Add Dictionary to List in Python
A common way for data storage in Python is dictionary and and when you work with real world data, you will often find yourself working with a list of dictionaries. So, it is important to know how to add a dictionary to a list in Python.
In this tutorial, you will learn how to add a dictionary to a list in Python in 4 different ways.
We will also pick the fastest way among them by comparing them.
- Using append() method
- Using insert() method
- Using + operator
- Using extend() method
- Speed Comparison
Table of Contents
1. Using append() method
The append() is a well known method in Python that is used to add an element to the end of a list.
It can also be used to add a dictionary to a list in Python.
my_list = []
my_dict = {'name': 'Alice', 'age': 30}
# add dictionary to list
my_list.append(my_dict)
print(my_list)
Output:
[{'name': 'Alice', 'age': 30}]
As you can see, the dictionary is added to the end of the list.
But, what if you want to add the dictionary at the beginning of the list or at some specific index?
For that, you can use the insert() method.
2. Using insert() method
The insert() method adds an element to the list at the specified index.
It accepts two arguments, first is the index at which you want to add the element and second is the element itself.
my_list = [{'name': 'John', 'age': 25}, {'name': 'Emily', 'age': 28}]
new_dict = {'name': 'Mike', 'age': 35}
# add a dictionary to list at index 1
my_list.insert(1, new_dict)
print(my_list)
Output:
[{'name': 'John', 'age': 25}, {'name': 'Mike', 'age': 35}, {'name': 'Emily', 'age': 28}]
In the output you can see, the dictionary is added at the index 1, i.e., the second position of the list.
3. Using list concatenation
In python you can concatenate two lists using the + operator.
You can use this operator to add a dictionary to a list in Python.
my_list = [{'name': 'Alice', 'age': 30}]
new_dict = {'name': 'Bob', 'age': 32}
# add a dictionary to list using +
my_list = my_list + [new_dict]
print(my_list)
Output:
[{'name': 'Alice', 'age': 30}, {'name': 'Bob', 'age': 32}]
4. Using extend() method
The extend() is another method that can be used to add a dictionary to a list in Python.
It accepts an iterable as an argument and adds all the elements of the iterable to the list.
To add a dictionary to a list, you need to pass a list containing the dictionary as an argument and all the dictionaries in that list will be added to calling list.
my_list = [{'name': 'Alice', 'age': 30}]
dicts = [{'name': 'Bob', 'age': 32}, {'name': 'Charlie', 'age': 34}]
# add a dictionary to list using extend()
my_list.extend(dicts)
print(my_list)
Output:
[{'name': 'Alice', 'age': 30}, {'name': 'Bob', 'age': 32}, {'name': 'Charlie', 'age': 34}]
Speed Comparison
Now, let's compare the speed of all the above methods to add a dictionary to a list. For this we will keep the dictionary same for all the methods and will add it to a list 100000 times.
To measure the time taken by each method, we will use the time module of Python.
import time
my_dict = {'name': 'Alice', 'age': 30}
# 1. using append()
my_list = []
start = time.time()
for i in range(100000):
my_list.append(my_dict)
end = time.time()
print("Time taken by append():", end - start)
# 2. using insert()
my_list = []
start = time.time()
for i in range(100000):
my_list.insert(0, my_dict)
end = time.time()
print("Time taken by insert():", end - start)
# 3. using + operator
my_list = []
start = time.time()
for i in range(100000):
my_list = my_list + [my_dict]
end = time.time()
print("Time taken by + operator:", end - start)
# 4. using extend()
my_list = []
start = time.time()
for i in range(100000):
my_list.extend([my_dict])
end = time.time()
print("Time taken by extend():", end - start)
Output:
Time taken by append(): 0.018741607666015625 Time taken by insert(): 4.275395393371582 Time taken by + operator: 36.189534425735474 Time taken by extend(): 0.025027751922607422
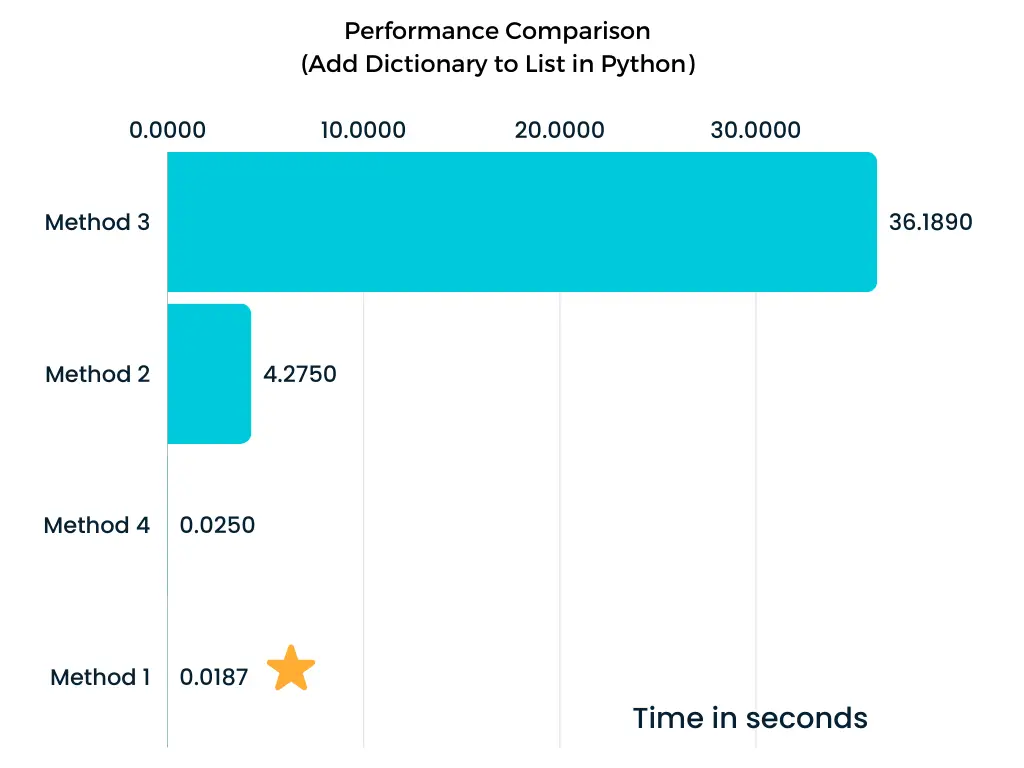
From the above output you can see that the Method 1 is fastest among all the methods, whereas the Method 3 is the slowest.
So, you should use the append() method to add a dictionary to a list in Python.