Reverse for loop Python
In this tutorial, you will learn how to use for loop in reverse order in Python.
In python, for loop is used to iterate over a sequence (list, tuple, string) or other iterable objects. By default, the for loop iterates over the sequence in ascending order. Means it starts from the first element and ends at the last element.
However, you can also iterate over a sequence in reverse order. Starting from the last element and ending at the first element.
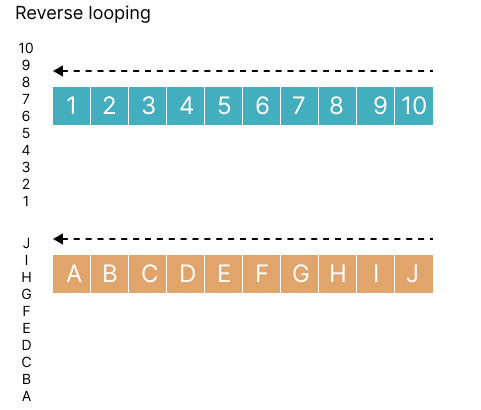
There can be multiple different approaches to iterate over a sequence in reverse order. Let's see them one by one.
1. Using reversed() function
The reversed() function returns a reverse iterator which can be used to iterate over a sequence in reverse order.
Here is an example of how to use reversed() function in for
loop.
my_list = [1, 2, 3, 4, 5]
# Iterate over the elements of the list in reverse order
for element in reversed(my_list):
print(element)
5 4 3 2 1
Here, we have created a list of numbers. Then we used reversed() function to iterate over the elements of the list in reverse order.
Note the original list is not modified by the reversed() function.
2. Reverse loop using negative step
Another way to create a reverse loop in Python is to use the built-in range() function with a negative step value.
For example range(5, 0, -1)
will return a list of numbers from 5 to 1 in reverse order.
# reverse for loop
for i in range(5, 0, -1):
print(i)
5 4 3 2 1
Here, the range() function starts at 5 and ends at 1. The step value is -1. So, effectively it iterates over the sequence in reverse order.
Another way of reversing the loop with a negative step is by using the len() function within the range() function as follows.
my_list = [1, 2, 3, 4, 5]
# Iterate over the elements of the list in reverse order
for i in range(len(my_list)-1, -1, -1):
print(my_list[i])
5 4 3 2 1
Here, we have used len() function to get the length of the list. Then we used the length of the list as the starting point of the range() function. The step value is -1. So, effectively it iterates over the sequence in reverse order.
This is all about for loop in reverse order in python. Hope you have learned something new today.
Happy coding!😊