Python Count Duplicates in List
Duplicate elements in a list are those elements which occur more than once in the list.
For example, if we have a list ['a', 'b', 'c', 'd', 'a', 'b']
, then 'a'
and 'b'
are duplicate elements.
In this tutorial, we will learn how to count the number of duplicate elements in a list in Python.
Speed comparison of each method is also given at the end of this article.
1. Using nested for loop
We can use a nested for loop to iterate over the list and check if the current element is present in the rest of the list or not.
If the element is present in the rest of the list, then we will increase the count by 1.
Let's see this in action.
my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e']
count = 0
# looping through each element of the list
for num in my_list:
for dup in my_list:
# increase count if element is duplicate
if num == dup:
count += 1
if count > 1:
print(num, count)
# reset count to 0
count = 0
Output
a 3 b 2 a 3 c 2 b 2 a 3 c 2
You can see that the above code is printing the duplicate elements along with their count.
But the problem with this approach is that it is printing the duplicate elements multiple times also it is not very efficient.
2. ⭐️⭐️⭐️Using Dictionary
To fix above problem, we can use a dictionary to store the count of each element.
Later we can iterate over the dictionary and print the elements with count greater than 1.
my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e']
# creating an empty dictionary
duplicates = {}
# looping through each element of the list
for num in my_list:
# checking whether it is in the dictionary or not
if num in duplicates:
# increasing count if present
duplicates[num] += 1
else:
# initializing count to 1 if not present
duplicates[num] = 1
# printing the element with count greater than 1
for key, value in duplicates.items():
if value > 1:
print(key, value)
Output
a 3 b 2 c 2
This approach is much better than the previous one, but still, it is not the best approach.
3. Using Dictionary Comprehension
Dictionary Comprehension is a quick and easy way to create dictionaries in Python.
We can use dictionary comprehension to create a dictionary with elements as keys and their count as values.
my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e']
# creating a dictionary with elements
# as keys and their count as values
duplicates = {num: my_list.count(num) for num in my_list}
# printing the element with count greater than 1
for key, value in duplicates.items():
if value > 1:
print(key, value)
Output
a 3 b 2 c 2
4. Using Counter from collections module
Counter is a subclass of dictionary object which helps to count hashable objects.
It is an unordered collection where elements are stored as dictionary keys and their count as dictionary value.
We can use Counter
to count the number of duplicate elements in a list.
from collections import Counter
my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e']
# creating a dictionary with elements
# as keys and their count as values
duplicates = Counter(my_list)
# printing the element with count greater than 1
for key, value in duplicates.items():
if value > 1:
print(key, value)
Output
a 3 b 2 c 2
5. Using set()
We can use set()
to remove duplicate elements from the list.
Then we can use count()
method to count the number of occurrences of each element in the list.
my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e']
# removing duplicate elements from the list
duplicates = set([num for num in my_list if my_list.count(num) > 1])
# printing the element with their count
for num in duplicates:
print(num, my_list.count(num))
Output
b 2 c 2 a 3
Counting total number of duplicate elements
Now, if you want to count the total number of duplicate elements in a list, then you can use the set()
method to remove duplicate elements from the list.
Then you can use the len()
method to count the number of elements in the list.
my_list = ['a', 'b', 'a', 'c', 'd', 'b', 'a', 'c', 'e']
# removing duplicate elements from the list
duplicates = set(my_list)
# printing the total number of duplicate elements
print(len(my_list) - len(duplicates))
Output
6
Conclusion
So these were some of the ways to count the number of duplicate elements in a list in Python.
There are many other ways to do this, but these are the most efficient and easy to understand.
Among all these methods, the fastest method is using 2nd method i.e. using dictionary.
Here is an image to compare the speed of all the methods.
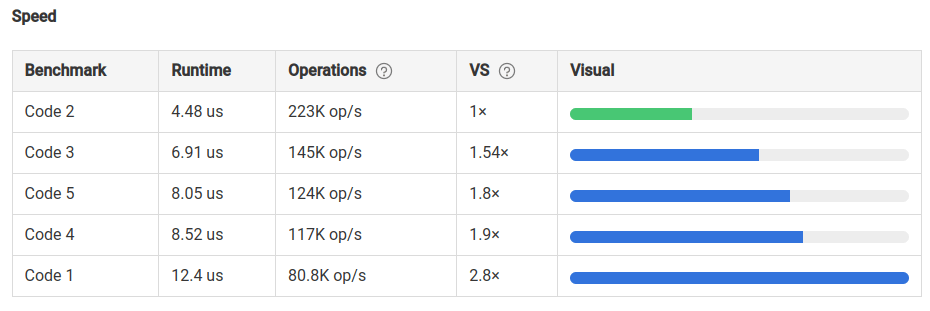