Substring In JavaScript And How To Get It
In this tutorial, You are going to learn about substring in JavaScript and how to extract a substring from a string using different methods.
We will first understand substring, and its relation with strings and then will look at different ways to get the substring from a string.
- What is substring in JavaScript?
- How to get substring in javascript
- substring() Method
- slice() Method
- substr() Method(deprecated ๐)
- Difference between substring and slice
- Difference between substring and substr
- Getting all substrings from a string
- Javascript Substring After Character
- check if string contains substring
Table Of Contents
What Is Substring In JavaScript?
A substring is a sequence of characters within a string. For example, "Hello World" is a string, and "Hello" is a substring of "Hello World".
A substring is always a part of a string. It can be of any length from 1 character to the entire string.
Note: It is to be noted that only a sequence of characters is considered to be a substring not a subsequence of characters.
For the string 'code' list of all substrings would be :
'code', 'cod', 'ode', 'co', 'od', 'de', 'c', 'o', 'd', 'e'
The image below is a visual representation of the above list of substrings.
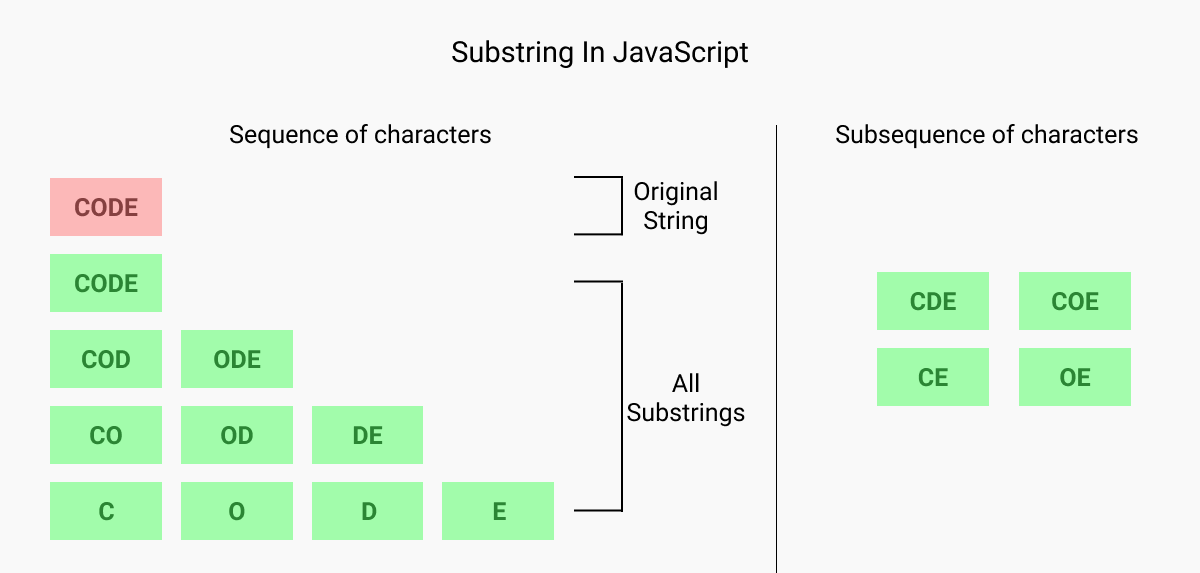
Get Substring In JavaScript
There are basically 3 methods to get substring in javascript:
- substring() Method
- slice() Method
- substr() Method (deprecated ๐)
Here we are going to all these methods in detail.
1. JavaScript substring Method
The substring() is a built-in javascript method which is used to extract a substring from a string.
It takes 2 indexes as arguments and returns the substring between those indexes.
Example
const str = "Learning";
// substring between index 0 and index 5
console.log(str.substring(0, 5));
// substring between index 2 and index 5
console.log(str.substring(2, 5));
# Syntax substring()
The syntax of the substring() method is:
str.substring(startIndex, endIndex);
Point to be noted:๐๐ก
- startIndex - This specifies the index of string from where the substring starts to be extracted.
- If startIndex is
undefined
,NaN
, or is not specified then it is treated as 0.
- If startIndex is
- endIndex (optional) - This specifies the ending index (not included) of the substring to be extracted.
