JavaScript Find Duplicates in Array
When working with arrays, you often need to find duplicate elements in the array and perform some operations on them like removing them, counting them, etc.
In this article, you will learn 10 different ways to find duplicate elements in an array in JavaScript.
We will also compare the efficiency of each method by calculating the time taken by each method to find the duplicates.
1. Using Nested for loop
The most basic way to find duplicate elements in an array is by using a nested for loops.
For each element in the array, check if it is present in the rest of the array or not, if it is present, then increment the count of duplicates by 1.
After checking for duplicates for each element, check if the count of duplicates is greater than 1, if yes, then print the element and its count.
Example
// array of elements
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
// nested for loops
for (let i = 0; i < arr.length; i++) {
let count = 1;
for (let j = 0; j < arr.length; j++) {
if (arr[i] == arr[j] && i != j) {
count++;
}
}
// printing duplicate elements
if (count > 1) {
console.log(arr[i] + ": " + count);
}
}
When you run the above code you will get all duplicate elements in the array and their count.
Problem: It prints the duplicate elements multiple times, for example, if the array contains 3 duplicate elements, then it will print the duplicate elements 3 times.
2. Using indexOf() and lastIndexOf()
Another way to find duplicate elements in an array is by using the indexOf() and lastIndexOf() methods.
For each element in the array, check if the first index of the element is equal to the last index of the element, if not, then it is a duplicate element.
Example
// array of elements
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
// looping through each element
for (let i = 0; i < arr.length; i++) {
// checking if first index of element is equal to last index
if (arr.indexOf(arr[i]) !== arr.lastIndexOf(arr[i])) {
console.log(arr[i]);
}
}
When you run the above code you will get all duplicate elements in the array.
Problem: Duplicate elements are printed multiple times.
To fix this problem, you can add duplicate elements to a new array and check if the element is already present in the new array or not, if not, then add it to the new array.
Example
// array of elements
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
// new array to store duplicate elements
let dupArr = [];
// looping through each element
for (let i = 0; i < arr.length; i++) {
// checking if first index of element is equal to last index
if (arr.indexOf(arr[i]) !== arr.lastIndexOf(arr[i])) {
// checking if element is already present in the new array
if(dupArr.indexOf(arr[i]) == -1) {
dupArr.push(arr[i]);
}
}
}
console.log(dupArr);
3. Using filter() and includes()
You can also filter out the duplicate elements from an array using the filter() method.
For each element in the array, check if the element is already present in the new array or not, if not, then add it to the new array.
Example
// array of elements
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
// new array to store duplicate elements
let dupArr = arr.filter((item, index) => arr.includes(item, index + 1));
// print duplicate
console.log(dupArr);
When you run the above code you will get all elements that are duplicates in the array.
4. Using forEach() and includes()
This is the same as using for loop, you need to go through each element in the array and check if the element is already present in the new array or not, if not, then add it to the new array.
Example
// array of elements
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
// new array to store duplicate elements
let dupArr = [];
// looping through each element
arr.forEach((item, index) => {
// checking if element is already present in the new array
if (arr.includes(item, index + 1)) {
// checking if element is already present in the new array
if(dupArr.indexOf(item) == -1) {
dupArr.push(item);
}
}
});
// print duplicate
console.log(dupArr);
5. Using reduce() and includes()
The reduce() method executes a function on each element of the array, that results in a single output value of the array.
You can use this property of the reduce() method to find duplicates by reducing the array by removing the duplicate elements from the array.
Example
// array of elements
let arr = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
// new array to store duplicate elements
let dupArr = arr.reduce((dup, item) => {
if (arr.indexOf(item) !== arr.lastIndexOf(item) && !dup.includes(item)) {
dup.push(item);
}
return dup;
}, []);
// print duplicate
console.log(dupArr);
6. Using map() method
With the use of the property of the map() method, you can find duplicate elements in an array.
