |
>>= | Right shift assignment | a >>= 2 | a = a >> 2 |
>>>= | Unsigned right shift assignment | a >>>= 1 | a = a >>> 1 |
&= | Bitwise AND assignment | a &= 4 | a = a & 4 |
|= | Bitwise OR assignment | a |= 2 | a = a | 2 |
^= | Bitwise XOR assignment | a ^= 5 | a = a ^ 5 |
&&= | Logical AND assignment | a &&= 3 | a = a && 3 |
||= | Logical OR assignment | a ||= 4 | a = a || 4 |
??= | Logical nullish assignment | a ??= 2 | a = a ?? 2 |
Assignment operator
The assignment operator = is the simplest value assigning operator which assigns a given value to a variable.
The assignment operators support chaining, which means you can assign a single value in multiple variables in a single line.
Addition assignment operator
The addition assignment operator += is used to add the value of the right operand to the value of the left operand and assigns the result to the left operand.
On the basis of the data type of variable, the addition assignment operator may add or concatenate the variables.
Example
let a = 10;
let b = 'hello';
let c = true;
console.log(a += 20); // addition
console.log(b += ' world!'); // concatenation
console.log(c += true); // boolean + boolean -> addition
Try It
Subtraction assignment operator
The subtraction assignment operator -= subtracts the value of the right operand from the value of the left operand and assigns the result to the left operand.
If the value can not be subtracted then it results in a NaN
.
Example
let a = 10;
let b = 'hello';
let c = true;
console.log(a -= 20); // -10
console.log(b -= 'world'); // NaN
console.log(c -= true); // 0
Try It
Multiplication assignment operator
The multiplication assignment operator *= assigns the result to the left operand after multiplying values of the left and right operand.
Example
let a = 10;
let b = 'hello';
let c = true;
console.log(a *= 2); // 20
console.log(b *= 5); // NaN
console.log(c *= true); // 1
Try It
Division assignment operator
The division assignment operator /= divides the value of the left operand by the value of the right operand and assigns the result to the left operand.
Example
let a = 10;
let b = 'hello';
let c = true;
console.log(a /= 2); // 5
console.log(b /= 5); // NaN
console.log(c /= false); // Infinity
Try It
Remainder assignment operator
The remainder assignment operator %= assigns the remainder to the left operand after dividing the value of the left operand by the value of the right operand.
Example
let a = 10;
let b = 'hello';
let c = true;
console.log(a %= 7); // 3
console.log(b %= 5); // NaN
console.log(c %= 1); // 0
Try It
Exponentiation assignment operator
The exponential assignment operator **= assigns the result of exponentiation to the left operand after exponentiating the value of the left operand by the value of the right operand.
Example
let a = 10;
let b = 'hello';
let c = true;
console.log(a **= 3); // 100
console.log(b **= 5); // NaN
console.log(c **= 10); // 1
Try It
Left shift assignment
The left shift assignment operator <<= assigns the result of the left shift to the left operand after shifting the value of the left operand by the value of the right operand.
Example
let a = 10;
// 10 = 00000000000000000000000000001010
let b = 2;
console.log(a <<= b);
console.log(a <<= 5);
Try It
Right shift assignment
The right shift assignment operator >>= assigns the result of the right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Unsigned right shift assignment
The unsigned right shift assignment operator >>>= assigns the result of the unsigned right shift to the left operand after shifting the value of the left operand by the value of the right operand.
Bitwise AND assignment
The bitwise AND assignment operator &= assigns the result of bitwise AND to the left operand after ANDing the value of the left operand by the value of the right operand.
Bitwise OR assignment
The bitwise OR assignment operator |= assigns the result of bitwise OR to the left operand after ORing the value of left operand by the value of the right operand.
Bitwise XOR assignment
The bitwise XOR assignment operator ^= assigns the result of bitwise XOR to the left operand after XORing the value of the left operand by the value of the right operand.
Logical AND assignment
The logical AND assignment operator &&= assigns value to left operand only when it is truthy.
Note: A truthy value is a value that is considered true when encountered in a boolean context.
Example
let a = 2;
let b = "some string";
let c = false;
let d = 0;
console.log(a &&= 50); // assigned
console.log(b &&= "yes truthy"); // assigned
console.log(c &&= 23); // not assigned
console.log(d &&= 80); // not assigned
Try It
Logical OR assignment
The logical OR assignment operator ||= assigns value to left operand only when it is falsy.
Note: A falsy value is a value that is considered false when encountered in a boolean context.
Example
let a = 2;
let b = "some string";
let c = false;
let d = 0;
console.log(a ||= 50); // not assigned
console.log(b ||= "truthy"); // not assigned
console.log(c ||= "yes falsy"); // assigned
console.log(d ||= 80); // assigned
Try It
Logical nullish assignment
The logical nullish assignment operator ??= assigns value to left operand only when it is nullish (null
or undefined
).
Example
let a = null;
let b;
let c = 20;
let d = 0;
console.log(a ??= 50); // assigned
console.log(b ??= "hello world!"); // assigned
console.log(c ??= 100); // not assigned
console.log(d ??= 80); // not assigned
Try It