Operators In JavaScript
In this tutorial, you will learn about different types of operators in JavaScript with examples and explanations.
What are Operators in Javascript?
Operators are symbols that defines different kind of oprtations like mathematical operation, logical operation, etc. For example, the symbol of addition (+)
tells the javascript engine to add given operators.
These operators with operands (variables or values) are used to perform mathematical operations on the operands. For example, 2 + 3
is a valid javascript expression.
There are many different types of operators in javascript.
- Arithmetic Operators
- Comparison Operators
- Assignment Operators
- Logical Operators
- Bitwise Operators
- Special Operators
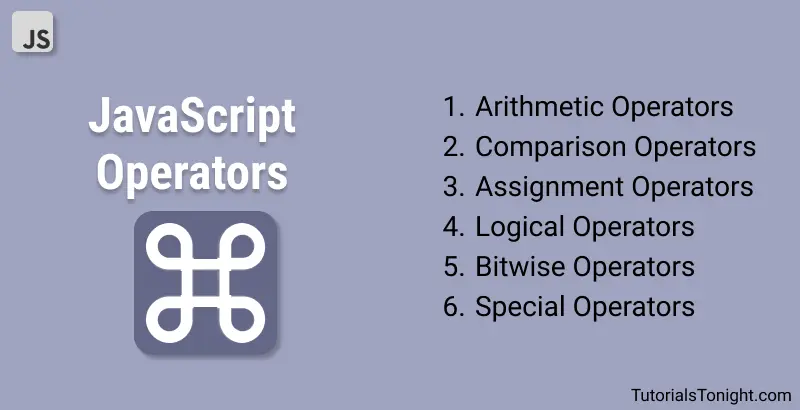
1. Arithmetic Operators
Arithmetic operators are used to perform arithmetic operations on javascript variables or values. For example, 2 + 3
, 2 - 3
, 2 * 3
, etc.
Following is the list of arithmetic operators in javascript.
We will be using operands x = 5 and y = 2 in the examples below.
Operator | Description | Example |
---|---|---|
+ | Addition | x + y (result = 7) |
- | Subtraction | x - y (result = 3) |
* | Multiplication | x * y (result = 10) |
/ | Division | x / y (result = 2.5) |
% | Modulus (remainder) | x % y (result = 1) y % x (result = 2) 5 % 4 (result = 1) |
++ | Increment (add 1 to the value) | x++ (result = 6) value become 7 but use in current operation will be 6 ++y (result = 3) value become 3 and use in current operation is 3 |
-- | Decrement (subtract 1 from the value) | x-- (result = 5) value become 4 but use in current operation will be 5 --y (result = 1) value become 1 and use in current operation is 1 |
Now let's see some examples of arithmetic operators in javascript.
Example
let x = 5, y = 2;
addition = x + y;
subtraction = x - y;
multiplication = x * y;
division = x / y;
console.log(addition); // 7
console.log(subtraction); // 3
console.log(multiplication); // 10
console.log(division); // 2.5
// modulus (remainder)
modulus1 = x % y; // 1
modulus2 = 7 % 5; // 2
modulus3 = 5 % 5; // 0
console.log(modulus1, modulus2, modulus3);
// increment (add 1 to the value)
x++; // 6
++y; // 3
console.log(x, y);
// decrement (subtract 1 from the value)
// x is 6 and y is 3 now
x--; // 5
--y; // 1
console.log(x, y);
Try It
2. Comparison Operators
Comparison operators are used to compare two values. They return true
or false
values on the basis of the result.
For example, 2 == 2
, 2 != 2
, 2 < 3
, etc.
Following is the list of comparison operators in javascript.
Operator | Sign | Description | Example |
---|---|---|---|
Equal to | == | Check if two values are equal | 2 == 2; // true |
Identical to | === | Check if two values are identical | 2 === 2; // true 2 === '2'; // false |
Not Equal to | != | Check if two values are not equal | 2 != 2; // false |
Less than | < | Check if the first value is less than the second value | 2 < 3; // true |
Greater than | > | Check if the first value is greater than the second value | 2 > 3; // false |
Less than or Equal to | <= | Check if the first value is less than or equal to the second value | 2 <= 3; // true |
Greater than or Equal to | >= | Check if the first value is greater than or equal to the second value | 2 >= 3; // false |
Now let's see some examples of comparison operators.
