Star Pattern In PHP (15 examples)
In this tutorial, we will learn how to print a star pattern in PHP. We will see 15 different types of star pattern in PHP with descriptions and complete code.
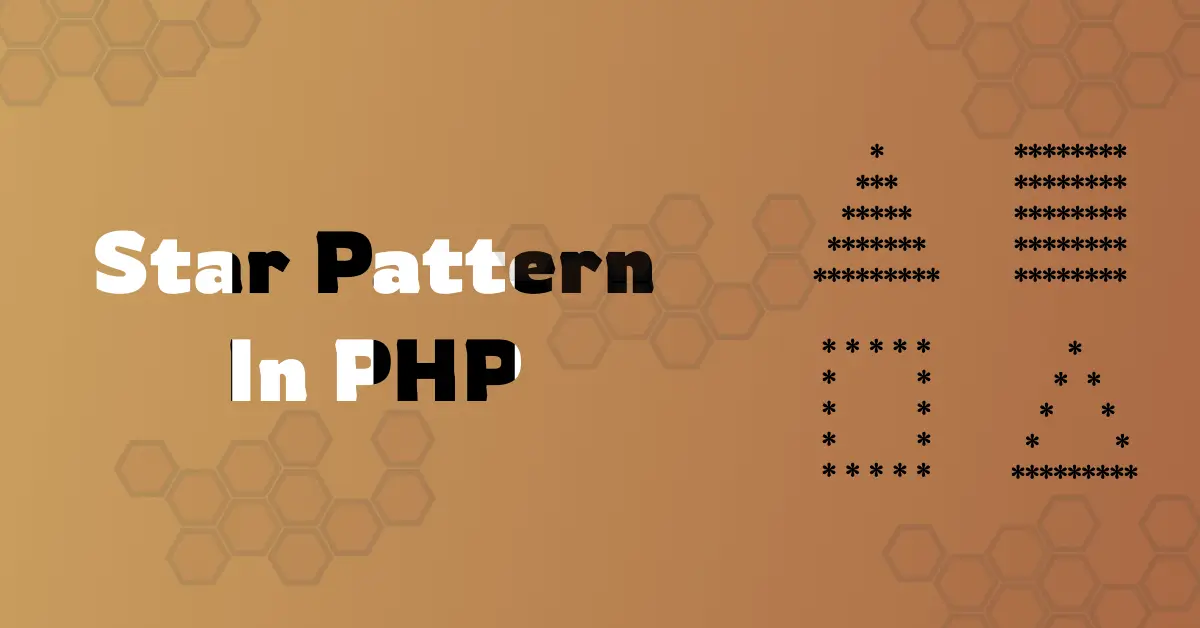
- square pattern in PHP
- Hollow square pattern
- left triangle star pattern in PHP
- right triangle star pattern in PHP
- Downward triangle star pattern in PHP
- Hollow triangle star pattern in PHP
- Pyramid star pattern in PHP
- Reverse pyramid star pattern in PHP
- Hollow pyramid star pattern in PHP
- Diamond star pattern in PHP
- Hollow diamond star pattern in PHP
- Hourglass star pattern
- Right Pascal star pattern
- Left Pascal star pattern
- Heart star pattern
Table Of Contents
1. Square pattern in PHP
The square pattern is the easiest pattern to start with creating patterns in PHP.
This star pattern makes a shape of a square or rectangle with a number of rows and columns.
***** ***** ***** ***** *****
To create a square pattern, we need 2 nested loops. The outer loop will print a number of rows and the inner loop will print the star in each row.
Repeat external loop for times equal to the size of the square, inside this loop, repeat inner loop for times equal to the size of the square and print star. At the end of each row, print a new line.
<?php
// square pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print column
for($j = 0; $j < $size; $j++) {
// print row
echo "*";
}
// change line
echo "<br>";
}
?>
Output:
***** ***** ***** ***** *****
2. Hollow Square pattern
***** * * * * * * *****
This star pattern is a hollow square. It is a square with a hollow inside.
