Convert Python String To datetime
In this tutorial, you will learn how to convert Python String to datetime object. Additionally, you will also see a various different forms of string and converting them to datetime object as examples.
In software development one of the most common problems you may face is handling date and time.
At first glance, it may seem very simple but when you have a datetime string and you have to extract the date or time from it, you may face some issues, if you are not aware of how to handle datetime string.
When you have datetime string then to get any information from it, first, you have to convert it to datetime object.
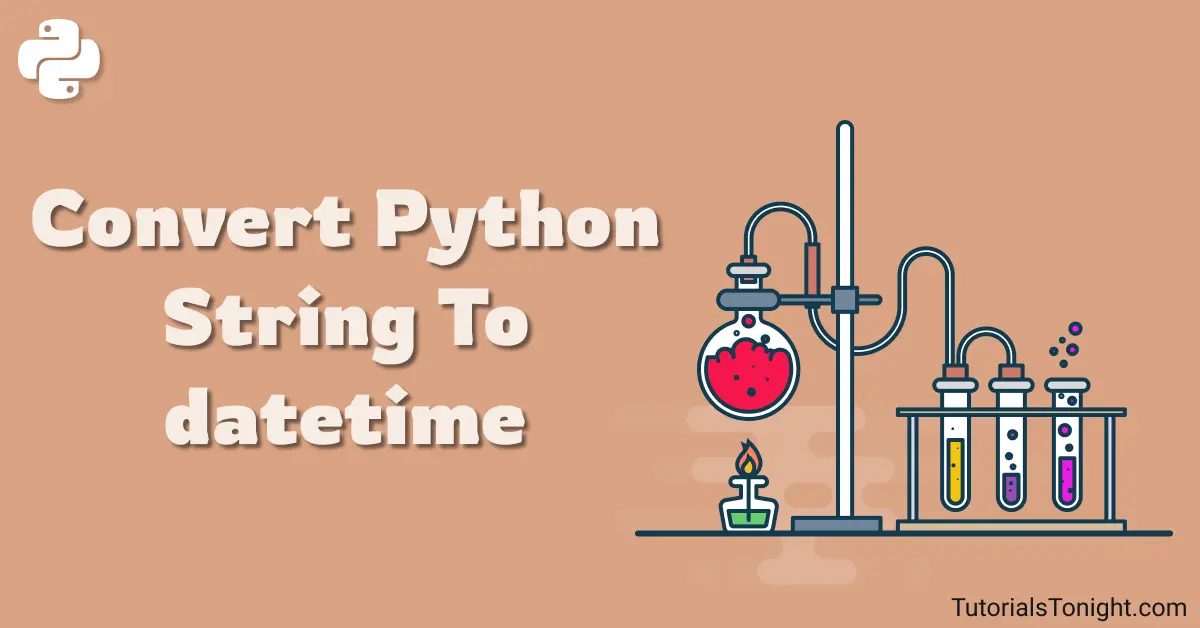
Luckily, Python provides a module called datetime that can be used to convert string to datetime object.
Let's see how to convert string to datetime object in Python.
- Quick Solution ⭐️
- String to datetime conversion
- Characters in the Format String
- Getting Date and Time from a String
- Multiple Examples
- 3rd party library: dateutil
- Conclusion
Table Of Contents
Quick Solution
For you in a hurry, here is a quick solution to convert string to datetime object.
For better understanding and detailed learning, you can proceed to the next section.
# Convert String to datetime object
import datetime as dt
# Example 1
str1 = '2020-01-01'
dt1 = dt.datetime.strptime(str1, '%Y-%m-%d')
print(dt1, type(dt1))
# Example 2
str2 = '1990-08-15 12:00:00'
dt2 = dt.datetime.strptime(str2, '%Y-%m-%d %H:%M:%S')
print(dt2, type(dt2))
# Example 3
str3 = 'Mon Feb 21 1920'
dt3 = dt.datetime.strptime(str3, '%a %b %d %Y')
print(dt3, type(dt3))
# Example 4
str4 = 'May 05 1995 12:00AM'
dt4 = dt.datetime.strptime(str4, '%B %d %Y %I:%M%p')
print(dt4, type(dt4))
The output of the above code will be as follows:
2020-01-01 00:00:00 <class 'datetime.datetime'> 1990-08-15 12:00:00 <class 'datetime.datetime'> 1920-02-21 00:00:00 <class 'datetime.datetime'> 1995-05-05 00:00:00 <class 'datetime.datetime'>
Python String To datetime
Why do we need to convert string to datetime object❓
A simple string is nothing but a sequence of characters. As a human, you may read and understand the date and time in the string but for a program to process it, use it, or to extract necessary data from it, it needs to be converted to a proper format (datetime).
