JAVASCRIPT GET SET ATTRIBUTE
HTML Attribute And Javascript Properties
The HTML attribute is a special keyword which is used inside the opening tag of the element. The attribute is used to control the element's behaviour and to provide additional information to the element
The attribute is generally a name-value pair which is separated by =
sign.
When the browsers load HTML and generate DOM object from it, then most standard HTML attributes automatically become properties of the DOM objects.
For example, if the tag is <p title="paragraph title">
, then the DOM object has thisParagraph.title = "paragraph title"
.
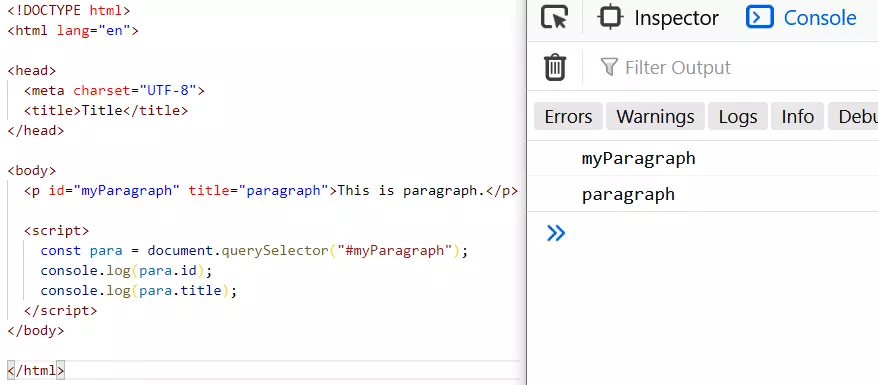
So using javascript you can access HTML element standard attributes as in the above picture.
Note: Every attribute of an element does not become DOM object property, only the standard attribute of the element becomes DOM object property.
From the above statement, you can easily understand that you can't access non-standard attributes since there won't be a DOM property for it.
So is there a way to access non-standard attributes?
Yes, Javascript provides some methods to access and manage such attributes. In this tutorial, we will see 4 different javascript method to handle attributes within the DOM.
javascript has attribute
The element.hasAttribute()
method is a javascript method to check whether a specific element has a specific attribute or not.
The method returns a boolean
value, true if attribute exist in the element and false if attribute does not exist.
hasAttribute Syntax:
const result = element.hasAttribute(attributeName);
hasAttribute Example:
const element = document.querySelector('p');
function check() {
if (element.hasAttribute('name')) {
alert("Yes 'name' attribute exist!");
}
else {
alert("No 'name' attribute doesn't exist!");
}
}
Try It
javascript get attribute
The getAttribute()
method is used to get the attribute value from any DOM element. This method returns the value of the attribute specified if the attribute doesn't exist then value returned is null or null
or ""
(empty string).
getAttribute Syntax
const value = element.getAttribute(attributeName);
Here: 'attributeName'' is the name of the attribute and 'value' is the value of the attribute.
When getAttribute
method is called on HTML element, the method lower-cases its argument before proceeding.
getAttribute Example
const element = document.querySelector('p');
function attr(elem) {
alert(elem.getAttribute('id'));
}
Try It
javascript set attribute
The setAttribute()
method is used to set new attribute and its value to the DOM element. If the attribute already exists then the value of the attribute is updated with the new value.
setAttribute Syntax
element.getAttribute(name, value);
This method takes 2 argument name and value. Here in the syntax: 'name' is the name of the attribute and 'value' is the value of the attribute.
setAttribute Examples
const element = document.querySelector("p");
function checkAttr() {
alert(element.hasAttribute("name"));
}
function addAttr() {
element.setAttribute("name", "myParagraph");
}
Try It
set boolean attribute javascript
Regardless of the actual value of Boolean attributes its presence make it to be true.
So while setting a boolean the attribute some people just pass attribute name, but it is a non-standard approach to do it. You should specify an empty string (""
) as a value.
Since value is converted to a string don't pass null
as a value because it will be converted to "null".
const element = document.querySelector("button");
function disableIt() {
element.setAttribute("disabled", "");
}
Try It
javascript remove attribute
The removeAttribute()
method is used to remove the attribute with the specific name from the element.
removeAttribute Syntax
const value = element.removeAttribute(attrName);
Here: 'attriName' is name of the attribute.
removeAttribute Example
const element = document.querySelector("button");
function remove() {
element.removeAttribute("title");
}
Try It