Steps to create a hollow square pattern:
- Repeat the outer loop for times equal to the size of the square.
- Inside this loop, repeat the inner loop for times equal to the size of the square.
- If the outer loop index is 0 or the size of the square - 1, print star.
- Else if the inner loop index is 0 or size of the square - 1, print star, else print space.
- At the end of each row, print a new line using <br>.
<?php
// hollow square pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print column
for($j = 0; $j < $size; $j++) {
// print only star in first and last row
if($i === 0 || $i === $size - 1) {
echo "*";
}
else {
// print star only in first and last position row
if($j === 0 || $j === $size - 1) {
echo "*";
}
else {
// use for space
echo " ";
}
}
}
echo "<br>";
}
?>
Output:
***** * * * * * * *****
3. Left triangle star pattern in PHP
* ** *** **** *****
The triangle start pattern in PHP is quite frequently used in practice. We will create a left triangle star pattern here.
Steps to create a left triangle star pattern:
- Repeat the outer loop for times equal to the size of the triangle.
- Inside this loop, repeat the inner loop for times equal to the index of the outer loop and print star (*).
- At the end of each row, print a new line using <br>.
<?php
// left triangle pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print column
for($j = 0; $j <= $i; $j++) {
echo "*";
}
echo "<br>";
}
?>
Output:
* ** *** **** *****
4. Right triangle star pattern in PHP
* ** *** **** *****
The right triangle star pattern in PHP is another triangle pattern. You can see above the pattern has space in the first column with 1 star, then 2 stars, and so on.
Steps to create a right triangle star pattern:
- Repeat outer loop to print columns.
- Inside this loop, repeat inner loop for times equal to size of the triangle and print star (*).
- At the end of each row, print a new line using <br>.
<?php
// right triangle pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print spaces
for($j = 1; $j < $size - $i; $j++) {
echo " ";
}
// print stars
for($k = 0; $k <= $i; $k++) {
echo "*";
}
echo "<br>";
}
?>
Output:
* ** *** **** *****
5. Downward triangle star pattern in PHP
***** **** *** ** *
You can see above downward triangle star pattern. Here are the steps to create a downward triangle star pattern:
- Create an external loop to print rows.
- Inside this loop, create an inner loop to print columns and repeat the inner loop for times equal to size minus the outer loop index and print star (*).
- At the end of each row, print a new line using <br>.
<?php
// downward triangle pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print stars
for($j = 0; $j < $size - $i; $j++) {
echo "*";
}
echo "<br>";
}
?>
Output:
***** **** *** ** *
6. Hollow triangle star pattern in PHP
* ** * * * * * * ******
Steps to create a hollow triangle star pattern:
- Create an outer loop to print rows.
- Inside this loop, create an inner loop to print columns.
- Inside the inner loop, check if the index of the inner loop does not last then print star (*) at first and last index of the inner loop.
- If last row, print only star (*).
- Break the row using <br>.
<?php
// hollow triangle pattern
$size = 5;
for($i = 1; $i <= $size; $i++) {
for($j = 0; $j < $i; $j++) {
// not last row
if($i != $size) {
if($j == 0 || $j == $i - 1) {
echo "*";
}
else {
echo " ";
}
}
// last row
else {
echo "*";
}
}
echo "<br>";
}
?>
Output:
* ** * * * * * * ******
7. Pyramid star pattern in PHP
* *** ***** ******* *********
The pyramid star pattern is the most famous star pattern. You can see it in the above pattern.
Steps to create a pyramid star pattern:
- Create an external loop to print all rows.
- Inside this loop, we have to create 2 inner loops. One for printing spaces and the other for printing stars.
- Inside the first inner loop, print number of spaces equal to size minus the outer loop index.
- Inside the second inner loop, print number of stars equal to 2 times the outer loop index minus 1.
