JavaScript String Methods
In this tutorial, we will learn about all the frequently use JavaScript string methods. We will see its use with examples.
Introduction To JavaScript String Methods
Javascript String methods are special build-in methods (functions) that play out a particular assignment when applied on any valid string.
These methods return a new string with little change from the original string. The original string is not changed.
String in javascript is a primitive data type, so it does not have any methods or properties but javascript treats these primitive values as objects when we execute it with some method or properties.
Here is a simple example of a string method, find the position of "World" in the string "Hello World".
Test String = "Hello World"
Using indexOf()
method
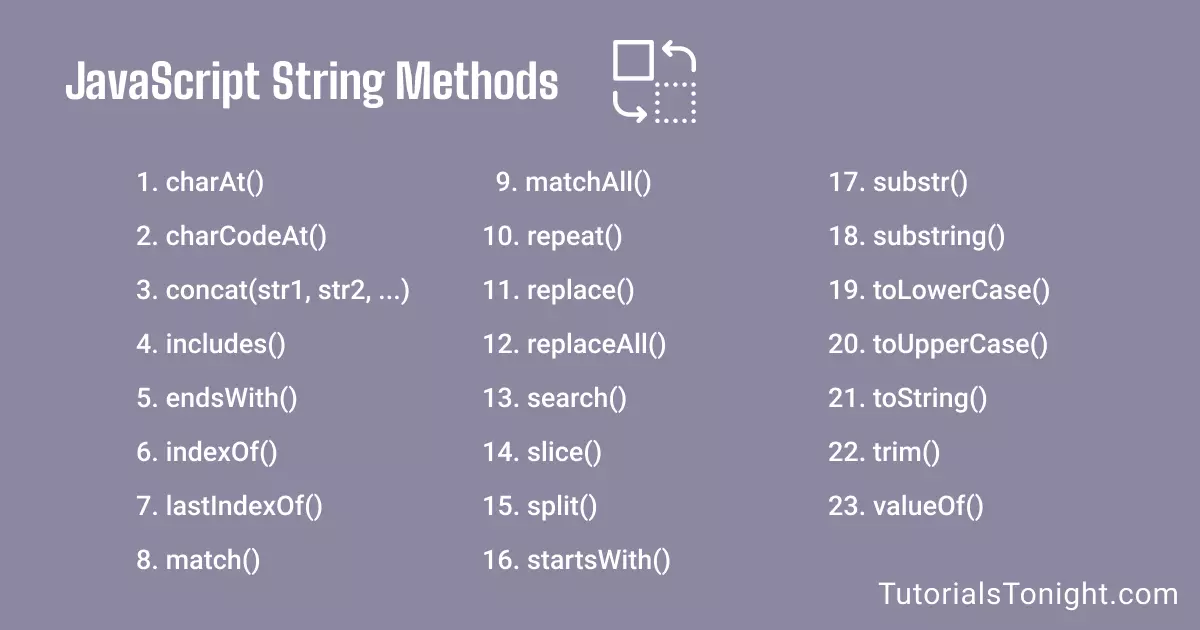
Javascript String Length
Before discussing string methods let's look at 1 string property that javascript has which is the length property.
The length is a string property that is used to find the length of a string. It returns the number of characters in the string including spaces.
To use it apply it to the string as shown below.
var str = "Hello World";
str.length; // 11
The length is only a read-only data property of a string.
The length of an empty string is 0.
Example
const str = "Hello World";
// using length property
const len = str.length; // output: 11
console.log("length of string = " + len);
const empty = "";
console.log(empty.length);
Try It
Javascript String Methods List
JavaScript has a large number of string methods all for different purposes. We have listed some of the most frequently use string methods for you.
Here is the list of string methods with examples. Click on any method to jump to the section.
- charAt()
- charCodeAt()
- concat()
- endsWith()
- includes()
- indexOf()
- lastIndexOf()
- match()
- matchAll()
- repeat()
- replace()
- replaceAll()
- search()
- slice()
- split()
- startsWith()
- substr()
- substring()
- toLowerCase()
- toUpperCase()
- toString()
- trim()
- valueOf()
let's now look at each String methods in detail.
1. charAt in javascript
The charAt() string method in javascript returns a character at the specific index of the given string.
The charAt()
method takes the index value as an argument and returns the corresponding character from the calling string.
An index value of a string starts with 0, which means the first character has an index value of 0, the second character has an index value of 1, and so on.
Example
const str = "Hello World";
// using charAt() method
console.log(str.charAt(1));
console.log(str.charAt(6));
Try It
The default index value in the charAt method is 0.
If the index value is out of range then it returns an empty string ('').
If the index can not be converted to an integer then this method returns the first character of the string.