How to convert string to datetime object❓🤔
Python provides a module called datetime. The datetime module provides a class called datetime and this class has a method called strptime that can be used to convert strings to datetime objects.

The strptime method accepts two arguments, the first one is the string to be converted and the second one is the format of the string.
The format of the string is a string that contains special characters that are used to define the format of the string. The special characters are arranged in the order of their appearance in the string.
Suppose you have a string '2020-01-01' then the format of the string is '%Y-%m-%d'. Where %Y is the year, %m is the month and %d is the day.
Let's look at a simple example to use the strptime method.
# import the datetime module
# rename the datetime module as dt
import datetime as dt
# using strptime method
str = '2022-05-10'
dtObject = dt.datetime.strptime(str, '%Y-%m-%d')
print(dtObject)
Output:
2022-05-10 00:00:00 <class 'datetime.datetime'>
To parse the string to datetime object, we have passed a formatting string that has the same order of characters as the elements in the string. '2022-05-10' matches the format '%Y-%m-%d'.
Look at the image below for a better understanding.
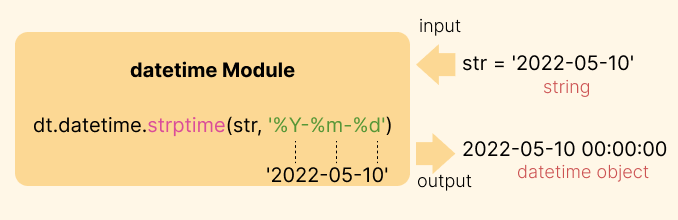
Characters in the Format String
Here is the list of the characters that can be used in the format string with their meaning.
Character | Meaning | Example |
---|---|---|
%a | Weekday, short version | Mon -> %a |
%A | Weekday, full version | Monday -> %A |
%b | Month, short version | Jan -> %b |
%B | Month, full version | January -> %B |
%d | Day of the month, zero-padded (01-31) | 01 -> %d |
%f | Microsecond (000000-999999) | 356172 -> %f |
%H | Hour (24-hour), zero-padded (00-23) | 15 -> %H |
%I | Hour (12-hour), zero-padded (01-12) | 03 -> %I |
%j | Day of the year (001-366) | 150 -> %j |
%m | Month (01-12) | 01 -> %m |
%M | Minute, zero-padded (00-59) | 15 -> %M |
%p | Locale's equivalent of either AM or PM | AM -> %p |
%S | Second, zero-padded (00-59) | 24 -> %S |
%U | Week number of the year (Sunday as the first day of the week), zero padded (00-53) | 10 -> %U |
%w | Weekday as a decimal number (0-6, Sunday is 0) | 3 -> %w |
%W | Week number of the year (Monday as the first day of the week), zero padded (00-53) | 10 -> %W |
%y | Year, last two digits (00-99) | 15 -> %y |
%Y | Year, full digits (0000-9999) | 2015 -> %Y |
%z | UTC offset in the form +HHMM or -HHMM | +0530 -> %z |
%Z | Time zone name | IST -> %Z |
%% | A literal '%' character | % -> %% |
Getting Date and Time from a String
To get the date or time from a string first we have to convert the string to datetime object. Because string has no property or method. It's just raw data or text.
Once we have the datetime object, we can apply the date() and time() method to get the date or time from the datetime object.
Let the date-time string be 'Jan 1, 2020 15:35:20', extract the date and time from it.
import datetime as dt
str = 'Jan 1, 2020 15:35:20'
# first, convert the string to datetime object
dtObject = dt.datetime.strptime(str, '%b %d, %Y %H:%M:%S')
# get the date from the datetime object
date = dtObject.date()
print(date)
# get the time from the datetime object
time = dtObject.time()
print(time)
Output:
2020-01-01 15:35:20
Here is another example to get the date from some other string.
import datetime as dt
str = 'Sunday, August 12, 2020 03:45AM'
# convert to datetime object
dtObject = dt.datetime.strptime(str, '%A, %B %d, %Y %I:%M%p')
# date
date = dtObject.date()
print(date)
# time
time = dtObject.time()
print(time)
Output:
2020-08-12 03:45:00
Multiple Examples For String To DateTime Conversion
Let's see various different types of date-time strings and convert them to datetime objects.
