Password Generator Python
In this tutorial, you will learn how to create a password generator in python. We will first create a simple password generator and then we will create a strong password generator.
Let's start with a question, why do we need passwords?🤔
Well, the answer is simple, we need passwords to protect🛡 our data. Passwords are something that is used to protect our data from unauthorized access.
Password generator helps you create secure and strong passwords that are hard to guess.
So, if you are a beginner and want to learn how to create a password generator in python, then you are at the right place.
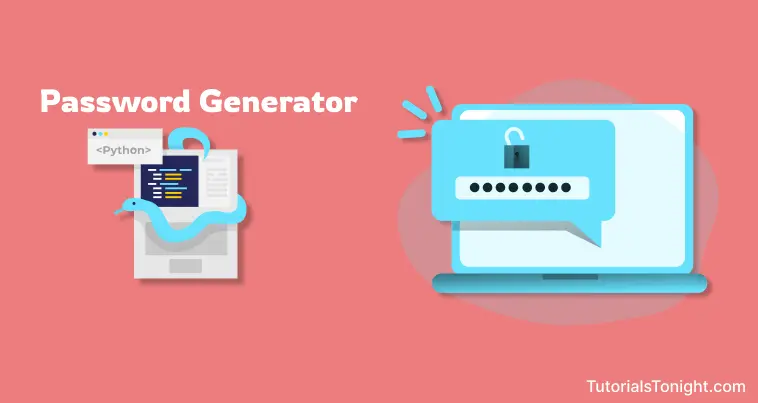
- Simple password generator
- Strong password generator
- Password strength checker
- Conclusion
Table of Contents
Simple Password Generator
Let's start with the simple password generator.
In a simple password generator, we will create a password of random size. The password will contain both uppercase and lowercase letters, numbers, and special characters.
To generate a random password you must first know how to generate random numbers in python.
Here are steps to create a simple password generator in python.
- Start with importing the random module to deal with random operations.
- Also, import the string module. It contains all the characters that we will use to create our password.
- Now, create a python function that takes the length of the password as an argument.
- Within the function create a variable that stores all the characters by adding uppercase letters, lowercase letters, numbers, and special characters. We will choose the characters from this variable randomly.
- Create a variable that stores the password. It will be an empty string.
- Now, create a for loop that runs for the number of times equal to the length of the password and in each iteration, it will choose a random character from the variable that stores all the characters and add it to the variable that stores the password.
- Finally, return the password.
import random
import string
upper = string.ascii_uppercase
lower = string.ascii_lowercase
digits = string.digits
symbols = string.punctuation
# password generator function
def password_generator(size):
chars = upper + lower + digits + symbols
password = ''
for i in range(size):
password += random.choice(chars)
return password
print(password_generator(8))
print(password_generator(12))
gE!Zn].X s%DVO!V#u4P|
Note: The output of the password generator will be different every time you run the program.
Strong Password Generator in Python
In the previous section, we created a simple password generator. It has some limitations. What if we do not want to use special characters in our password? What if we want to use only uppercase letters and numbers? What if we want to use only lowercase letters and numbers?
Also what if we want at least one uppercase letter or one special character in our password?
These are some of the limitations of the simple password generator. To overcome these limitations we will create a strong password generator.
Here are steps to create a strong password generator in python.
- Import both the random and string modules just like we did in the previous section.
- Now, create a python function that takes 5 arguments: length of password, uppercase, lowercase, digits, and symbols.
- Use boolean values to control the types of characters in the password. If the value is True then the character will be included in the password. If the value is False then the character will not be included in the password.
- Again use the if-else statement to add at least one character of each type in the password.
- Now, use a for loop to add the rest of the characters in the password.
- Finally, shuffle the characters in the password and return it.
import random
import string
# advance password generator
# control the number of each type of character
def password_generator(size, upper=1, lower=1, digits=1, symbols=1):
chars = ''
password = ''
if upper:
chars += string.ascii_uppercase
if lower:
chars += string.ascii_lowercase
if digits:
chars += string.digits
if symbols:
chars += string.punctuation
# make sure the password has at least one of each type of character
if upper:
password += random.choice(string.ascii_uppercase)
if lower:
password += random.choice(string.ascii_lowercase)
if digits:
password += random.choice(string.digits)
if symbols:
password += random.choice(string.punctuation)
# add the rest of the characters
for i in range(size - 4):
password += random.choice(chars)
# shuffle the characters
password = list(password)
random.shuffle(password)
password = ''.join(password)
return password
print(password_generator(16, True, True, True, True))
print(password_generator(8, False, True, False, True))
VSoR[KSVjt')cl02 y+qz.@
In the above function, we used if-else statements with the same condition 2 times for different purposes just for your clear understanding. You can merge both statements into one. This will also make your code shorter.
Password Strength Checker in Python
We were generating random passwords in the previous section. Then why not check the strength of the password?
Here is the program that checks the strength of the password.
# Password Strength Checker in Python
def password_strength(password):
strength = 0
if len(password) >= 8:
strength += 1
if any(c.isupper() for c in password):
strength += 1
if any(c.islower() for c in password):
strength += 1
if any(c.isdigit() for c in password):
strength += 1
if any(c in string.punctuation for c in password):
strength += 1
if strength == 1:
return 'Weak'
elif strength == 2:
return 'Medium'
elif strength == 3:
return 'Strong'
elif strength == 4:
return 'Very Strong'
elif strength == 5:
return 'Extremely Strong'
# get the password from the user
password = input('Enter your password: ')
print('Password Strength:', password_strength(password))
Enter your password: 12345678 Password Strength: Weak Enter your password: 12345678A Password Strength: Medium Enter your password: VSoR[KSVjt')cl02@ Password Strength: Extremely Strong
Conclusion
Now you have programs to generate both simple and strong passwords in python. You can also check the strength of the password.
Hope you enjoyed this tutorial. Play around with the code and try something new.
Happy Coding!😊