2D Array/List in Python
2D Array/List is an array of arrays means that each element of an array is a one dimensional array.
It is also called Matrix which can be represented as a collection of rows and columns.
For example, a 2D array in Python looks like this.
# 2D array in python
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
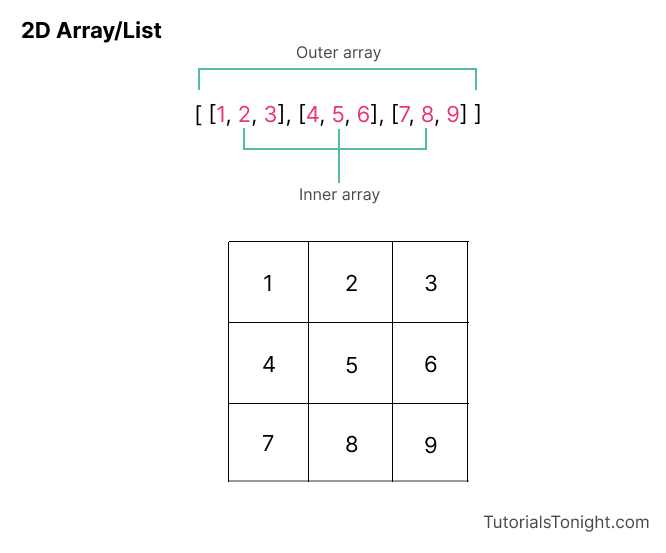
How to create a 2D array in Python?
There can be various ways to create a 2D array in python. Here we will discuss some of the most commonly used ways.
1. Naive Method
In this method, you can create a 2D array using the *
operator. When you multiply a list with a number, it creates multiple copies of that list.
# creating 2D array
matrix = [[0] * 3] * 2
print(matrix)
[[0, 0, 0], [0, 0, 0]]
Here, we have created a 2D array of size 2 x 3
with all elements initialized to 0
.
2. Using List Comprehension
Another way to create a 2D array is by using list comprehension inside another list comprehension.
# creating 2D array
matrix = [[0 for i in range(3)] for j in range(2)]
print(matrix)
[[0, 0, 0], [0, 0, 0]]
3. Using Nested Loop
Same as above, you can also create a 2D array using nested for loops.
# creating 2D array
matrix = []
for i in range(2):
row = []
for j in range(3):
row.append(0)
matrix.append(row)
print(matrix)
[[0, 0, 0], [0, 0, 0]]
Accessing elements of 2D array
Elements of the 2D array are accessed by 2 indices. The first index is used to access the row and the second index is used to access the column.
For example, to access element 6 in the above matrix, you can use matrix[1][2]
. Here, 1
is the index of row and 2
is the index of column.
The following image will help you understand the concept better.
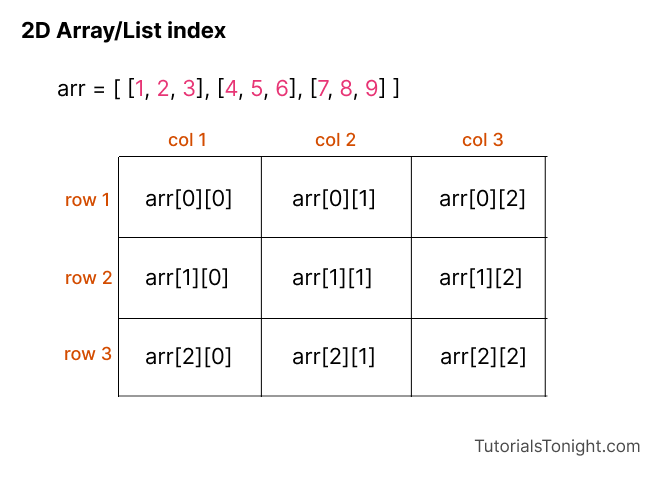
Here is an example that shows how to access elements of the 2D array.
