Python Add Element To List
In the article python add to list, you will learn how to add an element to a list in Python. An element can be a number, string, list, dictionary, tuple, or even another list.
A list is a special data type in Python. It is a collection of items, which are separated by commas. It is also known as a sequence or array in other programming languages.
A list is a mutable data type in python. It means that you can add a new element or change it in any form.
An example of a list is:
>>> list1 = [1, 2, 3, 4, 5]
>>> list2 = ['a', 10, True, -1.2]
- Quick Solution
- Methods To Add To List
- Python add to list if not in list
- Add List To Another List
- Conclusion
Table Of Contents - Python Add To List
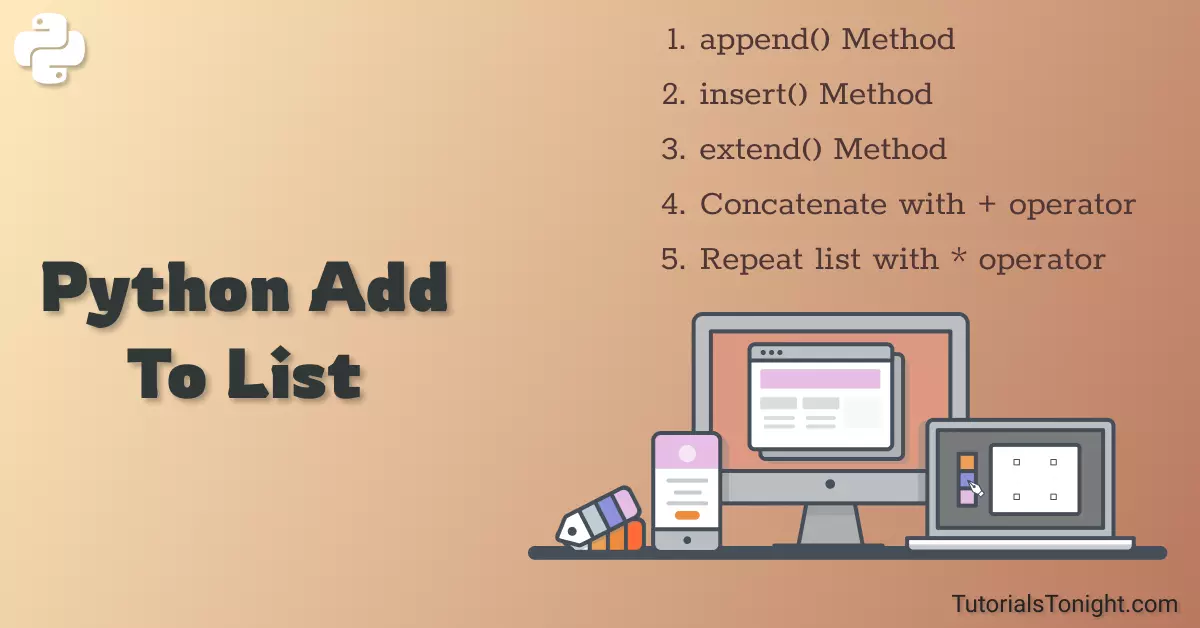
# quick solution: Python Add To List
For a quick solution, you can use the append() method to add an element to the list. You will learn about this method in detail later in this article.
The append() method accepts one argument, which is the element you want to add to the list. The function adds the element to the end of the list.
numbers = [1, 2, 3, 4, 5]
# add 6 to the end of the list
numbers.append(6)
print(numbers)
The output of the above code is:
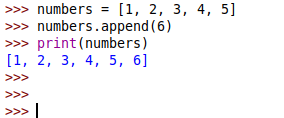
Methods To Add Items To Python List
You have seen the append() methods above in the quick solution section to add an element to the list. It is one of many methods to add an element to the list.
Let's see all methods to add items to the list with examples in detail.
1. append() Method
The append() is a list method in python that adds an element to the end of the list.
It accepts the adding element as an argument and appends it as the last element of the list.
Syntax:
list.append(element)
Here list is the list you want to add an element to and the element is the element you want to add to the list.
Here is an example:
# python add to list
numbers = [1, 2, 3]
print(f"Before appending: {numbers}")
# append 4 to list
numbers.append(4)
print(f"After appending: {numbers}")
Output
Before appending: [1, 2, 3] After appending: [1, 2, 3, 4]
Here is another example to add a string element to the list:
fruit = ['apple', 'banana']
print(f"Before appending: {fruit}")
# append 'orange' to list
fruit.append('orange')
print(f"After appending: {fruit}")
Output
Before appending: ['apple', 'banana'] After appending: ['apple', 'banana', 'orange']
2. insert() Method
The insert() method is also a list method to add an element to the list.
Unlike the append() method which adds elements only at the end of the list, the insert() method adds an element at a specific index of the list.
Here is the syntax of insert() method:
list.insert(index, element)
The method accepts 2 arguments - index and element. The index is the position of the element you want to add to the list. The element is the element you want to add to the list.