- At the end of both loop break the row using <br>.
<?php
// pyramid star pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print spaces
for($j = 0; $j < $size - $i - 1; $j++) {
echo " ";
}
// print stars
for($k = 0; $k < 2 * $i + 1; $k++) {
echo "*";
}
echo "<br>";
}
?>
Output:
* *** ***** ******* *********
8. Reverse pyramid star pattern in PHP
********* ******* ***** *** *
The reverse pyramid star pattern is the same as the pyramid star pattern but reverse.
To create this just change the loop order.
<?php
// pyramid star pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print spaces
for($j = 0; $j < $i; $j++) {
echo " ";
}
// print stars
for($k = 0; $k < 2 * ($size - $i) - 1; $k++) {
echo "*";
}
echo "<br>";
}
?>
Output:
********* ******* ***** *** *
9. Hollow pyramid star pattern in PHP
* * * * * * * *********
The hollow pyramid star pattern is a bit complex because you have to deal with outer spaces as well as inner spaces.
Here are the steps to create this:
- Run a loop to print all rows this will be an external loop.
- Inside this loop, we have to create 2 inner loops. One for printing spaces and the other for printing stars.
- The first inner loop will print a number of spaces equal to size minus the outer loop index.
- The second inner loop will run for 2 times the outer loop index minus 1 and print star only at the first and last index of the row.
- Finally break the row using <br>.
<?php
// pyramid star pattern
$size = 5;
for($i = 0; $i < $size; $i++) {
// print spaces
for($j = 0; $j < $size - $i - 1; $j++) {
echo " ";
}
// print stars
for($k = 0; $k < 2 * $i + 1; $k++) {
if($i === 0 || $i === $size - 1) {
echo "*";
}
else {
if($k === 0 || $k === 2 * $i) {
echo "*";
}
else {
echo " ";
}
}
}
echo "<br>";
}
?>
Output:
* * * * * * * *********
10. Diamond star pattern in PHP
* *** ***** ******* ********* ******* ***** *** *
You can see above the diamond star pattern is a combination of the pyramid and reverse pyramid pattern.
If you combine the code of pyramid and reverse pyramid wisely you can create a diamond star pattern.
Here is complete code to create this:
<?php
$size = 5;
// upside pyramid
for ($i = 1; $i <= $size; $i++) {
// printing spaces
for ($j = $size; $j > $i; $j--) {
echo " ";
}
// printing star
for ($k = 0; $k < $i * 2 - 1; $k++) {
echo "*";
}
echo "<br>";
}
// downside pyramid
for ($i = 1; $i <= $size - 1; $i++) {
// printing spaces
for ($j = 0; $j < $i; $j++) {
echo " ";
}
// printing star
for ($k = ($size - $i) * 2 - 1; $k > 0; $k--) {
echo "*";
}
echo "<br>";
}
?>
Output:
* *** ***** ******* ********* ******* ***** *** *
11. Hollow diamond star pattern in PHP
* * * * * * * * * * * * * * * *
You can see above the hollow diamond star pattern it is the same as the diamond star pattern but stars only at the boundary.
Here is complete code to create hollow diamond:
<?php
$size = 5;
// upside pyramid
for ($i = 1; $i <= $size; $i++) {
// printing spaces
for ($j = $size; $j > $i; $j--) {
echo " ";
}
// printing star
for ($k = 0; $k < $i * 2 - 1; $k++) {
if ($k === 0 || $k === 2 * $i - 2) {
echo "*";
}
else {
echo " ";
}
}
echo "<br>";
}
// downside pyramid
for ($i = 1; $i <= $size - 1; $i++) {
// printing spaces
for ($j = 0; $j < $i; $j++) {
echo " ";
}
// printing star
for ($k = ($size - $i) * 2 - 1; $k >= 1; $k--) {
if ($k === 1 || $k === ($size - $i) * 2 - 1) {
echo "*";
}
else {
echo " ";
}
}
echo "<br>";
}
?>
Output:
* * * * * * * * * * * * * * * *
12. Hourglass star pattern in PHP
********* ******* ***** *** * *** ***** ******* *********
You can see above the hourglass star pattern. It is a combination of reverse pyramid and pyramid pattern.
