Check if Object is Empty JavaScript
An object in JavaScript is a collection of key-value pairs where each key is unique.
When there are no key-value pairs in an object, then it is said to be an empty object.
// non-empty object
let obj1 = { name: "John", age: 25 }
// empty object
let obj2 = {}
There are many ways to check if an object is empty or not in JavaScript. Let's see them one by one.
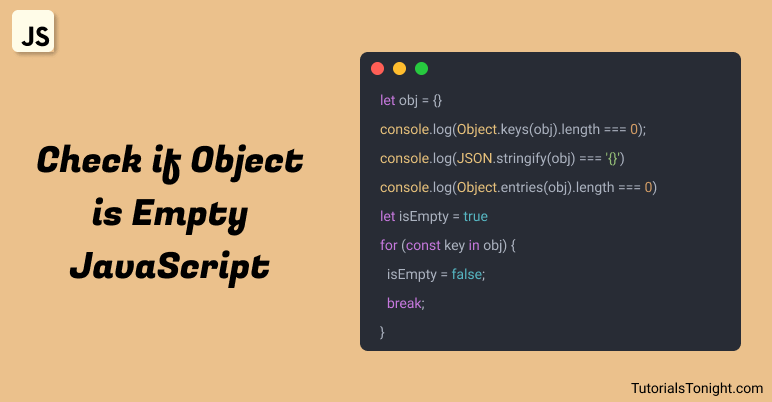
- Quick Solution
- Using Object.keys() Method
- Using JSON.stringify() Method
- Using Object.entries() Method
- Using for...in Loop
- Using Object.getOwnPropertyNames() Method
- Conclusion
Table of Contents
Quick Solution
Here is a quick solution for people who are in a hurry. We will learn everything in detail later in this article.
Example
// Methods to check if an object is empty or not
let obj = {}
// Method 1
if (Object.keys(obj).length === 0 && obj.constructor === Object) {
console.log("1: Object is empty")
}
// Method 2
if (JSON.stringify(obj) === '{}') {
console.log("2: Object is empty")
}
// Method 3
if (Object.entries(obj).length === 0 && obj.constructor === Object) {
console.log("3: Object is empty")
}
// Method 4
let isEmpty = true
for (const key in obj) {
isEmpty = false;
break;
}
console.log("4: Object is empty: " + isEmpty);
1. Using Object.keys() Method
The Object.keys() is a static object method that returns an array of a given object's own enumerable property names.
For example:
let obj = { name: "John", age: 25 }
console.log(Object.keys(obj)) // ["name", "age"]
For an empty object, it returns an empty array.
So to check if an object is empty or not, you can check the length of the array returned by the Object.keys() method. If the length is 0, then the object is empty.
Example
let obj = {}
if (Object.keys(obj).length === 0) {
console.log("Object is empty")
} else {
console.log("Object is not empty")
}
Problem with this method - This method only checks for enumerable properties. If the object has any non-enumerable property, then this method will not work. Also, check for falsy values.
So correct way to check if an object is empty is as follows:
Example
let obj = {}
if (obj
&& Object.keys(obj).length === 0
&& obj.constructor === Object
) {
console.log("Object is empty")
} else {
console.log("Object is not empty")
}
2. Using JSON.stringify() Method
The JSON.stringify() is JSON object method that converts a JavaScript object or value to a JSON string.
When an empty object is passed to this method, it returns "{}" as a string.
So to check whether an object is empty or not, you can check if the string is returned by the JSON.stringify() method is equal to "{}" or not.
Example
let emptyObj = {}
let nonEmptyObj = { name: "John", age: 25 }
// function to check if an object is empty or not
// using JSON.stringify() method
function isEmpty(obj) {
return JSON.stringify(obj) === '{}'
}
console.log(isEmpty(emptyObj)) // true
console.log(isEmpty(nonEmptyObj)) // false
3. Using Object.entries() Method
The Object.entries() is another static object method. It returns an array of a given object's own enumerable string-keyed property [key, value] pairs.
For example:
let obj = { name: "John", age: 25 }
console.log(Object.entries(obj)) // [["name", "John"], ["age", 25]]
For an empty object, it returns an empty array.
So to check if an object is empty or not, you can check the length of the array returned by this method. If the length is 0, then the object is empty.
Example
let obj = {}
if (Object.entries(obj).length === 0) {
console.log("Object is empty")
} else {
console.log("Object is not empty")
}
4. Using for...in Loop
The for...in loop is used to iterate over all enumerable properties of an object.
you can use this loop through the object and check for any property using hasOwnProperty() method. If the object has any property, then the object is not empty.
Here is an example:
Example
// check if an object is empty or not
// using for...in loop & hasOwnProperty() method
function isEmpty(obj) {
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
return false
}
}
return true
}
let emptyObj = {}
console.log(isEmpty(emptyObj)) // true
let nonEmptyObj = { name: "John", age: 25 }
console.log(isEmpty(nonEmptyObj)) // false
5. Using Object.getOwnPropertyNames() Method
The Object.getOwnPropertyNames() is an object method very similar to Object.keys(). But it returns an array of all properties (including non-enumerable properties except for those which use Symbol) found directly in a given object.
It returns an empty array for an empty object.
If the length of the returned array is 0, then the object is empty.
Example
function isEmpty(obj) {
return Object.getOwnPropertyNames(obj).length === 0
}
let emptyObj = {}
console.log(isEmpty(emptyObj)) // true
let nonEmptyObj = { name: "John", age: 25 }
console.log(isEmpty(nonEmptyObj)) // false
Conclusion
We have looked at 5 different ways to check if object is empty or not in JavaScript.
You can use any of the above methods that you find easy to use.
But if you want to check if an object is empty or not frequently, then I would recommend you to use the Object.keys() method.
Happy Coding!😇