Let's see an example:
number = [1, 2, 3]
print(f"Before inserting: {number}")
# insert 4 at index 1
number.insert(1, 4)
print(f"After inserting: {number}")
Output
Before inserting: [1, 2, 3] After inserting: [1, 4, 2, 3]
Here is another example using string:
fruit = ['apple', 'banana', 'cherry']
print(f"Before inserting: {fruit}")
# insert 'orange' at index 2
fruit.insert(2, 'orange')
print(f"After inserting: {fruit}")
Output
Before inserting: ['apple', 'banana', 'cherry'] After inserting: ['apple', 'banana', 'orange', 'cherry']
3. extend() Method
The extend() method is used to extend the list by adding all elements of another list, or sequence, to the end of the list.
It can add both single and multiple elements to the list.
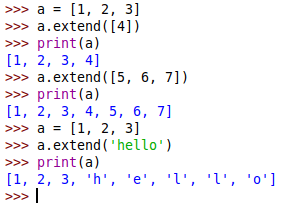
Syntax:
list.extend(sequence)
Here list is the list you are adding elements to and sequence is any valid sequence (list, tuple, string, range, etc.)
Here is an example of extending a list:
# python add to list using extend()
numbers = [1, 2, 3]
print(f"Before extending: {numbers}")
number.extend([4, 5, 6])
print(f"After extending: {numbers}")
Output
Before extending: [1, 2, 3] After extending: [1, 2, 3, 4, 5, 6]
Extending other sequences like strings, range, etc. is also possible:
# extending tuple
a = [1, 2, 3]
print(f"Before extending tuple: {a}")
a.extend(('a', 'b', 'c'))
print(f"After extending tuple: {a}")
# extending string
a = [1, 2]
print(f"Before extending string: {a}")
a.extend('hello')
print(f"After extending string: {a}")
# extending range
a = [1, 2]
print(f"Before extending range: {a}")
a.extend(range(10, 15))
print(f"After extending range: {a}")
Output
Before extending tuple: [1, 2, 3] After extending tuple: [1, 2, 3, 'a', 'b', 'c'] Before extending string: [1, 2] After extending string: [1, 2, 'h', 'e', 'l', 'l', 'o'] Before extending range: [1, 2] After extending range: [1, 2, 10, 11, 12, 13, 14]
Check out difference between extend and append.
4. Add element using + operator
The + operator can be used to add two lists together. The result is a new list that has the elements of both lists.
Here is an example:
# python add two lists
a = [1, 2, 3]
b = ['a', 'b', 'c']
# add two lists together
c = a + b
print(c)
Output
[1, 2, 3, 'a', 'b', 'c']
However, if you try to add a string or a number to a list, the result will be an error.
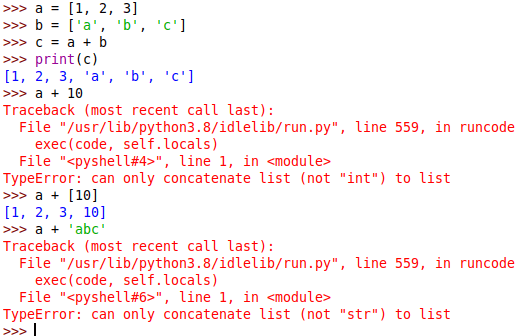
5. Repeat list items using * operator
Since multiplying a list by a number of times and repeating it is the same thing is not exactly adding an item to the list. But we are going to do it anyway because eventually, we are adding something to a list.
The * operator can be used to repeat a list a number of times.
It means when you multiply a list by a number of times, you are repeating the list that many times.
# python repeat a list
a = [1, 2, 3]
print(f"Before repeating: {a}")
a = a * 3
print(f"After repeating: {a}")
Output
Before repeating: [1, 2, 3] After repeating: [1, 2, 3, 1, 2, 3, 1, 2, 3]
Python add to list if not in list
Till now we have been adding anything thing to the list without thinking about whether the item is already in the list or not.
But how will you add something to a list only if it is not in the list?🤔
Simply you can use the 'in' operator to check if the item is already in the list. If it is not in the list, then add it to the list.
# add to list if not in list
a = [1, 2, 3, 4, 5]
b = 6
if b not in a:
a.append(b)
print(a)
b = 10
if b not in a:
a.append(b)
print(a)
b = 4
if b not in a:
a.append(b)
print(a)
Output
[1, 2, 3, 4, 5, 6] [1, 2, 3, 4, 5, 6, 10] [1, 2, 3, 4, 5, 6, 10]
Add List To Another List
Above all discussed methods what do you think, which method is most suitable for adding a list to another list?🤔
Simply the extend() method. Because it extends the list by adding each element of the list to another list as its own element.
The same can also be achieved by using the + operator.
# add list to another list
a = [1, 2, 3]
b = [4, 5, 6]
a.extend(b)
print(a)
Output
[1, 2, 3, 4, 5, 6]
Conclusion
In this awesome guide, we have covered how to add items to a list in Python using various different methods.
Now you know how to add items to a list in Python.
However, the most common way to add items to a list is by using the append() method.
Reference: append method