Remove Element From List Python
We have already seen how to add an element to the list in Python. Learning to remove elements from the list will help you to manipulate the list according to your needs.
- Quick solution
- 3 Ways to remove element from list
- Remove the element by index
- Remove the element by value
- Remove all occurrences of an element
- Conclusion
Table Of Contents
Quick Solution
Here is the quick solution to your problem for detailed learning you can proceed further.
In python you can use 3 different ways to remove element from list:
- remove() Method
- pop() Method
- del keyword
# python remove element from list
list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# 1. remove()
# remove method removes the first occurrence
# of the specified value from the list
list.remove(5)
print(list) # 5 is removed from the list
# 2. pop()
# pop method removes the last element from the list
list.pop()
print(list) # 10 is removed from the list
# pass index to pop() method
list.pop(0)
print(list) # 1 is removed from the list
# 3. del keyword
# del keyword removes the specified index from the list
del list[0]
print(list) # 1 is removed from the list
Here is the output of the above code.
[1, 2, 3, 4, 6, 7, 8, 9, 10] [1, 2, 3, 4, 6, 7, 8, 9] [2, 3, 4, 6, 7, 8, 9] [3, 4, 6, 7, 8, 9]
3 Ways to remove element from the list
Python provides 3 different ways to remove elements from the list. Let's see all these methods in detail.
1. remove() Method
The remove() is a list method that removes the first occurrence of the specified value from the list.
The value which is to be removed from the list is passed as an argument to the method.
Let's see an example:
# remove element from the list
# using remove() method
nums = [10, 5, 8, 2, 10, 3, 5]
# remove 10 from the list
nums.remove(10)
print(nums) # 1st occurrence of 10 is removed from the list
Output:
[5, 8, 2, 10, 3, 5]
2. pop() Method
pop() another list method. It is famously used to remove the last element from the list.
However, in python, you can also pass the index to pop() method to remove the element from the list at the specified index.
# remove last element from the list
# using pop() method
nums = [10, 5, 8, 2, 10, 3, 5]
print("Original list:", nums)
# remove last
nums.pop()
print(nums)
# remove at index 2
nums.pop(2)
print(nums)
Output:
Original list: [10, 5, 8, 2, 10, 3, 5] [10, 5, 8, 2, 10, 3] [10, 5, 2, 10, 3]
3. del keyword
The del keyword is used to remove the specified index from the list.
The del keyword proceeds to the element of the list accessed by the index.
nums = [10, 5, 8, 2, 10, 3, 5]
# using del keyword
del nums[0]
print(nums)
del nums[2]
print(nums)
Output:
[5, 8, 2, 10, 3, 5] [5, 8, 10, 3, 5]
Speed Comparison
Let's compare the speed of these 3 methods to remove elements from the list.
The following image shows the time taken by each method and you can see that the pop() method is the fastest among all.
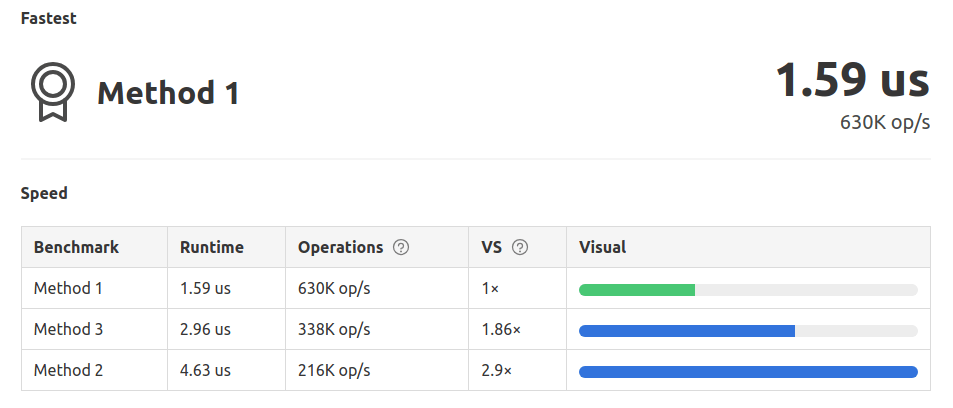
Remove element by index
You can use the pop() method and del keyword to remove elements from the list by index.
Pass the index of the element to the pop() method to remove the element from the list. If the element is not present in the list, it will throw an error.
Similarly, use the del keyword and refer to the list element by index to remove the element from the list.
# remove element by index
nums = [10, 5, 8, 2, 10, 3, 5]
# Method 1: pop()
nums.pop(2)
print(nums)
# Method 2: del keyword
del nums[2]
print(nums)
Output:
[10, 5, 2, 10, 3, 5] [10, 5, 10, 3, 5]
Remove the first element from the list
To remove the first element from the list, you can use list.pop(0) or del list[0].
# remove first element from the list
nums = [10, 5, 8, 2, 10, 3, 5]
# using pop() method
nums.pop(0)
print(nums)
# using del keyword
del nums[0]
print(nums)
Output:
[5, 8, 2, 10, 3, 5] [8, 2, 10, 3, 5]
Remove last element from the list
To remove last element from the list, you can use list.pop() or del list[-1].
# remove last element from the list
nums = [10, 5, 8, 2, 10, 3, 5]
# using pop() method
nums.pop()
print(nums)
# using del keyword
del nums[-1]
print(nums)
Output:
[10, 5, 8, 2, 10, 3] [10, 5, 8, 2, 10]
Remove element by value
You can use remove() method and pass the value of the element you ant to remove to the method.
If the value is not present in list, it will throw an error.
# remove element by value
nums = [10, 5, 8, 2, 10, 3, 5]
# remove 8
nums.remove(8)
print(nums)
# remove 10
nums.remove(10)
print(nums)
Output:
[10, 5, 2, 10, 3, 5] [5, 2, 10, 3, 5]
Remove all occurrences of an element
To remove all the occurrences of an element from the list, you need to loop through the list and check if the number of element is greater than 0. If it is greater than 0, then remove the element from the list.
# remove all occurrences of an element
nums = [10, 5, 8, 2, 10, 3, 5]
# remove all occurrences of 10
while nums.count(10) > 0:
nums.remove(10)
print(nums)
Conclusion
This brief guide taught you how python remove an element from the list.
The 3 methods discussed in this guide are:
- pop() Method
- del keyword
- remove() Method