Example
function findDuplicatesMap(arr) {
const duplicates = [];
const map = new Map();
arr.forEach(item => {
if (map.has(item)) {
if (!duplicates.includes(item)) {
duplicates.push(item);
}
} else {
map.set(item, true);
}
});
return duplicates;
}
const myArray = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
console.log(findDuplicatesMap(myArray));
Method 7
Example
function findDuplicates(arr) {
const map = new Map();
return arr.filter(item => {
return map.has(item) ? map.get(item) === true : (map.set(item, true), false);
});
}
const myArray = ['a', 'c', 'd', 'a', 'b', 'c', 'a', 'e'];
console.log(findDuplicates(myArray));
Method 8: Using reduce() and some()
Example
const myArray = [1, 2, 3, 2, 4, 3];
let duplicates = [];
myArray.reduce((acc, curr) => {
if (myArray.indexOf(curr) !== myArray.lastIndexOf(curr) && !duplicates.some(item => item === curr)) {
duplicates.push(curr);
}
return acc;
}, []);
console.log(duplicates);
// Output: [2, 3]
Method 9: Using object
Example
const myArray = [1, 2, 3, 2, 4, 3];
let duplicates = [];
let count = {};
myArray.forEach(item => {
count[item] = (count[item] || 0) + 1;
if (count[item] === 2 && !duplicates.includes(item)) {
duplicates.push(item);
}
});
console.log(duplicates);
// Output: [2, 3]
Method 10: Using lodash
Example
const _ = require('lodash');
function findDuplicatesLodash(arr) {
return _.filter(arr, (value, index) => _.includes(arr, value, index + 1));
}
const myArray = [1, 2, 3, 2, 4, 3];
console.log(findDuplicatesLodash(myArray));
// Output: [2, 3]
Efficiency of each method
Now that you have learned 10 different ways to find duplicate elements in an array, we found that there is a rece between a few methods upon testing each method. But Method 7 is the fastest among all.
Here is a speed comparison of each method:
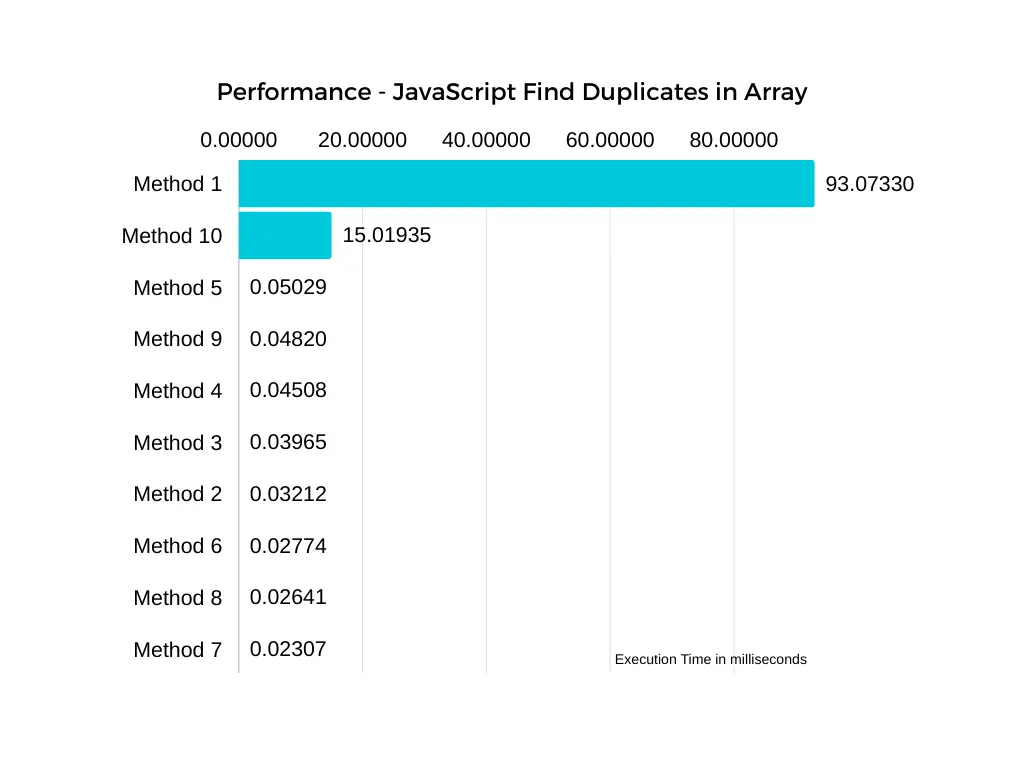