- If endIndex is not given substring() extracts characters to the end of the string
- If the value of startIndex is less than the value of endIndex then the value of both arguments is swapped.
- If both arguments are equal then the method returns an empty string
Now let us see some examples to get substring using the substring() method.
# Example 1
Example
const str = "Learning to code";
// substring between index 2 and index 5
console.log(str.substring(2, 5));
// substring between index 0 and index 4
console.log(str.substring(0, 4));
// using substring() method without endIndex
console.log(str.substring(2));
console.log(str.substring(5));
# Example 2 - Without endIndex
Example
const str = "Learning to code";
// using substring() method without endIndex
console.log(str.substring(2));
console.log(str.substring(5));
# Example 3 - When startIndex > endIndex they swap values
Example
const str = "Learning to code";
// both logs out 'earn'
// when startIndex is greater than endIndex
// the value of both arguments are swapped
console.log(str.substring(1, 5));
console.log(str.substring(5, 1));
# Example 4 - Make copy of string
Example
const str = "Learning to code";
// creating copy of string
console.log(str.substring(0));
console.log(str.substring());
2. JavaScript slice Method
The slice() is another built-in method that can be used to extract a substring from a string.
slice method accepts 2 parameters startIndex and the endIndex same as the substring() method and returns the part of the original string between 2 desired index values as a new string.
The original string is not modified.
Example
let str = "Learning to code";
// slice between index 0 and index 5
console.log(str.slice(0, 5));
// slice between index 2 and index 5
console.log(str.slice(2, 5));
The methods substring() and slice() are very similar. But there are a few differences between them which you will learn later in this chapter.
3. Javascript substr Method
Note: substr() method is deprecated and will be removed in future versions of JavaScript. Use slice() or substring() instead.
The substr() method is used to extract a substring from a string. It returns a part of the string starting from a given index of a given length.
Example
const str = "Learning to code";
// starting from index 1 and length of 4
console.log(str.substr(1, 4));
In the above example, the substr method extracts a substring from the original string from index 1 to index 4.
# Syntax substr()
The syntax of substr() method is:
string.substr(startIndex, length)
Point to be noted about this method:๐๐ก
- startIndex - It is the first parameter of the method which determines the index in the original string from where the substring is to be extracted.
- If startIndex is
NaN
orundefined
then it is treated as 0. - If startIndex is a negative number index is counted from the end of the string.
- If startIndex is
- length (optional) - This is an optional parameter that determines the length of the character to be included in the substring.
- If length is omitted or is
undefined
then substr() extracts substring to the end of the string. - If the length given is negative or
NaN
(Not a Number) then it is treated as 0.
- If length is omitted or is
let's now look at some examples to see substr() method in action.
# Example 1
The second parameter length specifies the length of the substring to be extracted using substr() method.
Example
const str = "Learning to code";
// start index is 1, length is 7
console.log(str.substr(1, 7));
// start index is 3, length is 2
console.log(str.substr(3, 2));
# Example 2 - Length is not given
Example
const str = "Learning to code";
// length not given
// string extract to end of the string
console.log(str.substr(5));
# Example 3 - Different length value
Let's see what is the behavior of substr() method at different length values.
Example
const str = "Learning to code";
// length 0
console.log("length 0 => " + str.substr(2, 0)); // ''
// length undefined
console.log("length 'undefined' => " + str.substr(5, undefined));
// length NaN
console.log("length 'NaN' => " + str.substr(10, NaN)); // ''
// length Infinity
console.log("length 'Infinity' => " + str.substr(1, Infinity));
// negative length
console.log("negative index => " + str.substr(-4, 3)); // ''
Javascript substring vs slice
We have learned both substring and slice methods in the above and you may be confused about the similarity and differences between them.
Look at the image below for knowing similarities and differences between substring() and slice() methods.

Let's see their difference with examples.
