Converting JavaScript String to Number
Converting string to number is a common task when dealing with data. Most of the time, the data is received as a string, and we need to convert it to a number for further processing.
In this article, we will explore 6 different ways to convert a string to a number in JavaScript.
- Using parseInt() Function
- Using parseFloat() Function
- Unary + Operator
- Using Number() Constructor
- Speed Comparison
Table of Contents
1. Using parseInt() Function
parseInt() function is used to convert a string to an integer. It parses a string and returns an integer.
It takes two parameters:
- string - The string to be parsed.
- radix - An integer between 2 and 36 that represents the base of the string. If not specified, then the default value is 10.
So we can use parseInt() to convert a string to an integer in the following way:
Example
const stringNumber = "42";
// ๐ converting string to number
const number = parseInt(stringNumber);
console.log(number);
// Output: 42
2. Using parseFloat() Function
parseFloat() is another function which you can use to convert a string to a number (floating-point number).
For this you can pass the string to be converted as a parameter to the parseFloat() function.
Example
const stringFloat = "3.14";
// ๐ converting string to number
const floatNumber = parseFloat(stringFloat);
console.log(floatNumber, typeof floatNumber);
// Output: 3.14, number
3. Using Unary + Operator
The + (plus) operator performs multiple tasks in JavaScript. It can be used to add two numbers, concatenate two strings, as well as convert a string to a number.
To convert a string to a number using the + operator, you can simply place a + sign before the string to be converted.
Example
const stringNum = "42";
// ๐ converting string to number
const convertedNum = +stringNum;
console.log(convertedNum);
// Output: 42
4. Using Number() Constructor
The Number() constructor creates a number wrapper object. You can use it to convert a string to a number.
Example
const stringNum = "42";
// ๐ converting string to number
const convertedNum = Number(stringNum);
console.log(convertedNum);
// Output: 42
Speed Comparison
So these were the 4 different ways to convert a string to a number in JavaScript. You can use any of these methods based on your requirements.
But which one is the fastest?
We run a speed test on all the above methods and we found following observations:
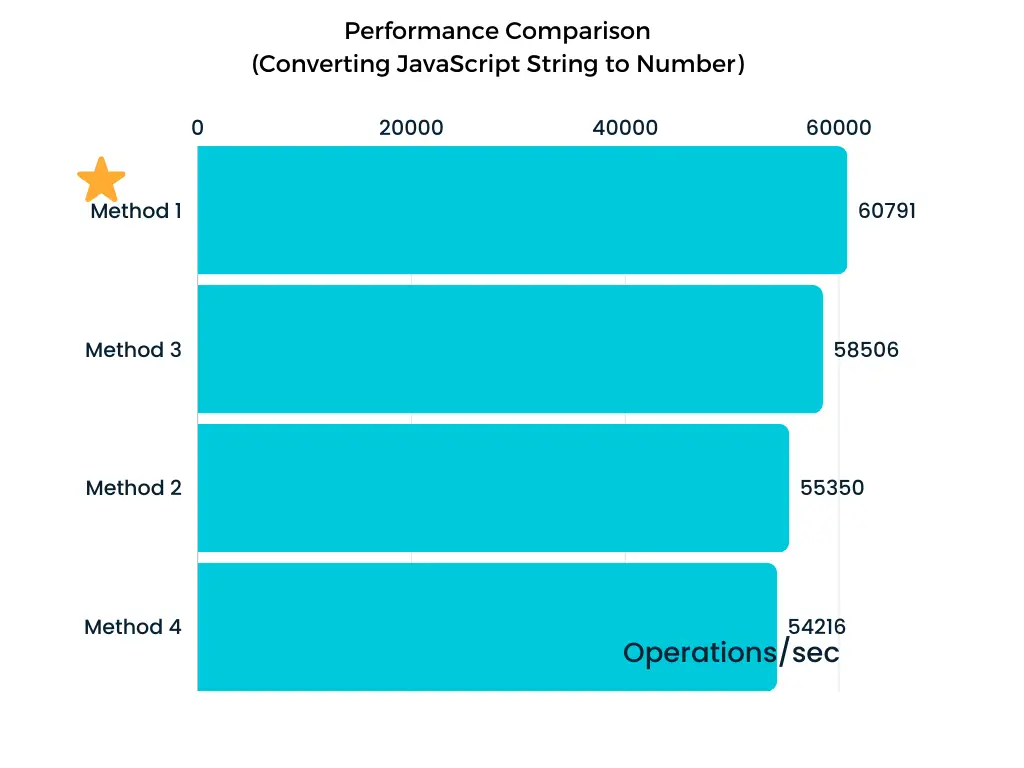
You can clearly see that Method 1 (parseInt) is the fastest method to convert a string to a number. It is followed by Method 3 (unary + operator), Method 2 (parseFloat) and Method 4 (Number constructor).
Happy Coding! ๐