Example
const str = "Hello World";
//default index value = 0, return first character
console.log(str.charAt());
// index out of range, return empty string
console.log(str.charAt(13));
// index can't be converted to an integer, return first character
console.log(str.charAt('a'));
# Altenative way to charAt() method
You can consider the string as an array of characters and call out any character of the string by its index value, as all the characters are a member of the array.
Example
const str = "Hello World";
// using square bracket notation
console.log(str[0]);
console.log(str[1]);
console.log(str[13]); // index out of range, return empty string
2. charCodeAt in javascript
The charCodeAt string method in javascript returns the Unicode value (between 0 and 65535) of the character present at the given index of the string. Example Unicode value of 'A' is 65.
The charCodeAt()
method takes an index as an argument and returns the Unicode value of the character present at that index value.
Example
const str = "Hello World";
// using charCodeAt() method
console.log(str.charCodeAt(0));
console.log(str.charCodeAt(1));
Try It
The default value of the index is 0. If the index value is not given or it can't be converted to a string the 0 is used by default.
If the index value is out of range then this method returns NaN
.
Example
const str = "Hello World";
//default index value = 0
console.log(str.charCodeAt());
console.log(str.charCodeAt("x"));
// Out of range returns NaN
console.log(str.charCodeAt(13));
3. concat in javascript
The concat string method in javascript concatenates the passed string in the method to the calling string and returns the concatenated string as a new string.
The concat()
method can take any number of strings as an argument.
If the passed argument is not a string then it converts the argument to string and then concatenates it.
Example
const firstName = "Hello";
const lastname = "World";
// using concat method
let result1 = firstName.concat(" ", lastname);
console.log(result1);
// multiple arguments
let result2 = "Learning".concat(" ", "to", " ", "code.");
console.log(result2);
Try It
Note: for concatenation of string it is suggested to use assignment operators (+
or +=
) instead of the concat
method.
Example
const firstName = "Hello";
const lastname = "World"
console.log(firstName + " " + lastname);
console.log("Learning" + " " + "to" + " " + "code.");
4. endsWith in javascript
The endsWith string method in javascript is used to determines whether the string ends with a specified substring or not.
If it ends with a specified string then it returns true
, else returns false
.
The substring to be checked for the ending is passed as the first argument in the method.
Example
const question = "What is DOM?";
// using endsWith method
// checks whether the string ends with "?"
console.log(question.endsWith("?"));
Try It
There is also a second argument in the method which is optional. It specifies a length value for the string. If you pass the length value is 5 then it will see only the first 5 characters of the string.
Please note that endsWith()
method is case sensitive.
Example
const question = "What is DOM?";
// check only first 5 characters
console.log(question.endsWith("?", 5));
5. String includes in javascript
The includes string method in javascript is used determines whether a given substring is present in the calling string or not.
If the string is present then the method returns true
, if not substring is not present then the method returns false
.
The matching of includes()
method for the string is case-sensitive.
Example
const sentence = "Carbon emission is increasing Day by day.";
// check if the string contains words
console.log(sentence.includes("day")); // true
console.log(sentence.includes("Day")); // true
console.log(sentence.includes("DAY")); // false
Try It
The includes also accept an optional second argument which determines the position from where the method starts searching within the string
The default value of the second argument is 0.
Example
const sentence = "Carbon emission is increasing day by day.";
// checking from the index 20
console.log(sentence.includes("is", 20));
// checking from the index 2
console.log(sentence.includes("is", 2));
// checking from the index 5
console.log(sentence.includes("emission", 5));
6. indexOf in JavaScript
The indexOf string method in javascript is used to get the index of the first occurrence of a specified value in a string.
If the character or substring is not present then it returns -1. The search string is case-sensitive.
Example
const sentence = "Carbon emission is increasing day by day";
// get 1st index of 'day'
console.log(sentence.indexOf("day"));
console.log(sentence.indexOf("Carbon"));
// case-sensitive, returns -1
console.log(sentence.indexOf("carbon"));
Try It
If no search string is provided to the method then default search string is 'undefined'. Example 'undefined'.indexOf()
will return 0.
There is a second argument that is optional that defines the start index from where the method starts to search for the character or substring.
Example
const sentence = "Carbon emission is increasing day by day";
// no substring provided to search
console.log(sentence.indexOf());
console.log('undefined'.indexOf());
// start searching from index 40
console.log(sentence.indexOf("day", 40));
7. String lastIndexOf JavaScript
The lastIndexOf()
string method in javascript searches the last occurrence of a substring within a string. The method starts searching from the end of the string for efficiency.
If the substring is not in the given string then it returns -1.
