Javascript Function Return Multiple Values
In this article, you are going to learn how JavaScript function return multiple values. We will look at 2 ways to return multiple values from a function in JavaScript with examples.
JavaScript Function Return
Every function in JavaScript returns a value. If you don't specify a return value, the function will return undefined
or in the case of the constructor function, it will return the value of this
.
Any manual return value can also be returned using the return keyword. The return value can be any data type. For example:
- Number -
return 1
- String -
return "Hello"
- Boolean -
return true
- Object -
return {name: "John", age: 30}
- Array -
return [1, 2, 3]
- ...and so on
Generally, we use return only a single value from a function. But in some cases, we need to return multiple values.
If you want to return multiple values from a function, you can use the following 2 ways:
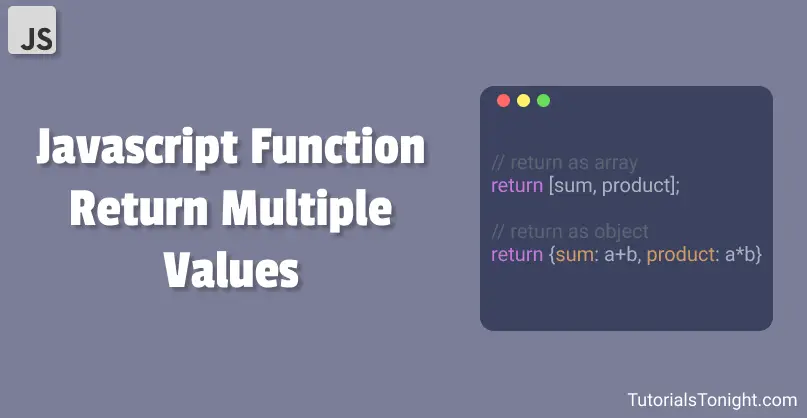
Return Multiple Values as Array
Directly you can't return more than one value from a function. Until and unless you wrap them to a single entity.
One of the ways to return multiple values from a function is to return an array.
The following example returns the sum and product of two numbers as an array.
Example
function operate(a, b) {
var sum = a + b;
var product = a * b;
// return them as an array
return [sum, product];
}
Now, you can call the function and store the returned array in a variable.
Then you can access the values using the index of the array.
Example
function operate(a, b) {
var sum = a + b;
var product = a * b;
// return them as an array
return [sum, product];
}
var result = operate(2, 3);
console.log(result[0]); // 5
console.log(result[1]); // 6
In the latest update in JavaScript, you can also use destructuring to access the values.
This way results can be accessed using the variable name instead of the index.
Example
function operate(a, b) {
var sum = a + b;
var product = a * b;
// return them as an array
return [sum, product];
}
var [sum, product] = operate(2, 3);
console.log(sum); // 5
console.log(product); // 6
Return Multiple Values as Object
Another way to return multiple values from a function is to return an object.
Objects are used to store data in key-value pairs. So, you have to create a key along with the value.
The following example returns the sum and product of two numbers as an object.
Example
function operate(a, b) {
var sum = a + b;
var product = a * b;
// return them as an object
return {sum: sum, product: product};
}
Now, you can call the function and store the returned object in a variable.
Then you can access the values using the key of the object either using dot notation or bracket notation.
Example
function operate(a, b) {
var sum = a + b;
var product = a * b;
// return them as an object
return {sum: sum, product: product};
}
var result = operate(2, 3);
console.log(result.sum); // 5
console.log(result.product); // 6
Even in the case of objects, you can use destructuring to access the values.
In the case of array destructuring, the order of the variables matters. But in the case of object destructuring, the order of the variables doesn't matter. Only the key matters.
Example
function operate(a, b) {
var sum = a + b;
var product = a * b;
// return them as an object
return {sum: sum, product: product};
}
var {sum, product} = operate(2, 3);
console.log(sum); // 5
console.log(product); // 6
Conclusion
In this tutorial, you have learned how to return multiple values from a function in JavaScript and destructuring assignments to unpack the values from the returned array or object.
You have learned two ways to return multiple values from a function.
- Return an array
- Return an object
Now, you can return multiple values from a function in JavaScript.