Python Merge Two List
In this article, you will how Python merge two lists using multiple different methods with examples.
List in Python stores the bucket of data. While working with lists, you may get 2 or more than 2 lists that hold similar data. In this situation, you may need to merge the data into a single list.
# Quick Solution
For a quick solution, you can use the + operator to merge the lists.
list1 = [1, 2, 3]
list2 = [4, 5, 6]
# merging two lists
list3 = list1 + list2
print(list3)
Output:
[1, 2, 3, 4, 5, 6]
Let's now see how to merge two lists in Python using different methods and understand the differences between them.
- Using + operator
- Using extend() Method
- By Unpacking Generalizations
- Using list comprehension
- Using itertools.chain() Method
- Using for loop
Table Of Contents
1. Merge List Using + Operator
The + operator can concatenate two or more than two lists.
The lists are connected end to end as a single list in the same order.
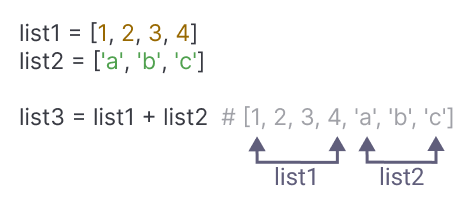
You can see in the above image the lists are in the same order and the items in the list are also in the same order.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
# merge two lists using + operator
list3 = list1 + list2
print(list3)
Output:
[1, 2, 3, 'a', 'b', 'c']
2. Merge List Using extend() Method
The extend() method extends the elements of a list with the elements of an iterable (list, tuple, string, etc).
You can use it to merge two lists. The elements of the first list will be extended with the elements of the second list.
All the elements of the second list will be appended to the end of the first list.
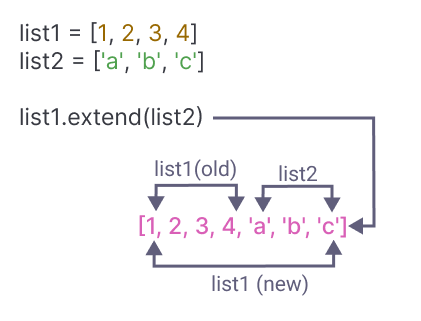
Let's see an example of merging two lists using the extend() method.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
# merge two lists using extend() method
list1.extend(list2)
print(list1)
Output:
[1, 2, 3, 'a', 'b', 'c']
3. Merge lists By Unpacking Generalizations [*l1, *l2]
The unpacking generalizations in PEP 448 is an added support in Python 3.5 and above.
It allows you to unpack a sequence into a sequence of variables.
Using this you can unpack lists within square brackets and it will automatically merge the lists.
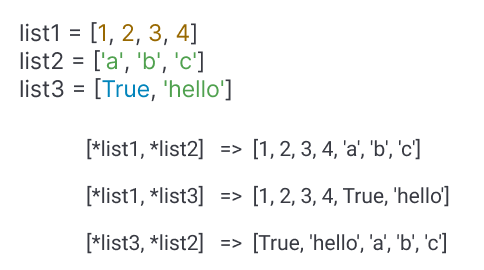
Look at the above image to understand how unpacking generalizations work for merging two lists.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
list3 = [True, 'hello', 3.14]
# using unpacking generalizations
list4 = [*list1, *list2]
print(list4) # [1, 2, 3, 'a', 'b', 'c']
list5 = [*list1, *list3]
print(list5) # [1, 2, 3, True, 'hello', 3.14]
list6 = [*list3, *list2]
print(list6) # [True, 'hello', 3.14, 'a', 'b', 'c']
Output:
[1, 2, 3, 'a', 'b', 'c'] [1, 2, 3, True, 'hello', 3.14] [True, 'hello', 3.14, 'a', 'b', 'c']
4. Merge lists Using list comprehension
In Python list comprehension is a powerful way to create a list from an existing list.
You can use logic to merge two lists and create a new list.
Let's see an example of merging two lists using list comprehension.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
# merge two lists using list comprehension
list3 = [item for lists in [list1, list2] for item in lists]
print(list3)
Output:
[1, 2, 3, 'a', 'b', 'c']
5. Merge lists Using itertools.chain()
The itertools module provides a chain() function that takes a variable number of iterables and returns a single iterable that contains all the elements of the input iterables.
You can use this function to merge two lists.
The return type of the function is an iterator. So to convert it into a list you can use the list() function.
Let's see an example of merging two lists using the itertools.chain() function.
from itertools import chain
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
# using chain() function
list3 = list(chain(list1, list2))
print(list3)
Output:
[1, 2, 3, 'a', 'b', 'c']
6. Merge lists Using for loop
Tell me with your programming experience, how you can merge two lists using a for loop?💭🤔
Let's see how you can merge two lists using a for loop.
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
# merge list2 into list1
for i in list2:
list1.append(i)
print(list1)
Output:
[1, 2, 3, 'a', 'b', 'c']
Here is a task for you to merge list1 into list2 using a for loop.
Conclusion
That's all with Python Merge Two Lists.🤗
You have learned 5 ways to merge two lists in Python. Now you can choose any one of them depending on your preference and practice.