Array In JavaScript: Complete Guide
In this tutorial, you will learn about arrays in javascript, how to create them, how to access them, how to modify them, how to delete them, how to use them, and everything you need to know about them with perfect examples.
- Array In JavaScript
- Array Declaration
- Array Access
- Adding Elements
- Updating Elements
- Removing Elements
- Loop Over Array
- Multidimensional Arrays
- Find If it is Array
- Array Methods List
Table of Contents
JavaScript Array
An array is a collection of values. It is a list of items, where each item has a particular position in the list.
Array is a very powerful tool in programming. While writing programs and working with some data collection most of the time you would be dealing with the array.
JavaScript array can store a mixed data formats in a single array unlike C, C++ which store only a particular data format. This means you can store a string, number, boolean, object, array, etc in a single array.
Array makes accessing data really fast from a collection of data. The location of any element in an array is defined by its index value. Index number starts from 0 and goes to the length of the array. The complexity of accessing data from an array is O(1). Look in the image below.
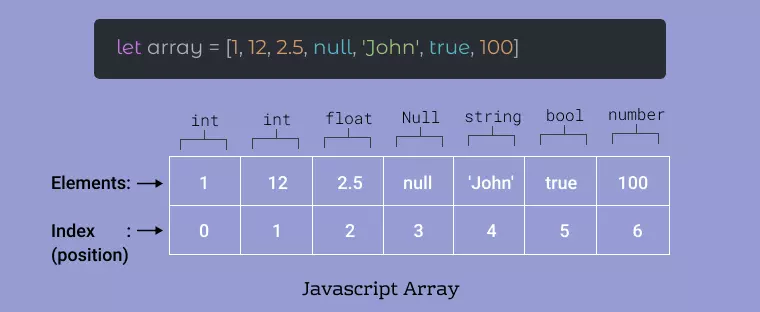
The array data stored in memory are in a contiguous memory location. This means within memory there is a contiguous block of memory that stores the array data. So, when you access any element in an array, you don't need to jump to another memory location to access the data. This is called linear access.
In javascript, array elements are enclosed in square brackets ([]). Example:
[1, 4, 8, 20, 16, ...]
Declare array in Javascript
An array can be defined in javascript in basically two different ways:
- Using Array literals
- Using constructor
1. Array literal
Array literal is the simplest and the most common way to define an array in javascript. Array literal is a list of values enclosed in square brackets ([]).
Array literal is so common that almost every javascript code has an array defined in it.
A list of values can be assigned to a variable using an array literal and then used in the code.
Here is an example to show how easy it is to declare an array using an array literal:
Example
// defining empty array
var empty = [];
console.log(empty);
// defining array with integers
var numbers = [1, 2, 3, 4, 5];
console.log(numbers);
// defining array with strings
var strings = ["Hello", "World"];
console.log(strings);
// defining array with mixed data
var mixed = [1, "Hello", true, [1, 2, 3], {a: 1, b: 2}];
console.log(mixed);
Try It
Note: Meanwhile you should also know to get the length of an array using the length property of the array.
Length of an array
You can use the length property on an array and it returns the number of elements in the array.
Example
// getting the length of an array
var numbers = [1, 2, 3, 4, 5];
console.log(numbers.length);
// mixed array
var arr = [1, 10.5, "Hello", true, [1, 2, 3], {a: 1, b: 2}];
console.log(arr.length);
Length of array is biggest index + 1.
To clear an array you can simply set its length to 0.
2. Array constructor
An array constructor is another way to define an array in javascript. It is a constructor function that creates an array.
An array constructor is a function that takes a variable number of arguments and returns an array. The function is called with the keyword new and the array constructor name.
It is a function that takes a variable number of arguments and returns an array.
For constructor array use var arr = new Array();
, this creates an array of length 0.
Example
// array with 0 length
var empty = new Array();
console.log(empty, empty.length);
// array with integer
var numbers = new Array(1, 2, 3, 4, 5);
console.log(numbers, numbers.length);
// mixed array
var mixed = new Array(1, "Hello", true, 2.5, [1, 2]);
console.log(mixed, mixed.length);
Try It
Array with a defined length: One of the cool things that you can do with a constructor array is to define the length of the array in advance.
