JavaScript lastIndexOf() Method - Check Last Occurrence Of A String
In this tutorial, you will learn about the lastIndexOf() method in JavaScript and will know how to get the last occurrence of any string within a string.

JavaScript lastIndexOf() String Method
The lastIndexOf() is used to find the last occurrence of a substring within another calling string.
It returns the last index value of the string found within the calling string. If no such string is present in the calling string then it returns -1.
Note - For the efficiency of the method, the method starts checking from the end of the string and returns the first occurrence index from backward.
Example
var string = "learning to code is learning to create and innovate";
var search_str = "learning";
// return last occurance of word "learning"
console.log(string.lastIndexOf(search_str)); // 20
console.log(string.lastIndexOf("king")); // -1
You can see in the above example the method returns the index of the last occurrence of the search string ("learning") which is 20 although it first occurs at index 0. Also when searching for the word "king" (which is not in the calling string) it returns -1.
Note: Even though the lastIndexOf() method searches the string from backward, it still returns the location value of the string's first letter from the end of the string.
Syntax of lastIndexOf method
The syntax of javascript lastIndexOf() string method is:
string.lastIndexOf(searchValue, position);
- searchValue - This is the string or character to be searched within the given string.
- position (optional) - It is an optional argument that specifies that the search should be searched before or equal to the given position. If the position is not specified, then the length of the string.
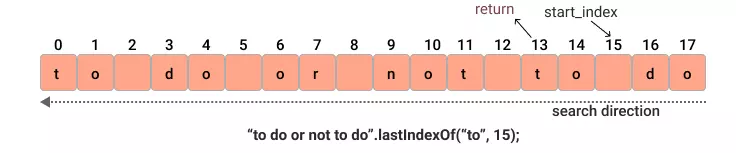
Since the method starts from the backward of the string so if you define the position to be 10, then it will start checking for the value from 10 to 0.
If position >= string.length then the whole string is searched. If position > 0 it would search for nothing.
Example
const string = "to do or not to do";
console.log(string.lastIndexOf("do", 10)); // 3
console.log(string.lastIndexOf("do")); // 16
console.log(string.lastIndexOf("or", 8)); // 6
console.log(string.lastIndexOf("or")); // 6
Note: 'tobetobe'.lastIndexOf('to', 4) will return 4 and not 0, as search_from limits only the beginning of the match.
Case-sensitivity
The lastIndexOf() method is case sensitive.
See the following example:
Example
const str = "To do or not to do";
console.log(str.lastIndexOf("To")); // 0
console.log(str.lastIndexOf("to")); // 13
Using indexOf() and lastIndexOf() Method
The indexOf() method is similar to the lastIndexOf method but it returns the first matching string rather than the last.
Here is an example that searches for the same word using 2 different methods.
Example
const str = "To do or not to do";
console.log(str.indexOf("do")); // 3
console.log(str.lastIndexOf("do")); // 16
Conclusion
The lastIndexOf() method finds and returns the last index of the specified substring from the given string. It is case-sensitive. To get the first index of a specified string using the indexOf() method.