Count duplicates in array javascript
In this article, we will walk through multiple different ways to count duplicates in an array in javascript.
At the end we will also compare the performance of each method and will find out which one is the fastest.
1. Using foreach loop
To find the count of duplicates in an array, we can use the foreach loop and create a new object to store the count of each element.
Then loop through the array and check if the element is already present in the object, if yes then increment the count by 1, else set the count to 1.
Example
function countDuplicatesReduce(arr) {
const count = {};
arr.forEach(item => {
count[item] = (count[item] || 0) + 1;
});
return count;
}
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
console.log(countDuplicatesReduce(arr));
// Output: { 'a': 3, 'b': 1, 'c': 2, 'd': 1, 'e': 1 }
Problem: You receive count of all the elements in the array, even if the count is 1.
2. Using reduce method
You can also use the reduce() method to find the count of duplicates in an array.
Same as the previous method, create a new object and keep track of the count of each element in the array.
Example
function countDuplicatesReduce(arr) {
return arr.reduce((count, item) => {
count[item] = (count[item] || 0) + 1;
return count;
}, {});
}
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
console.log(countDuplicatesReduce(arr));
Problem: All elements with count 1 are also included in the output.
3. Using map method
We can use the properties of map() method with loop to find the count of duplicates in an array.
First, create an empty map object, then loop through the array and set the count of each element in the map object.
Example
function countDuplicatesMap(arr) {
const count = new Map();
arr.forEach(item => {
count.set(item, count.has(item) ? count.get(item) + 1 : 1);
});
return count;
}
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
console.log([...countDuplicatesMap(arr)]);
4. Using object and simple logic
In this we are using simple logic to find the count of duplicates in an array.
We create an empty object to keep track of the count of each element in the array then for each element we check if it is already present in the object if yes then we increase the count by 1, else we set the count to 1.
At the end we loop through the object and print the elements with count greater than 1.
Example
let my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e'];
// creating an empty object
let duplicates = {};
// looping through each element of the list
for (let num of my_list) {
// checking whether it is in the object or not
if (num in duplicates) {
// increasing count if present
duplicates[num] += 1;
} else {
// initializing count to 1 if not present
duplicates[num] = 1;
}
}
// printing the element with count greater than 1
for (let [key, value] of Object.entries(duplicates)) {
if (value > 1) {
console.log(key, value);
}
}
5. Using Set() with filter method
In this method we create a Set from the array keeping only elements with count greater than 1.
Later you can also count the number of occurrences of each element in the array using the filter() method.
Example
let my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e'];
// removing duplicate elements from the list
let duplicates = new Set(my_list.filter((num) => my_list.filter((n) => n === num).length > 1));
// printing the element with their count
for (let num of duplicates) {
console.log(num, my_list.filter((n) => n === num).length);
}
Conclusion
So, these were 5 different ways to count the number of duplicates in an array in javascript.
Among all methods the fastest method is 3rd method using map() method. Here is performance comparison of all the methods.
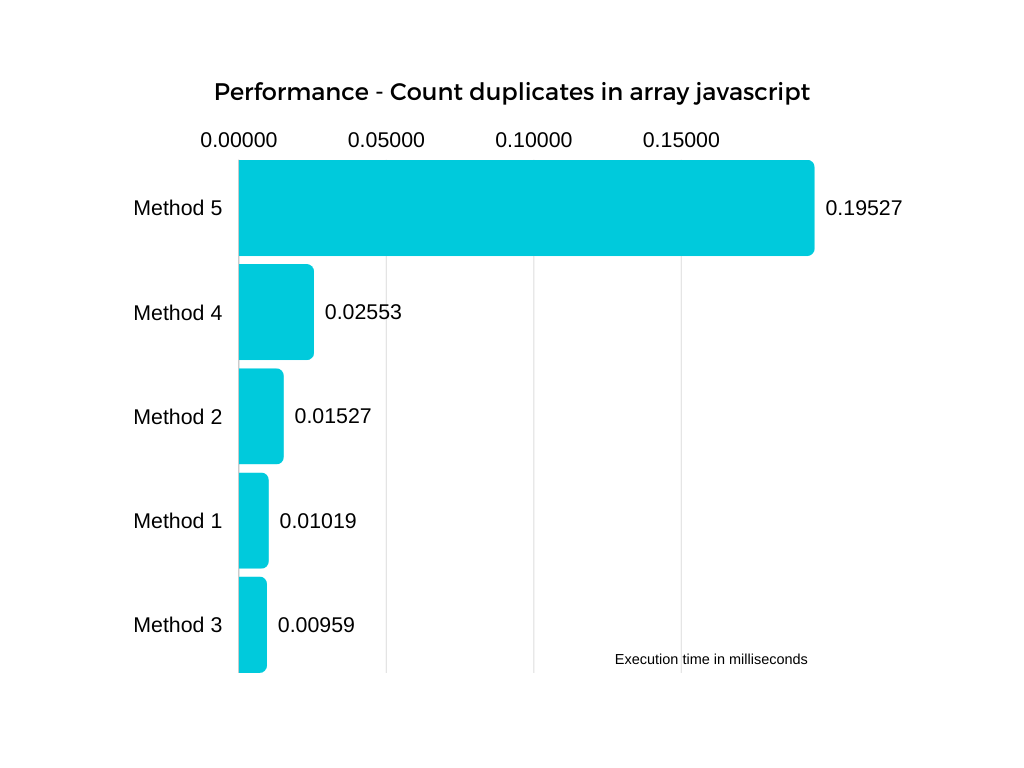