Alphabet Pattern in javascript
Alphabet pattern
An alphabet pattern is a series of alphabets (uppercase or lowercase) that create a certain pattern or geometrical shape such as square, pyramid, triangle, etc. These patterns are created using nested controlled loops.
These pattern programs are used to practice and boost programming skills mainly loops and are also asked in programming interviews.
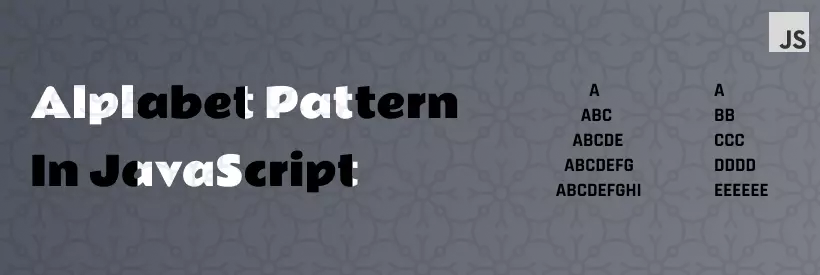
Javascript loop through alphabet
Before we start, let's see a simple example of how to loop through the alphabet in javascript.
We will use the ASCII value of a letter to loop through the alphabet.
ASCII value is a number that represents the character. For example, the letter A is represented by 65, Z is 90, and so on.
We will also use String.fromCharCode() to convert the number to a letter.
The following example is to loop through the alphabet and print the letter on the screen.
Example
for(let i = 65; i <= 90; i++){
console.log(String.fromCharCode(i));
}
Print Alphabet Pattern In Javascript
Here we have discussed 12 different alphabet patterns in detail with their programs in javascript.
Alphabet Pattern 1:
A A B A B C A B C D A B C D E
To create above pattern run 2 nested for loop
. Run external loop for 'N' number of times, where 'N' is number of rows in the square, i.e for(let i = 0;i < N;i++)
.
The internal loop will run for 1 time in the first iteration of external code, 2 times in the second iteration, and so on.
In each the iteration of internal loop add 65 to variable "j" and convert it to a character using String.fromCharCode()
(65 is ASCII value of 'A').
Example
let n = 5; // you can take input from prompt or change the value
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
// printing characters
for (let j = 0; j < i; j++) {
string += String.fromCharCode(j + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 2:
A B B C C C D D D D E E E E E
Pattern2 is similar to pattern1, only difference is in characters. You can see pattern2, characters are changing only in the next iteration of the external loop. So you can simply use 'i - 1' while creating characters instead of 'j' ('i - 1' because 'i' starts from 1).
Example
let n = 5;
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
// printing characters
for (let j = 0; j < i; j++) {
string += String.fromCharCode((i - 1) + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 3:
A B C D E F G H I J K L M N O
This pattern is the same as pattern1 only difference is that the character is changing in each and every iteration. To achieve this you can simply create a random variable ('count' in the below example) and increase it in every iteration and use it to get the character.
Example
let n = 5; // you can take input using prompt or change the value
let string = "";
let count = 0;
// External loop
for (let i = 1; i <= n; i++) {
for (let j = 0; j < i; j++) {
string += String.fromCharCode(count + 65);
count++; // increment cause next alphabet
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 4:
ABCDE ABCD ABC AB A
In this pattern just control the internal loop such as it runs for 'N' times in the first iteration of the external loop, 'N - 1' times in the second iteration, and so on. To get this set initialization variable (j) less than 'n - i + 1'
. Now use the initialization variable of the internal loop for character increment.
Example
let n = 5; // you can take input using prompt or change the value
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
for (let j = 0; j < n - i + 1; j++) {
string += String.fromCharCode(j + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 5:
EDCBA EDCB EDC ED E
This pattern is the same as pattern4 only difference is that instead of starting the character being 'A' it is the character at ASCII value 'N - 1 + 65', where 'N' is the height of the pattern.
To achieve this use 'N - 1 - j'
for character creation and add 65 to it (65 is ASCII value of A).
Example
let n = 5; // you can take input using prompt or change the value
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
for (let j = 0; j < n - i + 1; j++) {
string += String.fromCharCode((n - 1 - j) + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 6:
EDCBA DCBA CBA BA A
This pattern is the same as pattern4 only difference is that in pattern4 starting character is 'A' but in this pattern ending character is 'A' in each row.