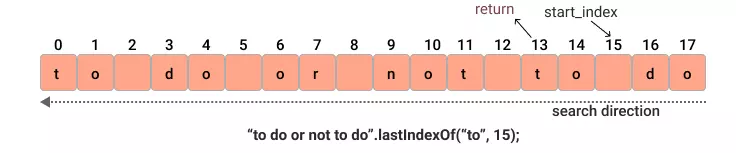
Example
const sentence = "to do or not to do";
// finding index of last "to"
console.log(sentence.lastIndexOf("to"));
Try It
If nothing is passed in the method then the default search string is 'undefined'.
Example 'undefined'.lastIndexOf()
will return 0, because there is no argument and now the lastIndexOf method will search for undefined
which is at index 0.
Example
const sentence = "to do or not to do";
// nothing to search, so will search for 'undefined'
console.log(sentence.lastIndexOf());
console.log('undefined'.lastIndexOf());
There is an optional second argument that is fromIndex. It defines the index from where the method starts to search for the substring from the end of the string.
Its default value is +Infinity. If value of fromIndex is greater than the length of the string then whole string is searched.
Example
const sentence = "to do or not to do";
// searching from index 10
console.log(sentence.lastIndexOf("do", 10));
console.log(sentence.lastIndexOf("do", -2));
Try It
8. match in javascript
The match() string method in javascript uses a regular expression to match a series of characters within the calling string.
The method returns the output as an array of string with matched character or strings as its element.
If the parameter passed is not a regular expression then it is implicitly converted to RegExp by using new RegExp(regexp)
.
Example
const series = "bdWg2AdjgH4du5jUgT";
// match all capital letters and numbers
console.log(series.match(/[A-Z0-9]/g));
Try It
If the used regular expression does not have a g flag then the method returns only the first complete match and its capturing group.
If nothing matches within the string then the method returns null
.
Example
const string = "to do or not to do";
// no g flag
console.log(string.match(/do/));
// no match found, return null
console.log(string.match(/dog/));
9. matchAll in javascript
The matchAll() string method in javascript is an improved variant of the match() method.
In the match method when the regular expression is used without the g flag then it works fine but with the g flag, it only returns the matches and not its capturing group.
But the matchAll() method finds all the matching string with its capturing group against the regular expression.
Instead of returning an array the matchAll() method returns an iterator to all results matching the string. You will have to explicitly convert it to array using sperad operator ([...]
) or using Array.from() method.
Note: It is compulsory to use g flag with the matchAll() method.
Example
const series = "to do or not to do";
// matching "do" and capturing group
const array = [...series.matchAll(/d(o)/g)];
console.log(array);
console.log(array[0])
Try It
10. repeat in javascript
The repeat() method concatenates a passed string by a specified number of times and return it as a new string.
A number of times string is to be repeated is passed as an argument in the method, where the number lies between 0 and +Infinity.
If a decimal value is passed then it will be converted to an Integer when passed.
The method reports an error for a negative number.

Example
const str = "Tick tock, ";
// repeat the string by 2 times
console.log(str.repeat(2));
// converts the decimal value to integer
console.log(str.repeat(3.5));
// repeat 0 times
console.log(str.repeat(0));
Try It
11. replace in javascript
The replace method selects one or all matches from a string and replace it with a replacement string and return it as new string.
To find the match method use string or regular expression. When a string is passed as an argument then it select only the first match while when a regular expression is passed then it selects all the matches.
Example
const str = "Carbon emission is increasing day by day.";
// using string to match
console.log(str.replace("day", "year"));
// using regular expression to match
console.log(str.replace(/day/g, "year"));
Try It
Further, the replacement can be a string to be replaced in thr string or a function to be called for each match.
Here is an example that use function in the replace method to be called for each match.
Example
const str = "Carbon emission is increasing day by day.";
// using string to match
str.replace("day", foundMatch);
// using regular expression to match
str.replace(/day/g, foundMatch);
function foundMatch(match, index, string) {
console.log(`match = ${match}, index = ${index}`);
}
12. replaceAll in javascript
The replaceAll method returns a new string after replacing all occurrences of matched pattern with a replacement string.
Unlike replace() method it replaces all the occurrences whether the given pattern is a string or a regular expression.
The replacement can be a string to be replaced or a function to be called for each match.
Note: While using regular expression in the replaceAll() method using a global flag (g) is compulsory with it otherwise it will throw a 'TypeError'.
Example
const str = "Carbon emission is increasing day by day.";
// select all match using both string or regular expression
console.log(str.replaceAll("day", "year"));
console.log(str.replaceAll(/day/g, "month"));
Try It
13. search in javascript
The search string method in javascript is used to determine whether a pattern exists within the calling string or not, if it exists then the method returns the index value of the first match within the string.
