2 Ways to Reverse forEach Loop in JavaScript
In this article, you will learn 2 different ways to reverse forEach loop in JavaScript.
forEach reverse JavaScript
Ideally, forEach loop in JavaScript is intended to iterate over an array in the forward direction. But, what if you want to iterate over an array in the reverse direction?π€
Well, you can do that by using the reverse() method on the array. Let's see how.
-
First, create an array of numbers or pick any array you want to iterate over.
const numbers = [1, 2, 3, 4, 5];
-
Then, reverse the array using the reverse() method.
numbers.reverse();
-
Finally, iterate over the reversed array using the forEach loop.
numbers.forEach((number) => { console.log(number); }); // π: 5, 4, 3, 2, 1
You can see above that the forEach loop outputs the element of the array in reverse order.
Here is a working example for this.
Example
// reverse forEach loop in JavaScript
const numbers = [1, 2, 3, 4, 5];
numbers.reverse().forEach((number) => {
console.log(number);
});
// π: 5, 4, 3, 2, 1
const str = 'Hello';
str.split('').reverse().forEach((char) => {
console.log(char);
});
// π: o, l, l, e, H
But there is an issue with this approach. The reverse() method reverses the original array. So, if you want to use the original array again, you will have to create a copy of it.
Make a copy of the array using the slice() method and then reverse it.
Example
// reverse forEach loop in JavaScript
const numbers = [1, 2, 3, 4, 5];
numbers.slice().reverse().forEach((number) => {
console.log(number);
});
// π: 5, 4, 3, 2, 1
// array is not reversed
console.log(numbers)
That's it! You have successfully reversed the forEach loop in JavaScript.π
Another way to reverse forEach
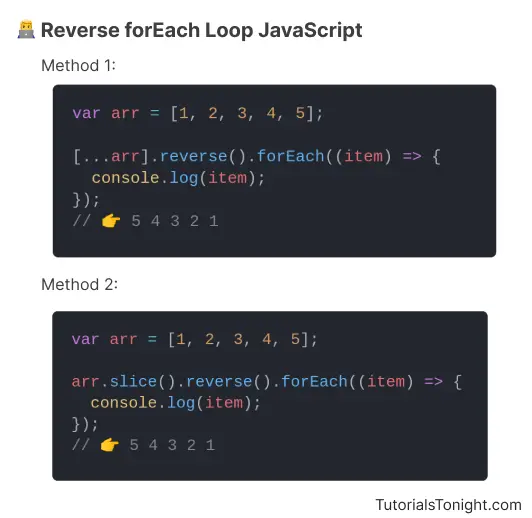
Use the spread operator to reverse the array and then iterate over it using the forEach loop.
-
Use the spread operator to make a copy of the array and apply the reverse() method on it.
[...numbers].reverse();
-
Finally, iterate over the reversed array using forEach loop.
[...numbers].reverse().forEach((number) => { console.log(number); }); // π: 5, 4, 3, 2, 1
Here is a working example for this.
Example
// reverse forEach loop in JavaScript
const numbers = [1, 2, 3, 4, 5];
[...numbers].reverse().forEach((number) => {
console.log(number);
});
// π: 5, 4, 3, 2, 1
Conclusion
In this article, you have learned 2 different ways to reverse forEach loop in JavaScript. You can use any of them as per your requirement.
Hope you liked this article. Check out other articles to learn more about JavaScript.
Happy Coding!π