// syntax
string.slice(start, stop);
string.substring(start, stop);
But there are some differences between them:
-
The substring() method swaps the value of arguments if startIndex is greater than endIndex but the slice() method returns an empty string in such case.
const str = "Learning to code"; // argument swapped console.log(str.substring(5, 1)); // return empty string console.log(str.slice(5, 1));
-
If any of the arguments in the substring() method is negative then the method treats them as 0, but the slice() method counts from backward for negative values.
const str = "Learning to code"; // negative start index value console.log(str.substring(-5, 3)); // -5 is treated as 0 console.log(str.slice(5, -3)); // negative end index value // -3 becomes 0, then value swapped console.log(str.substring(5, -3)); console.log(str.slice(5, -3));
Javascript substring vs substr
Both substring and substr methods are used to extract a substring from a string but there are some differences between them.
substring() | substr() |
---|---|
substring() method extracts substring between given 2 indexes. | substr() method extracts substring starting from a given index and upto a given length. |
substring() method is currently in use. | substr() method deprecated. |
substring() method is slower than substr() method. | substr() method is way faster than substring() method. |
Preformance comparision between substring() and substr() method:
Performance comparison
var str = "";
for (var i = 0; i < 1000000; i++) {
str += "a";
}
var start = new Date().getTime();
for (var i = 0; i < 1000000; i++) {
str.substring(0, 1);
}
var end = new Date().getTime();
console.log("substring: " + (end - start));
var start = new Date().getTime();
for (var i = 0; i < 1000000; i++) {
str.substr(0, 1);
}
var end = new Date().getTime();
console.log("substr: " + (end - start));
Get all substrings of a string
Let's use the above methods to get all possible substrings from a string and count them.
Using substring() Method
First, we will use the substring() method which has 2 parameters startIndex and endIndex.
By controlling the startIndex and endIndex in the substring() method we can print all possible substrings. Fix startIndex and iterate endIndex from the current value to the last index of the string, the increase startIndex by 1 and do the same.
Look at the code below to understand.
Example
const sentence = "Code";
// print all possible substrings
let count = 0;
for (let startIndex = 0; startIndex <= sentence.length; startIndex++) {
for (let endIndex = startIndex + 1; endIndex <= sentence.length; endIndex++) {
console.log(sentence.substring(startIndex, endIndex));
count++;
}
}
console.log("Number of substrings = " + count);
# Using substr() Method
To get all substrings we will loop through the given string and use its index value and check all possible lengths of a substring starting from that index. For example, fixing at index 0 we will get all substring starting from 0 of any length, then increase index to 1 and get all substring again and so on.
Example
const sentence = "Code";
// print all possible substrings
let count = 0;
for (let index = 0; index < sentence.length; index++) {
for (let len = 1; len <= sentence.length - index; len++) {
console.log(sentence.substr(index, len));
count++;
}
}
console.log("Number of substrings = " + count);
Javascript Substring After Character
How will you find the substring after a given character? ๐ญ๐ค
Simply find the index of the given character and then use the substring() method to get the substring starting from that index.
Here is how you can do it.
Example
const str = "Learning-Javascript is fun";
const char = "-";
// get substring after the character
console.log(str.substring(str.indexOf(char) + char.length));
Check If String Contains Substring Javascript
To check if a string contains a substring, we can use 2 different methods:
- includes() method
- indexOf() method
The includes() method returns true if the string contains the substring, otherwise it returns false.
Example
const str = "Learning Javascript is fun";
// Using includes method
console.log(str.includes("Javascript"));
console.log(str.includes("funny"));
The indexOf() method returns the index of the first occurrence of the substring, or -1 if the substring is not found.
Example
const str = "Learning Javascript is fun";
// Using indexOf method
console.log(str.indexOf("Javascript") !== -1);
console.log(str.indexOf("funny" !== -1));
Frequently Asked Questions
What is a substring JavaScript?
A substring is a part of a string that is selected from a string. For example, if you have a string "Learning Javascript is fun" then "Javascript" is a substring of "Learning Javascript is fun".
How do substring () and substr () differ in JavaScript?
The substring() method uses 2 indexes to get the substring, while substr() method uses 1 index (starting position) and length to get the substring.
How do you slice a substring in JavaScript?
You can use the slice() method to get a substring from a string. The syntax is stringName.slice(startIndex, endIndex).
How do I extract a string from a word in JavaScript?
You can use any of the three mentioned methods: substring(), slice(), and substr().
Conclusions
JavaScript substring is a sequence of characters from a given string without skipping any character. You can use substring(), slice() and substr() method to get substrings from a string.
We also have discussed multiple different scenarios that are worth mentioning.