The default elements filled inside the array are undefined
.
To do this you need to use var arr = new Array(length)
where length
is the length of the array.
Example
// array with length
var arr = new Array(5);
console.log(arr.length, arr[0]);
Accessing array elements
Accessing array elements is very easy in javascript. You can access any element in an array using its index number. The index number starts from 0 and goes to the length of the array.
So if you want to access the first element of an array, you can use arr[0]
, for the second element you can use arr[1]
, and so on.
Example
var arr = [1, 10, "Hello", true, 2.5];
// accessing first element
console.log(arr[0]); // 1
// accessing second element
console.log(arr[1]); // 10
// accessing last element
console.log(arr[arr.length - 1]); // 2.5
Try It
Adding Array Element
You can add new elements to an array at 3 different places:
- At the end of the array
- At the beginning of the array
- In the middle of the array
1. Adding element at the end of array
To add a new element at the end of an array, you can use arr.push(element)
where the element
is the element you want to add.
Example
var arr = [1, 10, "Hello", true, 2.5];
console.log(arr);
// adding element at the end of the array
arr.push(100);
console.log(arr);
2. Adding element at the beginning of array
To add a new element at the beginning of an array, you can use arr.unshift(element)
where the element
is the element you want to add.
Example
var arr = [1, 10, "Hello", true, 2.5];
console.log(arr);
// adding element at the beginning of the array
arr.unshift(100);
console.log(arr);
3. Adding element in the middle of array
To add a new element in the middle of an array, you can use arr.splice(index, 0, element)
where the index
is the index number of the element you want to add, 0
is the number of elements you want to remove and element
is the element you want to add.
This will shift all the elements after the index number to the right and add the new element in the middle.
Example
var arr = [1, 10, "Hello", true, 2.5];
console.log(arr);
// adding element at index number 2
arr.splice(2, 0, 100);
console.log(arr);
Update array element
To update an array element, you can use arr[index] = 'new value'
where the index
is the index number of the element you want to update.
Example
var arr = ["john", "smith", 23, 55, "hello"];
console.log(arr);
// updating element at index number 3
arr[3] = "world";
console.log(arr);
Try It
javascript array remove element
Removing an element from an array doesn't mean that you will update the element by 0 or null. It will still occupy the same space in the array.
To remove an element from an array, you can use arr.splice(index, 1)
where the index
is the index number of the element you want to remove.
The splice method will remove the element at the index number and shift all the elements after the index number to the left and also return the removed element.
Example
var arr = ["john", "smith", 23, 55, "hello"];
console.log(arr);
// removing element at index number 3
var removedElement = arr.splice(3, 1);
console.log(arr);
console.log(removedElement);
Removing the last element: There is a direct method to remove an element from the end of the array. You can use arr.pop()
where arr
is the array you want to remove the element from.
The pop method will return the removed element.
Example
var arr = ["john", "smith", 23, 55, "hello"];
console.log(arr);
// removing last element from the array
var removedElement = arr.pop();
console.log(arr);
console.log(removedElement);
loop through array
There are many ways to loop through an array but we are going to discuss the most common ways to loop through an array.
We are going to discuss 2 different ways to loop through an array:
- Using
for
loop - Using
forEach
loop
1. Array for loop
The most common way to loop through an array is using for loop. The syntax is:
for (var i = 0; i < arr.length; i++) { }
The for
loop will loop through all the elements of the array and will execute the code inside the curly braces.
Example
var arr = [1, 2, 3, 4, 5];
for (var i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
2. Array forEach loop
The forEach
loop is similar to the for
loop but more advanced. It accepts a function as a parameter and will execute that function for each element of the array.
The syntax is:
arr.forEach(function(element, index, array) { });
The first parameter is compulsory and other parameters are optional.
