JavaScript repeat String Method: Repeat String N Times
In this tutorial, you will get to know how to repeat a string multiple times and get a single string using the repeat() string method.
You would have come across many situations when you see a simple string repeating itself over and over. Example lines in a song, dance step count (123 123...), etc.
So how can you programmatically create such a string?
Simple, By using repeat() method for string.
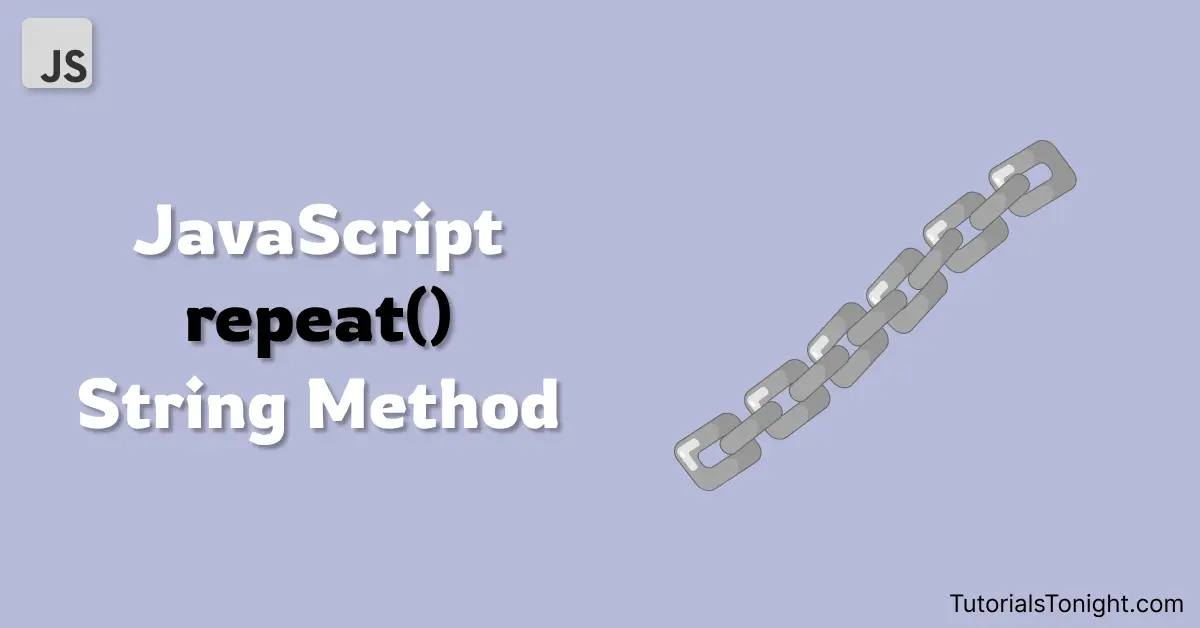
JavaScript repeat String
The repeat() method in javascript is used to create a new string by repeating and concatenating the existing string by the given number of times.
The method returns a new string containing copies of string on which method is called.
Example
const str = "tick tock, ";
// javascript repeat string by 5 times
console.log(str.repeat(5));
Syntax of repeat string method
The syntax of repeat() string method is:
string.repeat(count)
- string - This is the string you want to repeat.
- count - It is the number of times you want the string to repeat, between 0 and +Infinity. The default value of count is 0.
Exception
-
If the repeat count is a negative number then it reports a RangeError.
Example - negative number
const str = "tick tock, "; console.log(str.repeat(-1)); // RangeError
-
If the repeat count is not less than Infinity or string size overflow maximum string size then also it reports a RangeError.
Example - Infinity
const str = "tick tock, "; console.log(str.repeat(Infinity)); // RangeError
Using repeat()
Here is some random use of the repeat() method.
If the count is 0 then the method returns an empty string.
If count is a fractional value then the method converts it to an integer.
Example
console.log('abc'.repeat(0)); // ''
console.log('abc'.repeat(1)); // 'abc'
console.log('abc'.repeat(3)); // 'abcabcabc'
console.log('abc'.repeat(5.5)); // 'abcabcabcabcabc' (count converted to integer)
/*
console.log('abc'.repeat(-1)); // RangeError
console.log('abc'.repeat(1 / 0)); // RangeError
*/
Using Loop To Repeat String
You can use a while loop to create your own function similar to the repeat() method.
Example
function repeatString(string, count) {
let finalString = "";
while (count) {
finalString += string;
count--;
}
return finalString;
}
console.log(repeatString("tick tock, ", 5));
In the above example, the Javascript function accepts 2 arguments first is the string that will be repeated and the second is counter number, that counts the number of time the string is to be repeated.
A variable named 'finalString' is created that stores the repeated string and is returned finally by the function.
The function runs a 'while loop' that is executed for the number of times the string is to be repeated, every time loop is executed string is added to the final string and the counter is decreased by 1.
Another Hack To Repeat String
There is another way to repeat the string and concatenate it. Use Array.join method and pass the string to repeat as separator in the join method.
Since the separator is placed between 2 array elements so you have to call it for N + 1 times.
Example
const str = "tick tock, ";
const times = 5;
console.log(Array(times + 1).join(str)); // repeat string for 5 times
Also you can use fill with this.
Example
const str = "tick tock, ";
console.log(Array(5).fill(str).join('')); // repeat string for 5 times
Conclusion
In this article, we have seen how to use the repeat() method to repeat a string for a given number of times.
We have also seen how to use a while loop to repeat a string for a given number of times.
We have also seen how to use Array.join method to concatenate an array of strings.