Sort Dictionary by Value Python
Python Dictionary is used to store data in key-value pairs. However, the dictionary is unordered, you do not have any control over the order of the data stored in the dictionary.
But sometimes you need a proper order in your dictionary to perform some operations like task scheduling, inventory management, etc.
Here you will learn how to sort a dictionary by value in Python in both ascending and descending order in detail with examples.
At the end of this tutorial, we will also compare the speed of different methods discussed here to sort a dictionary by value.
1. How to sort a dictionary by value in Python?
The quickest and easiest way to sort a dictionary by value in Python is to use the sorted() function.
The sorted() function takes 3 arguments:
- iterable - The sequence to sort, list, dictionary, tuple, etc.
- key - A function that is called on each element of the iterable. The value returned by the function is used for sorting.
- reverse (optional) - If set to True, the iterable is sorted in reverse order. By default, it is set to False.
sorted(iterable, key, reverse)
Here is an example of sorting a dictionary using the sorted() function.
my_dict = {'a': 4, 'b': 3, 'c': 5, 'd': 1, 'e': 2}
# sort the dictionary by value
sort = sorted(my_dict, key=my_dict.get)
print(sort)
Output:
['d', 'e', 'b', 'a', 'c']
You can see that the dictionary is sorted by value in ascending order. The sorted() function returns a list of keys in sorted order.
Getting the sorted dictionary
If you want to get entire key-value pairs in sorted order, then you can pass my_dict.items() as the first argument to the sorted() function and lambda item: item[1] as the second argument to the sorted() function to sort the dictionary by value.
my_dict = {'a': 4, 'b': 3, 'c': 5, 'd': 1, 'e': 2}
# sort the dictionary by value
sort = sorted(my_dict.items(), key=lambda item: item[1])
# convert the sorted tuple into a dictionary
sorted_dict = dict(sort)
print(sorted_dict)
Output:
{'d': 1, 'e': 2, 'b': 3, 'a': 4, 'c': 5}
Sort dictionary by value in descending order
To sort a dictionary by value in descending order, you just need to set the reverse argument of the sorted() function to True.
my_dict = {'a': 4, 'b': 3, 'c': 5, 'd': 1, 'e': 2}
# sort the dictionary by value in descending order
sort = sorted(my_dict.items(), key=lambda item: item[1], reverse=True)
# convert the sorted tuple into a dictionary
sorted_dict = dict(sort)
print(sorted_dict)
Output:
{'c': 5, 'a': 4, 'b': 3, 'e': 2, 'd': 1}
2. Sort dictionary by value using operator module
Instead of using the lambda function, you can also use the itemgetter() function from the operator module to sort a dictionary by value.
The itemgetter() function takes the index as an argument and returns a function that fetches the item from its operand using the index.
from operator import itemgetter
my_dict = {'a': 4, 'b': 3, 'c': 5, 'd': 1, 'e': 2}
# sort the dictionary by value
sort = sorted(my_dict.items(), key=itemgetter(1))
# convert the sorted tuple into a dictionary
sorted_dict = dict(sort)
print(sorted_dict)
Output:
{'d': 1, 'e': 2, 'b': 3, 'a': 4, 'c': 5}
3. Using pandas module
The pandas module provides a vast variety of functions to perform various operations on data.
One of the functions is the sort_values() function which can be used to sort a dictionary by value.
It takes 2 arguments:
- by - The column name to sort by.
- ascending (optional) - If set to True, the dictionary is sorted in ascending order. By default, it is set to True.
First convert the dictionary into a DataFrame object and then use the sort_values() function to sort the dictionary by value.
import pandas as pd
my_dict = {'a': 4, 'b': 3, 'c': 5, 'd': 1, 'e': 2}
# convert the dictionary into a DataFrame object
df = pd.DataFrame(my_dict.items(), columns=['key', 'value'])
# sort the dictionary by value
df = df.sort_values(by='value')
# convert the DataFrame object into a dictionary
sorted_dict = dict(zip(df.key, df.value))
print(sorted_dict)
Output:
{'d': 1, 'e': 2, 'b': 3, 'a': 4, 'c': 5}
Conclusion
By now you must have learned how to sort a dictionary by value in Python.
Let's compare the speed of different methods discussed here to sort a dictionary by value.
Here is the image of the speed comparison of above discussed methods.
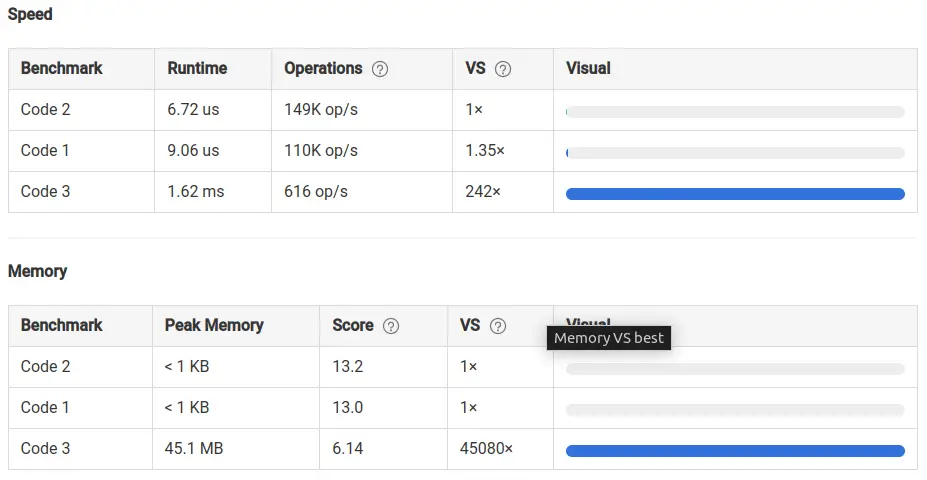
As you can see, Method 2 is the fastest to sort a dictionary by value in Python.