Example
// equal to
console.log(2 == 2); // true
console.log(2 == '2'); // true
// identical to
console.log(2 === 2); // true
console.log(2 === '2'); // false
// not equal to
console.log(2 != 2); // false
console.log(2 != '2'); // false
// less than
console.log(2 < 3); // true
// greater than
console.log(2 > 3); // false
// less than or equal to
console.log(2 <= 3); // true
// greater than or equal to
console.log(2 >= 3); // false
Try It
3. Assignment Operators
The assignment operator is used to assign or change the value of the operator. For example, a = 10
now a is equal to 10.
In assignment operators can also do arithmetic operations and then assign results to operators. For example, let a = 10; a += 10
now a become 20 as a += 10
is equivalent to a = a + 10
.
Following is the list of assignment operators in javascript. In the following table when a
is not defined then consider a = 10
.
Operator | Sign | Description | Example | Equivalent to |
---|---|---|---|---|
Assignment | = | Assign value to the operator | a = 10; // a is equal to 10 | a = 10; |
Addition | += | Add and assign value to the operator | a += 10; // a is equal to 20 | a = a + 10; |
Subtraction | -= | Subtract and assign value to the operator | a -= 10; // a is equal to 10 | a = a - 10; |
Multiplication | *= | Multiply and assign value to the operator | a *= 10; // a is equal to 100 | a = a * 10; |
Division | /= | Divide and assign value to the operator | a /= 10; // a is equal to 10 | a = a / 10; |
Modulus | %= | Modulus and assign value to the operator | a %= 10; // a is equal to 0 | a = a % 10; |
Exponent | **= | Exponent and assign value to the operator | a **= 2; // a is equal to 100 | a = a ** 2; |
Bitwise AND | &= | Bitwise AND and assign value to the operator | a &= 2; // a is equal to 2 | a = a & 2; |
Bitwise OR | |= | Bitwise OR and assign value to the operator | a |= 2; // a is equal to 12 | a = a | 2; |
Bitwise XOR | ^= | Bitwise XOR and assign value to the operator | a ^= 2; // a is equal to 6 | a = a ^ 2; |
Left Shift | <<= | Left Shift and assign value to the operator | a <<= 2; // a is equal to 8 | a = a << 2; |
Right Shift | >= | Right Shift and assign value to the operator | a >>= 2; // a is equal to 2 | a = a >> 2; |
Now let's see some examples of assignment operators.
Example
let x = 100;
console.log(x); // 100
// addition
let a = 10;
a += 10;
console.log(a); // 20
// subtraction
let b = 5;
b -= 3;
console.log(b); // 2
// multiplication
let c = 6;
c *= 2;
console.log(c); // 12
// division
let d = 15;
d /= 3;
console.log(d); // 5
// modulus
let e = 7;
e %= 3;
console.log(e); // 1
// exponent
let f = 2;
f **= 3;
console.log(f); // 8
// bitwise AND
let g = 5;
g &= 2;
console.log(g); // 0
// bitwise OR
let h = 5;
h |= 2;
console.log(h); // 7
// bitwise XOR
let i = 5;
i ^= 2;
console.log(i); // 7
// left shift
let j = 5;
j <<= 2;
console.log(j); // 20
// right shift
let k = 5;
k >>= 2;
console.log(k); // 1
Try It
4. Logical Operators
Logical operators perform logical operations in javascript. For example, && operation true && true = true
.
Logical operators are also used to combine conditional statements. They are used to combine conditional statements to determine the result of the statement.
The following table lists the logical operators and their results.
Operator | Sign | Description | Example |
---|---|---|---|
Logical AND | && | Returns true if both operands are true. If the first statement is false then it returns false and does not execute the second statement. | true && true = true false && true = false |
Logical OR | || | Returns true if at least one operand is true. | true || true = true false || true = true false || false = false |
Logical NOT | ! | Returns true if the operand is false, otherwise returns false. | !true = false !false = true |
Nullish coalescing operator | ?? | Returns first argument if it is not null or undefined | a ?? b (returns a if not null/undefined) |
Now let's see some examples of logical operators.
