JavaScript String Replace Using replace() Method
In this tutorial, you will learn how to replace a part of a string with another string in JavaScript using replace() method with various examples
Quick Learning
Here is quick learning of the replace() method in JavaScript using example. If you want to learn in detail then proceed further in this article.
Example
// replace() method is used to replace a part
// of a string with another string.
// 1. Replace "Hello" with "Hi" in "Hello World"
var str = "Hello World";
var newStr = str.replace("Hello", "Hi");
console.log(newStr); // Hi World
// 2. Replace numbers with "." (using regex)
var str = "Code12345";
var newStr = str.replace(/\d/g, ".");
console.log(newStr); // Code.....
// 3. call a function for each match
var str = "Hello World";
var newStr = str.replace(/o/g, function(match, index, originalText) {
return "*" + match + "*";
});
console.log(newStr); // Hell*o* W*o*rld
JavaScript replace Method
The replace() method in JavaScript is used to replace a part of a string with another string. It matches a pattern within the string using a string or regular expression and replaces it with a replacement string or a function.
The original string is not modified. The method returns a new string by replacing one or all matches of the calling string.
The search pattern in the method could be a string or a regex.
- string - If the given pattern is a string then the method replaces only the first match in the string
- regex - if the pattern is a regex then it replaces all the occurrences of the matching pattern with the replacement string
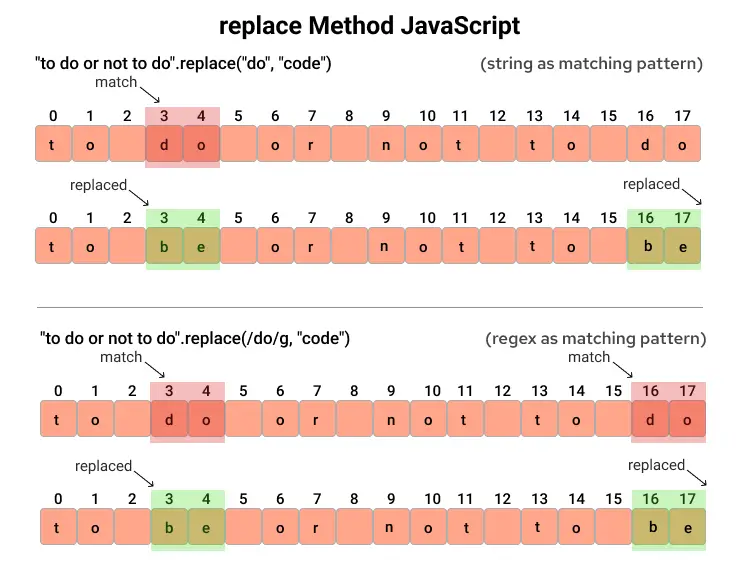
Example
const str = "to do or not to do.";
// string search pattern
const pattern1 = "do";
console.log(str.replace(pattern1, "be"));
// to be or not to do.
// regex search pattern
const pattern2 = /do/g;
console.log(str.replace(pattern2, "be"));
// to be or not to be.
You can see in the above example when the matching pattern is a string then it replaces only the first match ('do'), but when using regex as a matching pattern then it finds all the matches and replaces it.
One thing to note is we have used the /g flag in the regex if you don't use the /g flag then it replaces only the first occurrence just like string.
Syntax of replace method
The syntax of replace() method is as follows:
str.replace(substr, new_string);
str.replace(regexp, new_string);
str.replace(substr, function);
str.replace(regexp, function);
-
substr - It is the string that is to be replaced by the new_string. It is treated as a literal string and is not treated as a regular expression, so only the first occurrence of this is replaced.
-
regexp - It is the regular expression, the pattern used to replace the match or matches with new_string.
-
new_string - It is the replacement string that replaces the matches by the pattern.
-
function - It is a function that is invoked for every match of the pattern in the string. The return value of the replacement function is used to replace the matching string. This function is discussed in detail below.
Note: The original string is untouched by the replace() method, a new string is returned after replacement.
JavaScript replace String Examples
Now as we have discussed the replace() method and gone through its syntax let's now see various examples using string and regex.
# Example 1: Using string to match
The following example uses a substring to match content and replace it with a new string. When regex is used then it finds all the matchings within the string and replaces it with a new string using replace() method.
Example
const str = "to do or not to do.";
const pattern = "do"; // string
console.log(str.replace(pattern, "Sleep"));
// to Sleep or not to do.
# Example 2: Using regexp to match
The following example uses a regular expression to match content and replace it with a new string. As it is a string so only the first match is replaced by the new string when using replace() method.