Example 1:
String of type = '2012/12/12'
import datetime as dt
str = '2012/07/12'
dtObject = dt.datetime.strptime(str, '%Y/%m/%d')
print(dtObject)
Output:
2012-07-12 00:00:00
Example 2:
String of type = 'Mon Feb 21 1920'
import datetime as dt
str = 'Mon Feb 21 1920'
dtObject = dt.datetime.strptime(str, '%a %b %d %Y')
print(dtObject)
Output:
1920-02-21 00:00:00
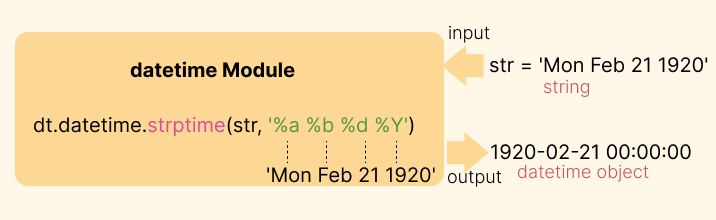
Example 3:
String of type = 'May 05 1995 05:00PM'
import datetime as dt
str = 'May 05 1995 05:00PM'
dtObject = dt.datetime.strptime(str, '%b %d %Y %I:%M%p')
print(dtObject)
Output:
1995-05-05 17:00:00
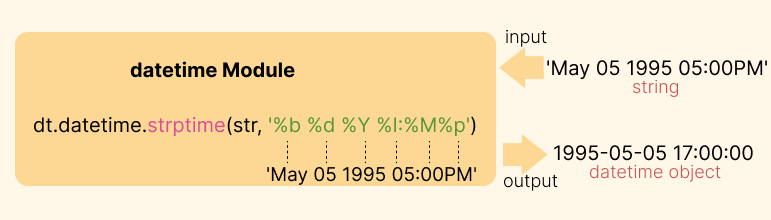
Example 4:
String of type = '2020-01-01 +0530'
import datetime as dt
str = '2020-01-01 +0530'
dtObject = dt.datetime.strptime(str, '%Y-%m-%d %z')
print(dtObject)
Output:
2020-01-01 00:00:00+05:30

Using 3rd party library: dateutil
The strptime() function can convert any string to datetime object but the problem with the above methods is that you have to create a perfect parsing string that matches the string you are trying to parse.
Here comes the dateutil library. It's a third-party Python library that provides a set of functions for working with dates and times.
It provides a parse() function that can parse any string to datetime object. You need not create the parsing string. It's done by the library itself.
dateutil is a vast library. We need to use only parsing functions. So just import parse function by from dateutil.parser import parse().
Let's see how to use the parse() function.
from dateutil.parser import parse
str = 'Jan 1, 2020 15:35:20'
# parse the string to datetime object
dtObject = parse(str)
print(dtObject)
Output:
2020-01-01 15:35:20
Here is another example to get the date from some other string.
from dateutil.parser import parse
date_str = [
'Sunday, August 12, 2020 03:45AM',
'Mon Feb 21 1920',
'May 05 1995 05:00PM',
'2020-01-01',
'Sun, 05/12/1999, 12:30PM',
'Mon, 21 March, 2015',
'2015-06-15T10:03:45Z'
]
for str in date_str:
dtObject = parse(str)
print(dtObject)
Output:
2020-08-12 03:45:00 1920-02-21 00:00:00 1995-05-05 17:00:00 2020-01-01 00:00:00 1999-05-12 12:30:00 2015-03-21 00:00:00 2015-06-15 10:03:45+00:00
Conclusion
String to datetime conversion is a very important task in the programming world. You may get datetime string from some other source like a database, file, return from an API, etc. You need to convert the string to datetime object to get the date, time, or other information from the string.
You can use the strptime() method from datetime module to convert the string to datetime object.