Python Dictionary Update
In this tutorial, you will learn how to update a dictionary in Python. You will learn how to add new key-value pairs to a dictionary and how to update existing items in a dictionary.
Dictionary is a mutable data type in Python. It means that we can change the contents of a dictionary after it has been created. We can add or update key-value pairs in a dictionary.
- update() method
- Update if Key Exists
- Alternative to update()
Table of Contents
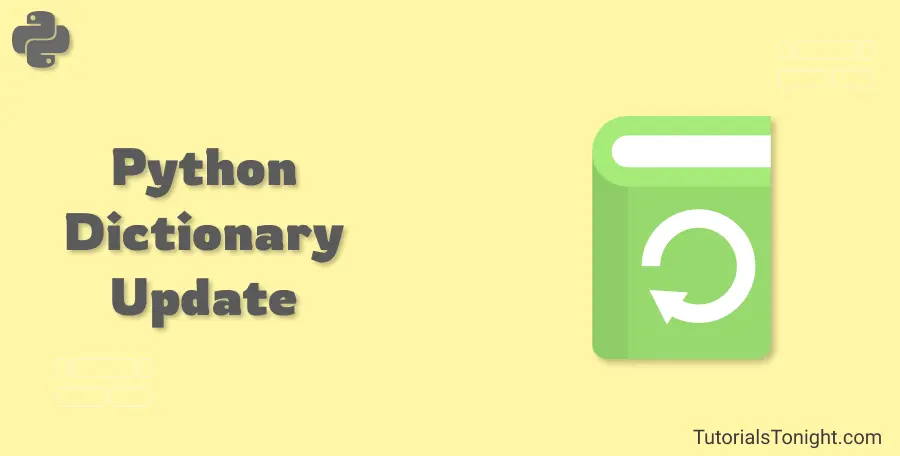
update() Method in Dictionary
The update() method in Python dictionary is used to add or update key-value pairs in a dictionary.
Syntax:
dict.update([other])
Parameters:
- other (optional) - dictionary or iterable of key/value pairs (default is None)
Example 1: Add new key-value pairs to a dictionary
If the key is not present in the dictionary, then the update() method adds the key-value pair to the dictionary.
Key in the dictionary below so, the item will be added
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3}
print("Before update:", dict1)
# add key-value pairs from dict2 to dict1
dict1.update(dict2)
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 2, 'c': 3}
Example 2: Update existing items in a dictionary
If the key is already present in the dictionary, then the update() method updates the value of the key with the new value.
Key in the dictionary below so, the item will be updated
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3}
print("Before update:", dict1)
# update value of key 'b' in dict1
dict1.update(dict2)
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 3}
Example 3: Add new key-value pairs to a dictionary using iterable
We can also add new key-value pairs to a dictionary using an iterable of key-value pairs (list of tuples).
Key in the dictionary below so, the item will be added
dict1 = {'a': 1, 'b': 2}
print("Before update:", dict1)
# add key-value pairs from iterable to dict1
dict1.update([('c', 3), ('d', 4)])
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 2, 'c': 3, 'd': 4}
Example 4: Update and add existing items in a dictionary using iterable
We can also update existing items in a dictionary using an iterable of key-value pairs (list of tuples).
Key in the dictionary below so, the item will be updated
dict1 = {'a': 1, 'b': 2}
print("Before update:", dict1)
# update value of key 'b' in dict1
dict1.update([('b', 3), ('c', 4)])
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 3, 'c': 4}
Example 5: Update and add items in a dictionary using keyword arguments
We can also update existing items in a dictionary using keyword arguments.
Key in the dictionary below so, the item will be updated
dict1 = {'a': 1, 'b': 2}
print("Before update:", dict1)
# update value of key 'b' in dict1
dict1.update(b=3, c=4)
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 3, 'c': 4}
Python Dictionary Update if Key Exists
To update a value in a dictionary only if the key exists, we can use an if-else statement in python to check if the key exists in the dictionary.
If the key exists, then we update the value of the key. Otherwise, we do nothing.
dict1 = {'a': 1, 'b': 2}
print("Before update:", dict1)
# update value of key 'b' in dict1
if 'b' in dict1:
dict1['b'] = 3
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 3}
Alternative to Python Dictionary Update
You can also update an item of a dictionary using the [ ] and = operators.
To update an item, we first access the item using the key and then assign a new value to it.
Let's see an example to understand this.
dict1 = {'a': 1, 'b': 2}
print("Before update:", dict1)
# update value of key 'b' in dict1
dict1['b'] = 3
print("After update:", dict1)
Before update: {'a': 1, 'b': 2} After update: {'a': 1, 'b': 3}
Frequently Asked Questions
Can Python dictionary be modified?
Yes, the Python dictionary is mutable. We can add, update, and delete items from a dictionary.
How do you update a dictionary in Python?
Use the update() method and pass the dictionary or iterable of key-value pairs as an argument (You can also use assignment operator). If the key is already present in the dictionary, then the update() method updates the value of the key with the new value. If the key is not present in the dictionary, then the update() method adds the key-value pair to the dictionary.
Does dictionary update overwrite?
Yes, if the key is already present in the dictionary, then the update() method updates the value of the key with the new value.