Example
const str = "to do or not to do.";
const pattern = /do/g; // regex
console.log(str.replace(pattern, "Sleep"));
// to Sleep or not to Sleep.
Note: Use /g flag with regex, if not used then it will only replace the first match.
Example
const str = "to do or not to do.";
const pattern = /do/; // no g flag
console.log(str.replace(pattern, "Sleep"));
// to Sleep or not to Sleep.
# Example 3: Channing replace method
Suppose you want to replace 2 more than 2 different substrings within a string with another new string. So the basic idea to do this would be to replace one by one different matches and assign a return value every time to the original string, as shown in the example below.
Example
let str = "To do or not to do.";
str = str.replace(/do/gi, 'code'); // replace all do with code
str = str.replace(/to/gi, 'love'); // replace all to with love
console.log(str);
// love code or not love code.
But instead of doing this you can chain the replace() method and do this in a single line of code.
Example
let str = "To do or not to do.";
str = str.replace(/do/gi, "code").replace(/to/gi, "love");
// replace all do with codeand replace all to with love
console.log(str);
// love code or not love code.
Using a replacement function
The replace method can also accept a replacement function instead of a new string as the second parameter.
let newString = str.replace(substr | regex, replacement_function);
When a replacement function is used then it will invoke after the match has been performed.
For multiple matches when used regular expression with /g flag it will be invoked for each match from the string.
The return value of the replacement function is used as a new string to replace matchings.
Example
const str = "to do or not to do.";
const pattern = /do/g;
function replacer() {
return "Sleep";
}
console.log(str.replace(pattern, replacer));
// to Sleep or not to Sleep.
In the above example, you can see the return value of the replacement function is used as a new string to replace the matches.
Syntax of replacement function
function replacer(match, p1, p2, ..., offset, string) {}
The arguments of the replacement function are as follows:
Parameter | Description |
---|---|
match | Matching part of the string by the pattern |
p1, p2, ... | This is the string found by parenthesized capturing group in series. This means p1 will be the first capture of capturing group, p2 will be the second, and so on. |
offset | It is the index value of matched substring within the string. |
string | The string method is called upon |
The following example shows for what match the string is replaced by using the replacement function.
Using match in replacement function
const str = "to do or not to do.";
const pattern = /do/g;
function replacer(match) {
return `(${match}) => Sleep`;
}
console.log(str.replace(pattern, replacer));
// to Sleep or not to Sleep.
The following example shows the captures of the capturing group by the regex.
Example
const str = "to do or not to do.";
const pattern = /d(o)/g;
function replacer(match, p1) {
return `(${match}) => Sleep \n(capturing ${p1})\n`;
}
console.log(str.replace(pattern, replacer));
This example shows the captures offset of the capturing group within the string.
Example
const str = "to do or not to do.";
const pattern = /d(o)/g;
function replacer(match, p1, offset) {
return `(${match}) => Sleep \n(capturing ${p1}) at index ${offset}\n`;
}
console.log(str.replace(pattern, replacer));
This example uses all the parameters of the replacement function.
Example
const str = "to do or not to do.";
const pattern = /d(o)/g;
function replacer(match, p1, offset, string) {
return `(${match}) => Sleep \n(capturing ${p1}) at index ${offset} in (${string})\n`;
}
console.log(str.replace(pattern, replacer));
Array Function As Replacement Function
The array function can also be used as a replacement function for the replace() method. You can either directly put it in the method as an anonymous function or use a function with a name.
Example
const str = "to do or not to do.";
const pattern = /d(o)/g;
const newString = str.replace(pattern, (match, p1, offset, string) => {
return `(${match}) => Sleep \n(capturing ${p1}) at index ${offset} in (${string})\n`
})
console.log(newString);
Switching Words In String
Use $n to catch the words to switch in the string. The $n represents the nth parenthesized capturing word, where n is a positive integer less than 100.
Example
const str = 'Do Be do be';
const regexp = /(\w+)\s(\w+)\s(\w+)\s(\w+)/;
const newstr = str.replace(regexp, "$2 $1, $4 $3");
console.log(newstr); // Be Do, be do
Alternate method: Using Split and Join Method In Array
Instead of using replace() method, you can use a combination of split and join method to create the same functionality.
Split the string from the matching string you want and join them using the join method and use the new string as a separator. See the example below.
Example
const str = "to do or not to do.";
const subStr = "do";
const replaceBy = "Sleep";
const newStr = str.split(subStr).join(replaceBy);
console.log(newStr);
The above example split the string using the split method at "do" and join them using "Sleep" as a separator in the join method.
Conclusion
In this tutorial, you learned everything about the replace() method and it's uses. The method can be used to replace a string with another string or call a function for each match in the string.