# accessing elements of 2D array
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# accessing element at row 2 column 3
print(matrix[1][2])
# accessing element at row 1 column 2
print(matrix[0][1])
6 2
Traversing a 2D array
Traversing a 2D array means accessing each element of the 2D array once. This can be done using nested for loops.
# traversing a 2D array
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in matrix:
for element in row:
print(element, end=" ")
print()
1 2 3 4 5 6 7 8 9
Adding elements to a 2D array
Adding elements to a 2D array is similar to adding elements to a one dimensional array. You can use the append()
method to add elements to a 2D array.
For this choose the row in which you want to add the element and then use the append()
method to add the element to that row.
# adding elements to a 2D array
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# adding element 10 to row 2 column 3
matrix[1].append(10)
print(matrix)
# adding element 11 to row 4 column 1
matrix.append([11])
print(matrix)
[[1, 2, 3], [4, 5, 6, 10], [7, 8, 9]] [[1, 2, 3], [4, 5, 6, 10], [7, 8, 9], [11]]
Deleting elements from a 2D array
To delete you can use the del
keyword followed by the name of the array and the index of the element to be deleted.
For example, to delete element 6 from the above matrix, you can use del matrix[1][2]
.
# deleting elements from a 2D array
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# deleting element at row 2 column 3
del matrix[1][2]
print(matrix)
# deleting element at row 1 column 2
del matrix[0][1]
print(matrix)
[[1, 2, 3], [4, 5], [7, 8, 9]] [[1, 3], [4, 5], [7, 8, 9]]
Updating elements of a 2D array
Same as accessing elements, you can update elements of a 2D array using indices.
You can assign a new value to the element at a particular index to update it.
# updating elements of a 2D array
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# updating element at row 2 column 3
matrix[1][2] = 10
print(matrix)
# updating element at row 1 column 2
matrix[0][1] = 11
print(matrix)
[[1, 2, 3], [4, 5, 10], [7, 8, 9]] [[1, 11, 3], [4, 5, 10], [7, 8, 9]]
2D array operations
Let's look at some of the operations that can be performed on a 2D array. Going through various operations will boost your understanding of 2D arrays.
1. Transpose of a 2D array
Transpose of a matrix is a matrix that is formed by turning all the rows of a given matrix into columns and vice-versa.
For example, the transpose of the above will be [[1, 4, 7], [2, 5, 8], [3, 6, 9]]
.
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# transpose of a matrix
transpose = [[0, 0, 0], [0, 0, 0], [0, 0, 0]]
for i in range(len(matrix)):
for j in range(len(matrix[0])):
transpose[j][i] = matrix[i][j]
print(transpose)
[[1, 4, 7], [2, 5, 8], [3, 6, 9]]
To create a transpose matrix we assigned the value of matrix[i][j]
to transpose[j][i]
. This is because the rows of the matrix become columns of transpose and columns of the matrix become rows of transpose.
2. Addition of two matrices
Two matrices can be added only if they have the same dimensions. The sum of two matrices A
and B
will be a matrix that has the same dimensions as A
and B
, that is m x n
.
The sum of two matrices A
and B
will be A + B
and it will be equal to B + A
.
For example, the sum of the above two matrices will be [[2, 4, 6], [8, 10, 12], [14, 16, 18]]
.
matrix1 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
matrix2 = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
# addition of two matrices
sum = [[0, 0, 0], [0, 0, 0], [0, 0, 0]]
for i in range(len(matrix1)):
for j in range(len(matrix1[0])):
sum[i][j] = matrix1[i][j] + matrix2[i][j]
print(sum)
[[2, 4, 6], [8, 10, 12], [14, 16, 18]]
Conclusion
So this was all about 2D array in python. By now you must have understood what is 2D array and how to create, access, update and delete elements of a 2D array.
Also, we have seen some of the operations that can be performed on 2D array.
To boost your understanding of 2D array, try to solve the following questions.
- Write a program to find the maximum element of a matrix.
- Write a program to find the diagonal sum of a matrix.