Python Touch File (Touch Module)
Creating and managing files is an important part of any programming language. There is a third-party module called touch which is used to create a file in python.
But this module have some compatibility issues with different Python versions. Also there are limitations to what you can do with this module.
So we will use different ways to create a file using built-in functions and modules in python.
Creating a File
To create a file we are going to use os module. It provides a function called open() which we can use to create a file.
It accepts two arguments:
- File name
- Mode in which we want to open the file, like read (r), write (w), append (a) etc.
Let's see how we can create a file using open() function.
import os
# open file in write mode
# if file doesn't exist, it will be created
file = open("newfile.txt", "w")
Output:
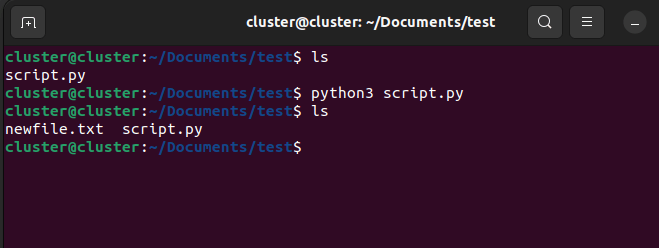
Here, we have created a file named newfile.txt in the current directory. The file is opened in write mode.
Now, if you check your current directory, you will find a file named newfile.txt in it.
Note: If you don't provide any path, the file will be created in the current directory.
Creating a File in a Specific Directory
To create a file in a specific directory, you need to provide the path of the directory in which you want to create the file.
Let's see how we can create a file in a specific directory.
import os
# file path
path = "/home/username/Documents/"
# open file in write mode
file = open(path + "newfile.txt", "w")
Output:
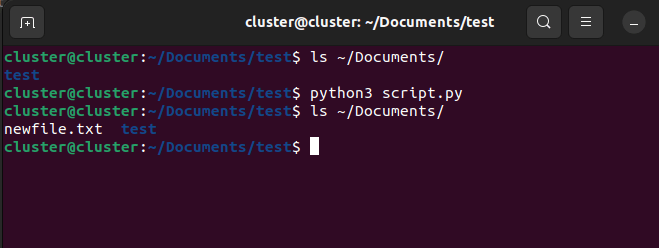
Run your program and check the directory, you will find a file named newfile.txt in it.
Creating Multiple Files
Now, let's see how we can create multiple files using open() function.
For this you can either store the file names in a list and loop through it or you can dynamically create the file names.
Let's see both the methods.
Method 1: Using List
Let's see how we can create multiple files using a list.
import os
# list of file names
files = ["file1.txt", "file2.txt", "file3.txt"]
# loop through the list
for file in files:
# open file in write mode
file = open(file, "w")
Output:
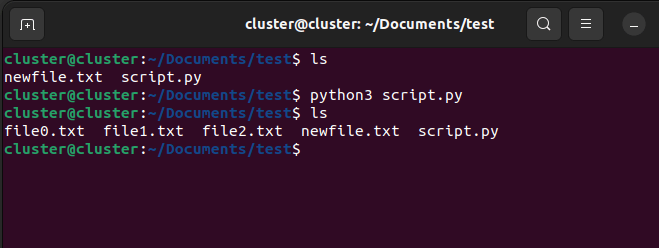
Here, we have created three files named file1.txt, file2.txt and file3.txt in the current directory.
Method 2: Dynamically Creating File Names
Let's see how we can create multiple files by dynamically creating the file names.
import os
# loop 3 times
for i in range(3):
# open file in write mode
file = open("file" + str(i) + ".txt", "w")
Output:
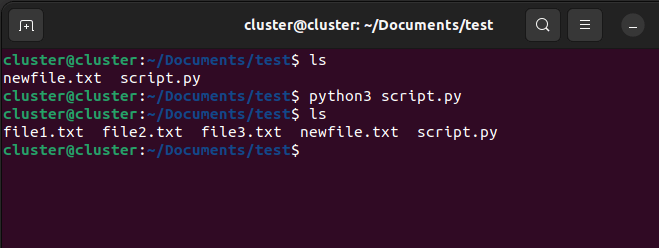
Here, we have created three files named file0.txt, file1.txt and file2.txt in the current directory.
Conclusion
Using open() function you can now create file or multiple files in any given directory both dynamically and through a list.
This skill will come in handy when you will be working on projects.
Happy Learning!