Here is complete code to create hourglass pattern:
<?php
$size = 5;
// reversed pyramid star pattern
for ($i = 0; $i < $size; $i++) {
// printing spaces
for ($j = 0; $j < $i; $j++) {
echo " ";
}
// printing star
for ($k = 0; $k < ($size - $i) * 2 - 1; $k++) {
echo "*";
}
echo "<br>";
}
// pyramid star pattern
for ($i = 2; $i <= $size; $i++) {
// printing spaces
for ($j = $size; $j > $i; $j--) {
echo " ";
}
// printing star
for ($k = 0; $k < $i * 2 - 1; $k++) {
echo "*";
}
echo "<br>";
}
?>
Output:
********* ******* ***** *** * *** ***** ******* *********
13. Right pascal star pattern
* ** *** **** ***** **** *** ** *
The right pascal star pattern can be created using 2 nested loops.
You can see in the above pattern it is a combination of the right triangle star pattern and reversed triangle star pattern together. Here is the complete code for the right pascal star pattern.
<?php
$size = 5;
for ($i = 1; $i <= $size; $i++) {
for ($j = 0; $j < $i; $j++) {
echo "*";
}
echo "<br>";
}
for ($i = 1; $i <= $size - 1; $i++) {
for ($j = 0; $j < $size - $i; $j++) {
echo "*";
}
echo "<br>";
}
?>
Output:
* ** *** **** ***** **** *** ** *
14. Left pascal star pattern
* ** *** **** ***** **** *** ** *
The left pascal star pattern is a mirror image of the right pascal star pattern.
It is bit complex than right pascal star pattern because you have to deal with spaces. Here is complete code to create left pascal star pattern:
<?php
$size = 5;
for ($i = 1; $i <= $size; $i++) {
for ($j = 0; $j < $size - $i; $j++) {
echo " ";
}
for ($k = 0; $k < $i; $k++) {
echo "*";
}
echo "<br>";
}
for ($i = 1; $i <= $size - 1; $i++) {
for ($j = 0; $j < $i; $j++) {
echo " ";
}
for ($k = 0; $k < $size - $i; $k++) {
echo "*";
}
echo "<br>";
}
?>
Output:
* ** *** **** ***** **** *** ** *
15. Heart pattern
*** *** ***** ***** *********** ********* ******* ***** *** *
Heart star pattern is quite complex pattern to create. Here is complete code to create heart star pattern:
<?php
// heart star pattern
$size = 6;
for ($i = $size / 2; $i < $size; $i += 2) {
// print first spaces
for ($j = 1; $j < $size - $i; $j += 2) {
echo " ";
}
// print first stars
for ($j = 1; $j < $i + 1; $j++) {
echo "*";
}
// print second spaces
for ($j = 1; $j < $size - $i + 1; $j++) {
echo " ";
}
// print second stars
for ($j = 1; $j < $i + 1; $j++) {
echo "*";
}
echo "<br>";
}
// lower part
// inverted pyramid
for ($i = $size; $i > 0; $i--) {
for ($j = 0; $j < $size - $i; $j++) {
echo " ";
}
for ($j = 1; $j < $i * 2; $j++) {
echo "*";
}
echo "<br>";
}
?>
Output:
*** *** ***** ***** *********** ********* ******* ***** *** *
Conclusion
In this article, we have discussed 15 star patterns in PHP. We have created a star pattern using the concepts of loops and conditionals.
There are many other star patterns you can create, but these examples are enough to give you a basic understanding of how to use them.
Also, look at star pattern in javascript and star pattern in python.