JavaScript If Else: Condition Within The Code
In this tutorial, you will learn about conditional statements in JavaScript. You will know about if-else statements and how to use them in JavaScript.
Introduction
While writing code there are many situations when you need to make a decision and decide what to do if a certain thing happens.
For example, if today is Sunday then print "don't go to the class", if feeling hungry then "eat something", etc.
While programming you also need to take such a decision. To make such decisions you need to use if-else statements also knows conditionals.

Example
let hungry = true;
if (hungry) {
console.log('eat something then code');
} else {
console.log('Sit and code');
}
- Conditional Statements
- if Statements
- else Statements
- else if Statements
- Switch statement
- Nested if statements
Table Of Contents
Conditional Statements
A conditional statement is a statement that is used to make a decision. When the condition is given is true then the code is executed.
JavaScript has the following conditional statements:
- if statement - if statement is used to execute a block of code only when a given condition is true.
- else statement - else statement is used to execute a block of code only when a given condition is false.
- else if statement - else-if statement provides a new condition to be checked if the previous condition was false to execute a new block of code.
- switch statement - switch statement is a shorter and cleaner way to use when there are many conditions to be checked and it works the same as an if-else statement.
1. JavaScript if statement
if statement is the most basic conditional statement. It executes a block of code only when the given condition is true
.
if the condition is false then execution leaves the block and jumps to the next section.
Here is a flow diagram that shows how the if statements execute their block of code.
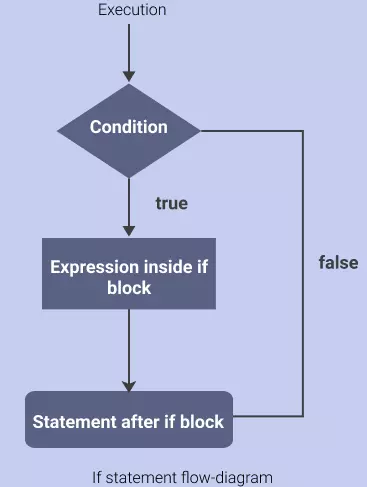
You can see in the first code execution checks the condition if the condition is true
then it enters in the if block and executes the code if the condition is false
then statement after if block is executed.
if statement syntax
if (condition) {
//code
}
Here is an example that checks conditions and outputs the result if the condition is true
.
In the above example condition, x == 10
is true so it executes the statement inside the if block.
Possible mistake: While checking condition don't use assignment operator i.e. if (x = y)
is correct while if (x == y)
is correct.
Example #1 - Using boolean in condition
It is not necessary to give some statement as a condition, you can directly specify true or false in the place of condition. If it is true then code will always execute.
Example
if (true) {
console.log("Condition is true. Block executed!");
}
Example #2 - multiple conditions
The if statement accepts multiple conditions, which means you can specify more than 1 condition separated by logical operators.
The following example checks if the number is between 10 and 20 using multiple conditions.
Example
let a = 15;
if (a > 10 && a < 20) {
console.log("Yes 'a' is between 10 and 20.");
}
Example #3 - multiple conditions 2
The following example checks if the number is between 10 and 20 or is divisible by 5 using multiple conditions.
Example
let a = 15;
let b = 5;
if (a % 5 == 0 || a > 10 && a < 20) {
console.log("Yes 'a' is between 10 and 20 or is divisible by 5.");
}
if (b % 5 == 0 || b > 10 && b < 20) {
console.log("Yes 'b' is not between 10 and 20 but is divisible by 5.");
}
Example #4 - Skipping curly braces
If there is only 1 line of code in the block of the if statement then you may emit the curly braces and can write the code within the same line.
Example
if (true) console.log("Condition is true");
2. else statement JavaScript
The else statement is executes a code block when the condition in the if statement is false
.
else statement can't be used alone it must be used after the if statement
.
Here is a flow diagram for else statements working.

You can see from the above image that if the condition becomes false then code execution jumps to a different block which is an else block.
else statement syntax
if (condition) {
// executes this block if the condition is true
} else {
// executes this block if the condition is false
}
Example #1
In the example below condition is false so it executes the else block.
Example
var a = 15;
if (a == 10) {
console.log("True, a = 10");
}
else {
console.log("False, a is not equal to 10");
}
Try it
Example #2
Example
var a = 15;
if (a == 10 || a > 20) {
console.log("True, a = 10 or a >20");
}
else {
console.log("a = 15");
}
3. else if statement JavaScript
The else if statement is used to execute a block of code when the condition in if statement is false
.
