Check if String Contains Substring from List Python
Checking if a string contains a substring from a list is a common task in Python, often encountered when parsing text or searching for specific patterns.
There are several ways to check if a string contains a substring from a list in Python. In this article, we are going to look at 4 different methods to do so.
- Checking for Substrings in Python
- Speed Comparison
- Conclusion
Table of Contents
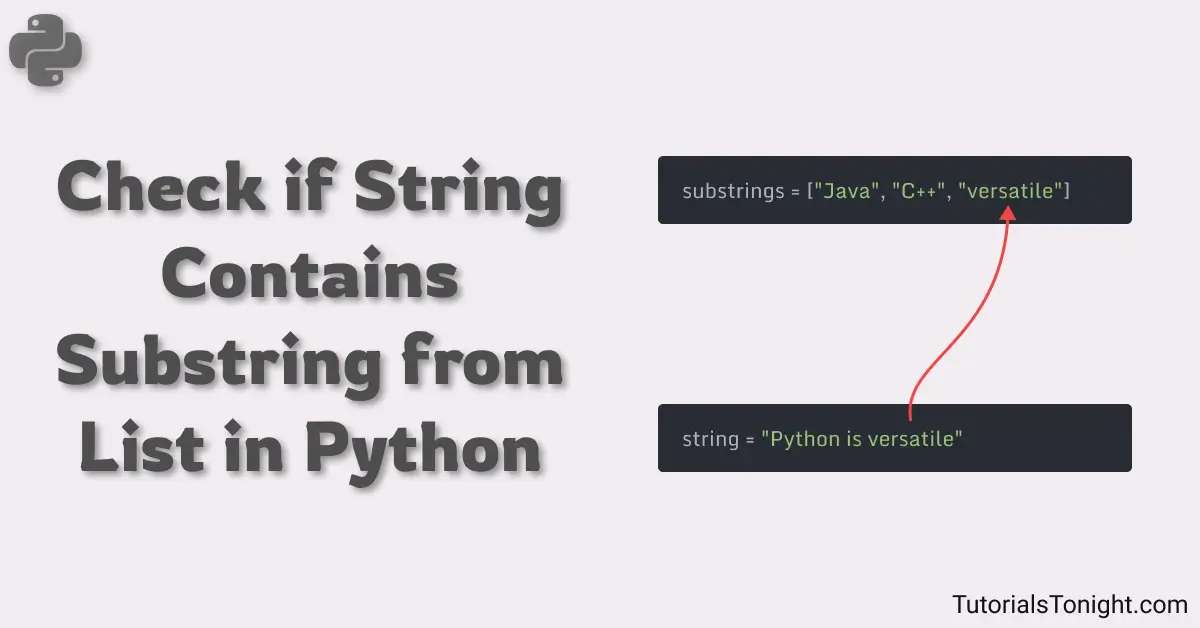
Checking for Substrings in Python
Before diving into specific methods, let's understand the basics of checking for substrings in Python.
The in keyword proves valuable for these scenarios, allowing us to determine if a substring is present in a given string.
You can use the in
keyword to check the presence of a substring in a string, as shown below.
# Basic substring check
string = "Hello, World!"
substring = "Hello"
if substring in string:
print("Substring found!")
1. Using a Loop
We have seen above how to check if a string contains a substring. Now to check for multiple substrings (list of substrings), for this use a loop and iterate through each substring, and check if it is present in the given string.
In the following example we are using for loop and checking for each element of the list and printing it if it is present in the given string.
string = "Python is amazing"
substrings = ["Python", "Java", "C++"]
# Iterate through each substring
for substring in substrings:
# Check if substring is in the main string
if substring in string:
print(f"Found: {substring}")
Output:
Found: Python
2. Using any() Function
The any()
function accepts an iterable and returns True
if any element of the iterable is True
.
Pass sub in the string for sub in substrings
generator expression to the any()
function to check if any substring is present in the main string.
string = "Python is powerful"
substrings = ["Java", "C++", "powerful"]
# Use any() to check if any substring is present
if any(sub in string for sub in substrings):
print("Substring found!")
Output:
Substring found!
3. List Comprehension
The following example uses List comprehension to create a list of substrings present in the main string.
string = "Python is versatile"
substrings = ["Java", "C++", "versatile"]
# Use list comprehension to create a list of found substrings
found_substrings = [
sub for sub in substrings if sub in string
] # found_substrings will contain the substrings present in the main string
print(f"Found: {found_substrings}")
Output:
Found: ['versatile']
4. Using map() Function
The map()
function applies a function to each element of an iterable. Use the lambda function to check if each substring is present in the given string.
Look at the example below to understand it.
string = "Python is dynamic"
substrings = ["Java", "C++", "dynamic"]
# Use map() to apply the 'in' operation and convert to list
found_substrings = list(map(lambda sub: sub in string, substrings))
print(f"Found: {found_substrings}")
# found [False, False, True]
Output:
Found: [False, False, True]
Later you can use [item for item in found_substrings if item]
to get the list of substrings present in the main string.
Speed Comparison
While checking be mindful of case sensitivity; consider converting both the string and substrings to lowercase or uppercase.
For a large list of substrings, the any()
function is the most efficient method, followed by list comprehension.
Here is a speed comparison of all the methods discussed above.
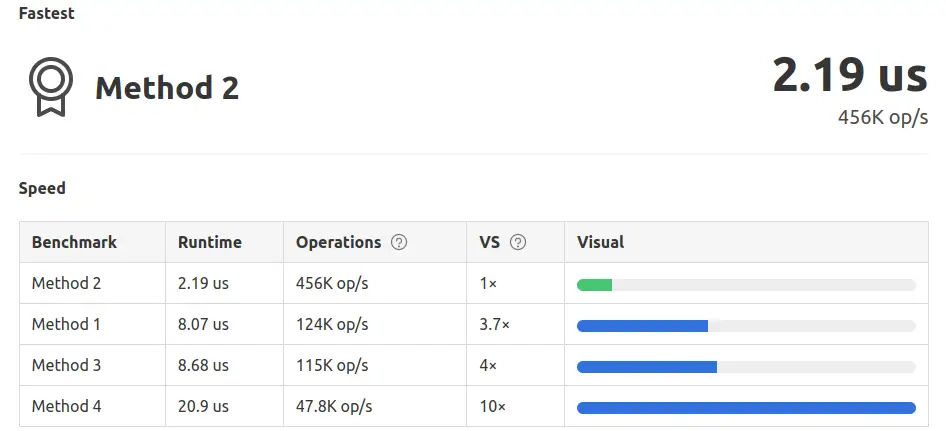
Conclusion
Selecting the right method depends on the specific use case. Whether utilizing a loop, any()
function, list comprehension, or map()
, understanding these approaches equips you to efficiently check if a string contains a substring from a list.
Happy coding! 🚀✨