The search method uses regex to search for a pattern in a string, if a string is passed to search for then the method implicitly convert it to regex.
14. slice in javascript
The slice string method in javascript extracts a part of the string and returns it as a new string.

str.slice( startIndex, [, endIndex])
The slice()
takes 2 arguments, the first argument is the start index for the slicing string and the second argument is the end of the slicing string, where the second argument is optional.
The default value of endIndex is str.length When the second argument is not passed then the string is sliced from 'startIndex' to the end of the string.
The slice()
method also accepts negative value, where -1 represents the last index
Example
const sentence = "Carbon emission is increasing day by day";
console.log(sentence.slice(5, 15));
console.log(sentence.slice(5));
console.log(sentence.slice(-15, -5));
Try It
15. split in javascript
The split string method in javascript divides the given string intro substring and returns an array of substrings.
The method takes an argument which is a pattern to be used for dividing the string.
str.split(pattern, [, limit])
- If the pattern is an empty string ('') then the method split the string at each character
- If the pattern is a string (' ') then the method split the string at each space
- If the pattern can be a regular expression. '\n' splits the string at each new line
The limit defines the maximum number of substrings to be returned. If it is 0 then an empty array ([]) is returned.
Example
const sentence = "Carbon emission is increasing day by day";
// no pattern -> return whole string in array
console.log(sentence.split());
// split at each space
console.log(sentence.split(''));
// split at each space
console.log(sentence.split(' '));
// split at each 'is'
console.log(sentence.split('is'));
Try It
16. startsWith in javascript
The startsWith string method in javascript determines whether a string starts with some given substring or not. If it starts with the desired string then it returns true
else return false
.
The search string is passed as the first argument to the method. There is also an optional argument that defines the position from where the method should start checking.
The startsWith method is case-sensitive.
Example
const sentence = "Carbon emission is increasing day by day";
console.log(sentence.startsWith("Car"));
console.log(sentence.startsWith("carbon")); // return false case-sensitive
console.log(sentence.startsWith("bon", 3));
Try It
17. substr in javascript
The substr() string method in javascript is used to extract a substring from a string. It returns a part of the string, starting at a specific index and ending after a given number of characters.
str.substr(startIndex, [, length])
- startIndex - It specifies the index value from where the substring starts
- length - It defines number of characters to be extracted
If the value of startIndex is negative then the index is counted from the end of the string in opposite direction. If it is NaN
then it is treated as 0.
Example
const sentence = "Carbon emission is increasing day by day";
// start at index 10, cut 6 characters
console.log(sentence.substr(10, 6));
// start at index 10, cut all characters
console.log(sentence.substr(10));
// negative index
console.log(sentence.substr(-4, 3));
Try It
18. substring in javascript
The substring() method extracts a part of string between 2 given index values. It returns a part of the string, starting at a specific index and ending after a given number of characters.
str.substring(startIndex, endIndex)
- startIndex - It specifies the index value from where the substring starts
- endIndex - It specifies the index value from where the substring ends
If endIndex is not specified then it is treated as the last character of the string.
If the value of startIndex is greater than the value of endIndex then value of these two variables is swapped.
Example
const sentence = "Carbon emission is increasing day by day";
// extracting string
// start at index 10, cut all characters
console.log(sentence.substring(10));
// start at index 10, and end at index 20
console.log(sentence.substring(10, 20));
// startIndex > endIndex
console.log(sentence.substring(20, 10));
Try It
19. toLowerCase in javascript
The toLowerCase()
string method in javascript converts the case of a string to lowercase and returns it as a new string.
Example
const sentence = "CARBON emission IS INCREASING DAY BY DAY";
console.log(sentence.toLowerCase());
Try It
20. toUpperCase in javascript
The toUpperCase string method in javascript returns a new string by converting the calling string to uppercase.
Example
const sentence = "carbon emission is increasing day by day";
console.log(sentence.toUpperCase());
Try It
21. toString in javascript
The toString()
string method in javascript returns a string representing the specified object.
This method also convert numbers to strings in a different number system. For example you can convert a number to a string in binary system by using toString(2)
, octal system by using toString(8)
and hexadecimal system by using toString(16)
, etc.
Example
const str = new String("hello World!");
console.log(str.toString(str));
const num = 20;
console.log(num.toString(2));
Try It
22. trim in javascript
The trim()
string method in javascript removes whitespaces from both ends of the string. Whitespaces are space, tabs, newline, etc.
23. valueOf in javascript
The valueOf()
string method in javascript returns the primitive value of a String object.
Conclusion
You have seen 23 different string methods in javascript with their use and examples. This string method makes your job easy while dealing with string in javascript.