Nested For Loop in Python
In this article, we will learn about Nested For Loop in Python.
A nested for loop is a loop inside a loop. The inner loop executes for every iteration of the outer loop.
For example, if the outer loop executes 3 times and the inner loop executes 2 times, then the inner loop will execute 3 × 2 = 6 times in total.
The syntax of a nested for loop is as follows:
for i in range(outer_loop):
for j in range(inner_loop):
# do something
From the above syntax, we can see that the inner loop is indented inside the outer loop so that the inner loop executes for every iteration of the outer loop.
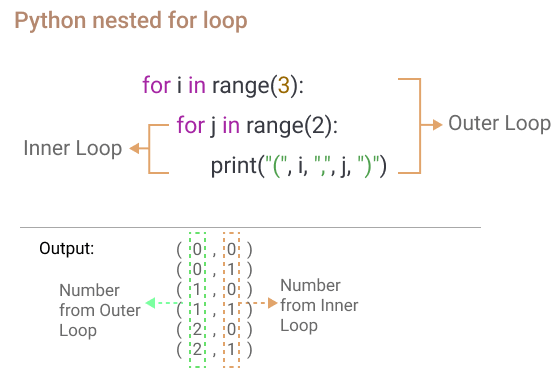
Let's see some examples of nested for loop in Python.
Nested For Loop Examples
Examples make things more clear. So, let's see some examples of nested for loop in Python.
Example 1
This is a simple example of a nested for loop that prints the execution point.
# Outer loop
for i in range(3):
print("Outer Loop: ", i,)
# Inner loop
for j in range(2):
print(" Inner Loop: ", j)
Output:
Outer Loop: 0 Inner Loop: 0 Inner Loop: 1 Outer Loop: 1 Inner Loop: 0 Inner Loop: 1 Outer Loop: 2 Inner Loop: 0 Inner Loop: 1
You can see in the output above that the inner loop executes for every iteration of the outer loop.
Example 2
This is another example of a nested for loop that prints the multiplication table of 1 to 5.
# Outer loop
for i in range(1, 6):
# Inner loop
for j in range(1, 11):
print(i*j, end=' ')
print()
Output:
1 2 3 4 5 6 7 8 9 10 2 4 6 8 10 12 14 16 18 20 3 6 9 12 15 18 21 24 27 30 4 8 12 16 20 24 28 32 36 40 5 10 15 20 25 30 35 40 45 50
When you look at the functioning of the outer loop you will observe that the outer loop executes the number of times as the number of rows in the multiplication table. And the inner loop executes 10 times to complete the multiplication table of a number.
Example 3
Let's use nested for loop to generate coordinates of a 2D plane (x, y) where x and y are in the range of 0 to 5 and x is greater than y.
# Outer loop
for i in range(6):
# Inner loop
for j in range(6):
if i > j:
print("(", i, ",", j, ")", sep='', end=' ')
print()
Output:
(1,0) (2,0) (2,1) (3,0) (3,1) (3,2) (4,0) (4,1) (4,2) (4,3) (5,0) (5,1) (5,2) (5,3) (5,4)
Example 4
Let's use nested for loop to generate a pyramid pattern in Python.
size = 5
# Outer loop
for i in range(size):
# Inner loop to print spaces
for j in range(size-i):
print(" ", end='')
# Inner loop to print stars
for k in range(i+1):
print("* ", end='')
print()
Output:
* * * * * * * * * * * * * * *
Example 5
The most commonly nested for loop is used in the operations of list in python. One such operation is sorting a list.
Let's see how we can sort a list using nested for loop.
num = [5, 2, 8, 1, 3]
print("Original List: ", num)
# sort the list
# using nested for loop
for i in range(len(num)):
for j in range(i+1, len(num)):
if num[i] > num[j]:
temp = num[i]
num[i] = num[j]
num[j] = temp
print("Sorted List: ", num)
Output:
Original List: [5, 2, 8, 1, 3] Sorted List: [1, 2, 3, 5, 8]
Nested For Loop with Break and Continue
break and continue statements can be used in nested for loop as well.
break and continue statements are used to control the flow of the loop. break statement is used to terminate the loop and continue statement is used to skip the current iteration of the loop.
Let's see some examples of nested for loop with break and continue.
Break nested for loop
In the following example, we will use the break statement to terminate the outer loop when the inner loop is executed 3 times.
# Outer loop
count = 0
for i in range(3):
print("Outer Loop: ", i,)
# Inner loop
for j in range(3):
print(" Inner Loop: ", j)
count += 1
if count == 3:
break
Output:
Outer Loop: 0 Inner Loop: 0 Inner Loop: 1 Inner Loop: 2
As you can see in the output above, the outer loop is terminated after the inner loop is executed 3 times.
Continue nested for loop
In the following example, we will use the continue statement to skip the current iteration if the number is divisible by 2.
# Outer loop
for i in range(1, 6):
# Inner loop
for j in range(1, 6):
if (i*j) % 2 == 0:
continue
print(i*j, end=' ')
print()
Output:
1 3 5 3 9 15 5 15 25
As you can see in the output above, the numbers that are divisible by 2 are skipped.
Conclusion
So, this is all about nested for loop in Python. Go through the examples, modify them, and print elements at different stages of the loop to get a better understanding.