To achieve this use 'N - i - j'
for character creation and add 65 to it (65 is ASCII value of A).
Example
let n = 5; // you can take input using prompt or change the value
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
for (let j = 0; j < n - i + 1; j++) {
string += String.fromCharCode((n - i - j) + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 7: Pyramid patten
A ABC ABCDE ABCDEFG ABCDEFGHI
This is a pyramid-shaped pattern using the alphabet, we have created the pyramid pattern using stars in the last section. Using the same technique create the pattern and instead of printing stars, print alphabets using String.fromCharCode
.
Example
let n = 5;
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
// creating spaces
for (let j = 0; j < n - i; j++) {
string += " ";
}
// creating alphabets
for (let k = 0; k < 2 * i - 1; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 8: Pyramid pattern
A BCD EFGHI JKLMNOP QRSTUVWXY
This pattern is the same as pattern7 just alphabets are increasing in each and every iteration.
To keep track of this create a variable and increment it in every iteration of the internal loop and use this variable to create alphabets.
Example
let n = 5;
let string = "";
let count = 0;
// External loop
for (let i = 1; i <= n; i++) {
// creating spaces
for (let j = 0; j < n - i; j++) {
string += " ";
}
// creating alphabets
for (let k = 0; k < 2 * i - 1; k++) {
string += String.fromCharCode(count + 65);
count++;
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 9: Reverse Pyramid Pattern
ABCDEFGHI ABCDEFG ABCDE ABC A
This is a reverse pyramid pattern using alphabets. Just control the formation of spaces and the creation of stars in reverse order. See the code below to understand.
Compare codes of pyramid and reverse pyramid for better understanding.
Example
let n = 5;
let string = "";
// External loop
for (let i = 1; i <= n; i++) {
// creating spaces
for (let j = 1; j < i; j++) {
string += " ";
}
// creating alphabets
for (let k = 0; k < 2 * (n - i + 1) - 1; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 10: Diamond Pattern
A ABC ABCDE ABCDEFG ABCDEFGHI ABCDEFG ABCDE ABC A
The diamond pattern is a combination of the pyramid and reverse pyramid alphabet patterns.
Example
let n = 5;
let string = "";
// Pyramid
for (let i = 1; i <= n; i++) {
for (let j = 1; j < n - i + 1; j++) {
string += " ";
}
for (let k = 0; k < 2 * i - 1; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
// Reverse Pyramid
for (let i = 1; i <= n - 1; i++) {
for (let j = 1; j < i + 1; j++) {
string += " ";
}
for (let k = 0; k < 2 * (n - i) - 1; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 11: Hourglass Pattern
ABCDEFGHI ABCDEFG ABCDE ABC A ABC ABCDE ABCDEFG ABCDEFGHI
Hourglass pattern is a combination of the reverse pyramid and pyramid alphabet patterns.
Example
let n = 5;
let string = "";
// Reverse Pyramid
for (let i = 1; i <= n; i++) {
for (let j = 1; j < i; j++) {
string += " ";
}
for (let k = 0; k < 2 * (n - i + 1) - 1; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
// Pyramid
for (let i = 1; i <= n - 1; i++) {
for (let j = 1; j < n - i; j++) {
string += " ";
}
for (let k = 0; k < 2 * (i + 1) - 1; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
console.log(string);
Try It
Alphabet Pattern 12: Pascal Pattern
A AB ABC ABCD ABCDE ABCD ABC AB A
Pascal pattern is the same as a diamond pattern just remove spaces and change conditions in internal loops.
Example
let n = 5;
let string = "";
// Pyramid
for (let i = 1; i <= n; i++) {
for (let k = 0; k < i; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
// Reverse Pyramid
for (let i = 1; i <= n - 1; i++) {
for (let k = 0; k < n - i; k++) {
string += String.fromCharCode(k + 65);
}
string += "\n";
}
console.log(string);
Try It
Conclusion
In this article, we learn and create 12 different alphabet patterns. You can think of alphabet pattern programs as 1 step above star pattern programs because they need a little more logic than start pattern programs.