Example
// Logical AND
console.log(true && true); // true
console.log(true && false); // false
console.log(false && true); // false
// Logical OR
console.log(true || true); // true
console.log(true || false); // true
console.log(false || true); // true
// Logical NOT
console.log(!true); // false
console.log(!false); // true
// Nullish coalescing operator
let a = null;
let b = 5;
console.log(a ?? b); // return second argument, because a is null
console.log(b ?? a); // return first argument, because it is neither null nor undefined
Try It
5. Bitwise Operators
Bitwise operators are the operators that are used to change the bit values of the operands. Bitwise operators treat their operands as a sequence of 0's and 1's of 32 bits.
These are the following bitwise operators in javascript.
Operator | Name | Description |
---|---|---|
& | AND | give 1 only when both bits are 1. ie 1 & 1 = 1 |
| | OR | give 0 only when both bits are 0. ie 0 | 1 = 1 and 0 | 0 = 0 |
^ | XOR | give 1 only when one bits is 1. ie 1 & 1 = 0 and 0 ^ 1 = 1 |
~ | NOT | Reverse the bit value. ie ~ 1 = 0 |
<< | Left Shift(zero fill) | Shift all bit values to left by the given number and fill 0 in rightmost. ie 5 << 1=10 |
>> | Right Shift (sign preserving) | Shift all bit values to right by the given number and preserving sign. ie -10 >> 1 = -5 |
>>> | Right Shift (zero fill) | Shift all bit values to right by the given number and fill 0 in rightmost. ie 15 >>> 1=7 |
Lets see an example.
Example
let a = 5 & 2; // output 0
let b = 5 | 2; // 101 | 010 = 111 (output: 7)
let c = 5 ^ 2; // 101 ^ 010 = 111 (output: 7)
let d = 5 << 1; // 101 -> 1010 (output: 10)
let e = 5 >> 1; // 101 -> 010 (output: 2)
let f = 8 >>> 1; // 1000 -> 0100 (output: 4)
console.log(a, b, c, d, e, f);
Try It
6. Special Operators
Special operators serve special purposes. The following operators lie under special operators categories.
These are the following special operators in javascript.
Operators | Description | Example |
---|---|---|
Instanceof | Checks whether the object is an instance of the given constructor. |
|
typeof | Returns the type of the given object. |
|
delete | Deletes the given property from the object. |
|
in | Checks whether the given property is present in the object. |
|
new | Creates a new object. |
|
this | Gives the current object. |
|
? : | Conditional operator. |
|
Let's see these operators in brief with an example.
Instanceof
The instanceof
operator checks whether the object is an instance of the given constructor.
Example
let a = {};
console.log(a instanceof Object); // true
console.log(a instanceof Array); // false
let b = 25;
console.log(b instanceof Number); // true
console.log(b instanceof String); // false
typeof
The typeof
operator returns the type of the given object.
Example
let a = {};
console.log(typeof a); // object
console.log(typeof 1); // number
console.log(typeof "string"); // string
delete
The delete
operator deletes the given property from the object.
Example
let car = {
"name": "Ferrari",
"year": "2016",
"color": "red"
};
delete car.year;
console.log(car);
// { "name": "Ferrari", "color": "red" }
in
The in
operator checks whether the given property is present in the object.
Example
let car = {
"name": "Ferrari",
"year": "2016",
"color": "red"
};
console.log('year' in car); // true
console.log('color' in car); // true
console.log('engine' in car); // false
new
The new
operator creates a new object.
Example
let a = new Object(); // a is an object
console.log(typeof a);
this
The this
operator gives the current object.
Example
let a = {
"name": "John",
outputName: function() {
return this.name;
}
};
console.log(a.outputName()); // John
Ternary Operator ? :
The ? :
operator (Ternary Operator) is a conditional operator. It evaluates the first expression and returns the first value. If the first expression is false, it evaluates the second expression and returns the second value.
Example
let a = 1;
console.log(a ? "Yes" : "No"); // Yes