The else if statement is similar to the else statement but it is used to check multiple conditions.
It is always used after any if
statement.
The if-else statement is used to execute a block of code among 2 alternatives while else if statement gives other alternatives to it.
The following image explains how the else if statement is executed.
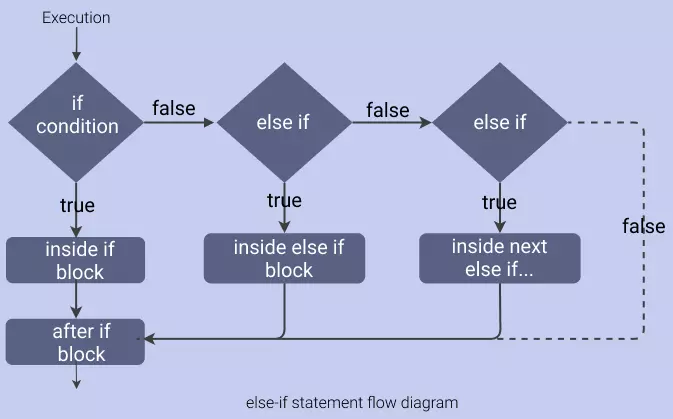
You can see in the above image when the condition is false in the if statement then execution jumps to the next else if statement, if again its condition is false then execution jumps to the next else if statement, and so on. And finally, if all statements are false then it executes else
statement and exit the statement.
else if syntax
if (condition) {
// executes this block if the condition is true
}
else if (condition) {
// executes this block if the condition is false
}
... (more else if blocks)
else {
// executes this block if the condition is false
}
The syntax above may look complicated in the first view but is just a series of if, else if, and else statement.
The following examples show how else if statement is used.
Example #1
Example
var a = 15;
if (a == 10) {
console.log("True, a = 10");
}
else if (a == 15) {
console.log("True, a = 15");
}
else {
console.log("False, a is not equal to 10 or 15");
}
Try it
Example #1
An example to check what day is today.
Example
let today = new Date().getDay();
if (today == 0) {
console.log("Sunday!");
}
else if (today == 1) {
console.log("Monday!");
}
else if (today == 2) {
console.log("Tuesday!");
}
else if (today == 3) {
console.log("Wednesday!");
}
else if (today == 4) {
console.log("Thursday!");
}
else if (today == 5) {
console.log("Friday!");
}
else {
console.log("Saturday!");
}
Try it
4. switch statement
The switch statement is used to execute a block of code based on a value.
The switch statement is similar to the if statement but it is used to check multiple conditions.
switch
statement is a collection of many conditions in an ordered manner that work exactly like if statement.
We will study switch statements in detail in the coming section.
Let's see how you will write the above day of the week example using the switch statement.
Example
let today = new Date().getDay();
switch (today) {
case 0:
console.log("Sunday!");
break;
case 1:
console.log("Monday!");
break;
case 2:
console.log("Tuesday!");
break;
case 3:
console.log("Wednesday!");
break;
case 4:
console.log("Thursday!");
break;
case 5:
console.log("Friday!");
break;
case 6:
console.log("Saturday!");
break;
}
Nested if statements
The conditional statements can be nested to each other. This means you can write an if statement within another if statement.
Here is an example that clearly explains how you can use nested if-else.
Example
let employee = {
name: "herry",
age: 32,
experience: 6
}
if (employee.age > 30) {
if (employee.experience > 5) {
console.log("Eligible!");
}
else {
console.log("Not eligible!");
}
}
else {
if (employee.experience > 3) {
console.log("Eligible!");
}
else {
console.log("Not eligible!");
}
}
Conclusion
In this section, you learned how to make decisions in javascript in different cases. You looked at four different types of conditional statements in JavaScript.
Frequently Asked Questions
-
What is if else statement in JavaScript?
The if-else statement is used to execute a block of code based on a condition. If the condition is true, the code inside the if statement is executed. If the condition is false, the code inside the else statement is executed.
-
What is switch statement in JavaScript?
The switch statement is used to execute a block of code based on the match of a value.
-
Which is an alternative for if else statement?
You can use either switch statement, ternary operator, or logical operators.