Example
var arr = [1, 12, 2.5, false, 100];
// using forEach loop
arr.forEach(function(element, index) {
console.log(element + " is at index " + index);
});
Javascript multidimensional array
Javascript arrays are multidimensional arrays. This means that you can have an array with multiple dimensions. For example, when you have an array like [1, 2, 3, 4, 5]
, it is a one-dimensional array. But when you have an array like [1, 2, [3, 4, 5]]
, it is a two-dimensional array.
If there are nested arrays inside the main array, it will be a multidimensional array.
// one dimensional array
var oneD = [1, 2, 3, 4, 5];
// two dimensional array
var twoD = [1, 2, [3, 4, 5]];
// three dimensional array
var threeD = [1, 2, [3, 4, [5, 6]]];
You can also create an array with multiple dimensions using the new Array()
method.
// one dimensional array
var oneD = new Array(1, 2, 3, 4, 5);
// two dimensional array
var twoD = new Array(1, 2, new Array(3, 4, 5));
// three dimensional array
var threeD = new Array(1, 2, new Array(3, 4, new Array(5, 6)));
To access the elements from multidimensional you may need more than one index number. See the example below:
Example
var arr = [1, true, [3, "Hello", 5]];
// accessing "Hello" from the array
console.log(arr[2][1]);
var multiD = [1, 5, [2.5, "Hello", [3, "World"]]];
// access "World" from the array
console.log(multiD[2][2][1]);
Since "Hello" is in an array whose index is 2 so first select the array and then select its position inside the array which is index value 1. And same for other selections.
Find if array or not
An array is an object in javascript, so if check typeof
array it says "object"
.
var arr = [1, 2, 3, 4, 5];
console.log(typeof arr);
So typeof
will confuse you if it's an array or not. To check if an array or not, you can use Array.isArray
method.
Example
var arr = [1, 2, 3, 4, 5];
console.log(Array.isArray(arr));
The Array.isArray
method will return true
if the parameter is an array and false
if it's not.
Another way to check if an array or not is to use instanceof
operator.
Example
var arr = [1, 2, 3, 4, 5];
console.log(arr instanceof Array);
Note: JavaScript does not support associative arrays. javascript always has numbered indexes for the array elements.
List of array methods
JavaScript array has lots of methods. You will learn about them in the coming sections.
Here is a list of array methods:
Method | Description |
---|---|
Array.length | Returns the number of elements in the array |
Array.push(element) | Adds an element to the end of the array |
Array.pop() | Removes the last element from the array and returns it |
Array.shift() | Removes the first element from the array and returns it |
Array.unshift(element) | Adds an element to the beginning of the array |
Array.splice(start, deleteCount, elements) | Changes the content of an array by removing existing elements and/or adding new elements |
Array.concat(array) | Returns a new array consisting of the array on which it is called joined with the array(s) and/or value(s) provided as arguments |
Array.join(separator) | Joins all elements of an array into a string |
Array.slice(start, end) | Returns a shallow copy of a portion of an array into a new array object |
Array.sort(compareFunction) | Sorts the elements of an array in place and returns the array |
Array.reverse() | Reverses the elements of an array in place and returns the array |
Array.indexOf(searchElement[, fromIndex]) | Returns the index of the first occurrence of a value in an array |
Array.lastIndexOf(searchElement[, fromIndex]) | Returns the index of the last occurrence of a specified value in an array |
Array.every(callbackfn[, thisArg]) | Calls a function for each element in an array and returns true if the function returns true for all elements |
Array.some(callbackfn[, thisArg]) | Calls a function for each element in an array and returns true if the function returns true for any element |
Array.forEach(callbackfn[, thisArg]) | Calls a function for each element in an array and returns the array |
Array.map(callbackfn[, thisArg]) | Calls a function for each element in an array and returns an array containing the return values |
Array.filter(callbackfn[, thisArg]) | Calls a function for each element in an array and returns an array containing the elements for which the function returned true |
Array.reduce(callbackfn[, initialValue]) | Calls a function for each element in an array and returns a single value constructed by concatenating the return values of the function |
Conclusions
In this section, you learned everything about javascript array to give a good start. We also looked at array methods that are specific to JavaScript.
We looked at various ways to create an array, loop through an array, check if an array is an array, and more.