JAVASCRIPT VARIABLES
In this tutorial, you will learn about variables in javascript, their type, their scope, how to create them, and how to use them.
Variables in JavaScript
In programming, we need to store data and values to be used later in the programs (ex. a program to double a number, so we must have that number stored). So we need something where we can store and use the data
In javascript, variables are containers that can be used to store data like strings, numbers, objects, etc.
Variables can be defined, can be assigned value, value can be altered, and can use stored value later by referring to the name of the variable.
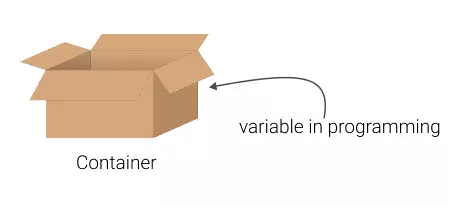
How to create a variable?
In javascript there are three different ways to create a variable:
- By using the
var
keyword - By using the
let
keyword - By using the
const
keyword
We will discuss variable creation only using the var
keyword here. We will discuss the other two in the next section.
To create a variable use the var
keyword followed by the name of the variable
Example:
// variable is created using the var keyword
var name;
var age;

Now we have 2 different containers (variables) with the name 'name' and 'age'.
Assigning value to a variable
To assign value to a variable use the =
sign.
You can assign both single or multiple variables to the same line. Example:

Example
var name = "John";
var age = 25;
console.log(name, age);
// multiple variable assignment in same line
var fruit = "Apple", price = 50;
console.log(fruit, price);
Note: Variable names are case-sensitive. so the variable names "Bob&"" and "bob" are different.
Variable Naming Convention
Variable names can be simple like x, y or can be more clear like bookName. Every variable name should be unique.
When you have a more meaningful name of a variable then it becomes easy to read and maintain the code.
There are some rules that need to be followed while giving a name to the variable.
- Variable name should start with a letter or underscore
_
- Variable name should not contain any special characters like
$
,#
,@
,%
,^
, etc. - Variable name should not start with a number
- Variable names can contain numbers, digits (A-Z, a-z) and underscores
- Only two special characters are allowed in variable name:
$
and_
- Reserved words can't be used as variable names like
let
,class
,true
, etc.
Here is an example to show the above rules:
var 1abc; // invalid
var _1abc; // valid
var _1_abc; // valid
var _1_abc_; // valid
var $ = 5; // valid
var _$ = 5; // valid
var _1_abc$ = 5; // valid
var _ = "hello"; // valid
var cat123$ = "Some cats"; // valid
var cat123_ = "Some cats"; // valid
var dog@12 = "Some Dog"; // invalid
var abc = 10; // valid
var Abc = 11; // invalid
// abc and Abc are different
How to use a variable?
To use a variable in a program, we need to use the name of the variable.
While using variable name shouldn't be wrapped in quotes ('
or "
) otherwise it will become a string.
Example:
Example
var name = "John";
console.log(name);
// this output name as string
console.log("name");
How to change a variable?
You can also change a value stored in a variable, just like replacing one object with another in a container.
To change a variable value use the =
sign followed by the new value.
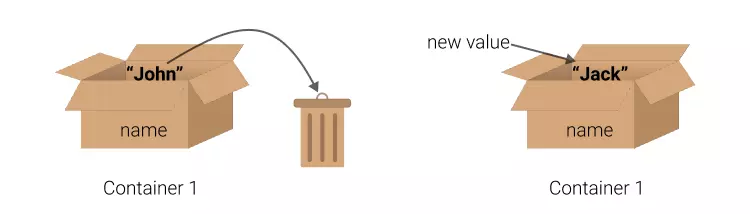
Example
var name = "John";
name = "Jack";
console.log(name);
Scope of a variable
The scope of a variable is the area of the program where it can be used. It can be used in the entire program or only in a specific part of the program.
The scope of a variable is determined by the location where it is declared. It can be declared in the global scope, in a function, in a loop, etc.
Scopes are of 2 types:
- Global Scope - The variable is accessible in the entire program
- Local Scope - The variable is accessible only in the function where it is declared
Example:
Example
var user = "John"; // global scope
function sayHello() {
console.log(user);
}
sayHello();
In the above example, the user is declared in the global scope. So, it is accessible in the entire program.
In the below example, the user is declared in the function sayHello. So, it is accessible only in the function sayHello.
Example
function sayHello() {
var user = "John"; // local scope
console.log(user);
}
sayHello();
// undefined 👇
// console.log(user);
Let and Const in Javascript
let
and const
are the new keyword in javascript that came with ES6. These two keywords are also used to define variables in javascript.
scope of let
and const
are at block { } level. const
is similar to let
but it can't be reassigned once assigned a value. const
is generally used to declare constant variables.
Example
let a = 5;
let x = "hello";
const PI = Math.PI;
const b = 100;
console.log(x, PI, b);
// will show error because it is const can't reassign value
// b = 15;
Conclusion
In this tutorial, we have learned how to use variables in javascript. We have also learned about the scope of variables and how to change a variable value.
We looked at let
and const
keywords briefly.
Frequently asked questions
-
what are variables used for in javascript programs?
Variables are used to store values in a program to be used later on.
-
How many types of variables are there in JavaScript?
There are 5 types of variables in JavaScript: numbers, strings, booleans, arrays, and objects.
-
What is a global variable JavaScript?
Global variables are variables that are accessible in the entire program.
-
What is a local variable JavaScript?
Local variables are variables that are accessible only in the function where they are declared.
-
What is scope of a variable in JavaScript?
The scope of a variable is the area of the program where it can be used. It can be used in the entire program or only in a specific part of the program.