Nested List Comprehension Python
In this article, we are going to learn about nested list comprehension in python with examples.
List comprehension is a powerful feature of python which is used to create a new list from an existing list, tuple, set, dictionary, etc by modifying elements.
This makes the code more readable and cleaner. This is to be noted that list comprehension is not limited to a single list. It can be used to create a list from multiple lists.
To deal with the lists of lists, we use nested list comprehension.
Nested list comprehension is a list comprehension inside another list comprehension.
The syntax of list comprehension look like this:
new_list = [expression for item in iterable]
You must be familiar with this syntax by now. To create nested list comprehension, add another for loop inside the list comprehension next to the first for loop that uses elements of the first list as the iterable.
new_list = [expression for item1 in iterable1 for item2 in item1]
This is how we can create a nested list comprehension.
For simple understanding look at the image below to understand how nested for loops can be converted into nested list comprehension.
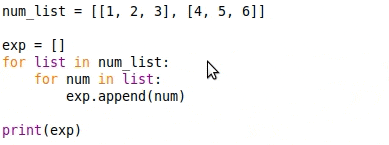
Examples of Nested List Comprehension
With nested list comprehension, we work either on the list or on a list of lists.
Look at the examples below to understand how nested list comprehension works. Thy also shows for loop equivalent of the nested list comprehension.
Example 1: Create a list of lists
The following example create a list of lists of numbers from 1 to 3.
- list comprehension
- for loop
# creating a list of lists
# using nested list comprehension
list_of_lists = [[i for i in range(1,4)] for j in range(3)]
print(list_of_lists)
list_of_lists = []
# nested for loop
for j in range(3):
list_of_lists.append([])
for i in range(1,4):
list_of_lists[j].append(i)
print(list_of_lists)
Output:
[[1, 2, 3], [1, 2, 3], [1, 2, 3]]
[i for i in range(1,4)]
will create a list of numbers from 1 to 3. And further, for j in range(3)
will create the same list 3 times. Thus we get a list of lists.
Example 2: Expand a list of lists
The following example expands a list of lists into a single list.
- list comprehension
- for loop
list_of_lists = [[1,2,3],[4,5,6],[7,8,9]]
# expanding a list of lists
list_of_numbers = [num for lst in list_of_lists for num in lst]
print(list_of_numbers)
list_of_lists = [[1,2,3],[4,5,6],[7,8,9]]
list_of_numbers = []
# nested for loop
for lst in list_of_lists:
for num in lst:
list_of_numbers.append(num)
print(list_of_numbers)
Output:
[1, 2, 3, 4, 5, 6, 7, 8, 9]
for lst in list_of_lists
will iterate over the list of lists. And for num in lst
will iterate over the elements of the list and append them to a new list.
Example 3: Create a list of lists using if condition
The following example creates a list of fruits whose lenght is greater than 5.
- list comprehension
- for loop
# List of fruits
fruits = [['Apple', 'Banana'], ['Cherry', 'Grapes']]
# list of fruits with length
# greater than 5
new_list = [fruit for lst in fruits for fruit in lst if len(fruit) > 5]
print(new_list)
fruits = [['Apple', 'Banana'], ['Cherry', 'Grapes']]
new_list = []
# nested for loop
for lst in fruits:
for fruit in lst:
if len(fruit) > 5:
new_list.append(fruit)
print(new_list)
Output:
['Banana', 'Cherry', 'Grapes']
for lst in fruits
will iterate over the list of lists. And for fruit in lst
will iterate over the elements of the list. And if len(fruit) > 5
will check if the length of the fruit is greater than 5. If yes, then it will append the fruit to a new list.