Replace Item in List Python
Replacing items in a list is a common task while manipulating lists in Python. You may need to update specific elements or modify multiple occurrences of a value.
In this article, we will explore different methods to replace items in a list in Python.
- Using index Assignment
- Using List Comprehension
- Using enumerate() function
- Using map() function
- Using numpy.where() function
- Speed Comparison
Table of Contents
1. Using index Assignment
Most of the time, we know the index of the item we want to replace. In that case, we can simply assign the new value to the index of the item we want to replace.
Let's see an example.
fruits = ['apple', 'banana', 'cherry', 'orange', 'kiwi', 'mango']
# replace banana with grapes
fruits[2] = 'grapes'
print(fruits)
Output:
['apple', 'banana', 'grapes', 'orange', 'kiwi', 'mango']
As you can see, we have replaced the item at index 2 with the new value.
2. Using List Comprehension
Another way to replace items in a list is by using list comprehension.
Here is an example.
num = [1, 2, 3, 4, 5]
# replace all even numbers with 0
num = [0 if i%2==0 else i for i in num]
print(num)
Output:
[1, 0, 3, 0, 5]
You can replace multiple items in a list at once using list comprehension.
Visit to understand if-else in list comprehension in detail.
3. Using enumerate() function
The enumerate() function returns an object containing the index and value of each item in the list as a tuple.
You can loop through this object and replace the items you want.
num = [1, 2, 3, 4, 5]
# Replacing items using enumerate()
for index, value in enumerate(num):
if value == 3:
num[index] = 'new_item'
print(num)
# Output: [1, 2, 'new_item', 4, 5]
Above we accessed the value of the element and as we found a match we replaced it with the new value. With the help of the enumerate() function, you can also replace items based on their index.
num = [1, 2, 3, 4, 5]
# Replacing items using enumerate()
for index, value in enumerate(num):
if index == 3:
num[index] = 'new_item'
print(num)
# Output: [1, 2, 3, 'new_item', 5]
4. Using map() function
Another approach to replacing items in a list is by using the map() function along with lambda functions.
The map() function takes a function as an argument and applies it to each item in the list.
my_list = [1, 2, 3, 4, 5]
old_value = 2
new_value = 10
# Replacing items using map() function
my_list = list(map(lambda x: new_value if x == old_value else x, my_list))
print(my_list)
# Output: [1, 10, 3, 4, 5]
In the above example the lambda function checks if the element matches the old value and replaces it with the new value accordingly.
5. Using numpy.where() function
Let's use the numpy library to replace items in a list.
To replace an item in a list using numpy, you can use the numpy.where() function.
Here is how you can do it.
import numpy as np
my_list = [1, 2, 3, 4, 5]
old_value = 2
new_value = 10
# Replacing items using numpy.where() function
my_list = np.where(np.array(my_list) == old_value, new_value, my_list)
print(my_list)
# Output: [1, 10, 3, 4, 5]
Speed Comparison
By leveraging the above techniques such as list indexing and assignment, list comprehensions, or the map() function, you can easily modify the contents of a list to meet your requirements.
However, if you are working with large lists, you may want to consider the speed of each method.
We ran each method 1000 times on a list of 5 elements and plotted a graph to compare the speed of each method.
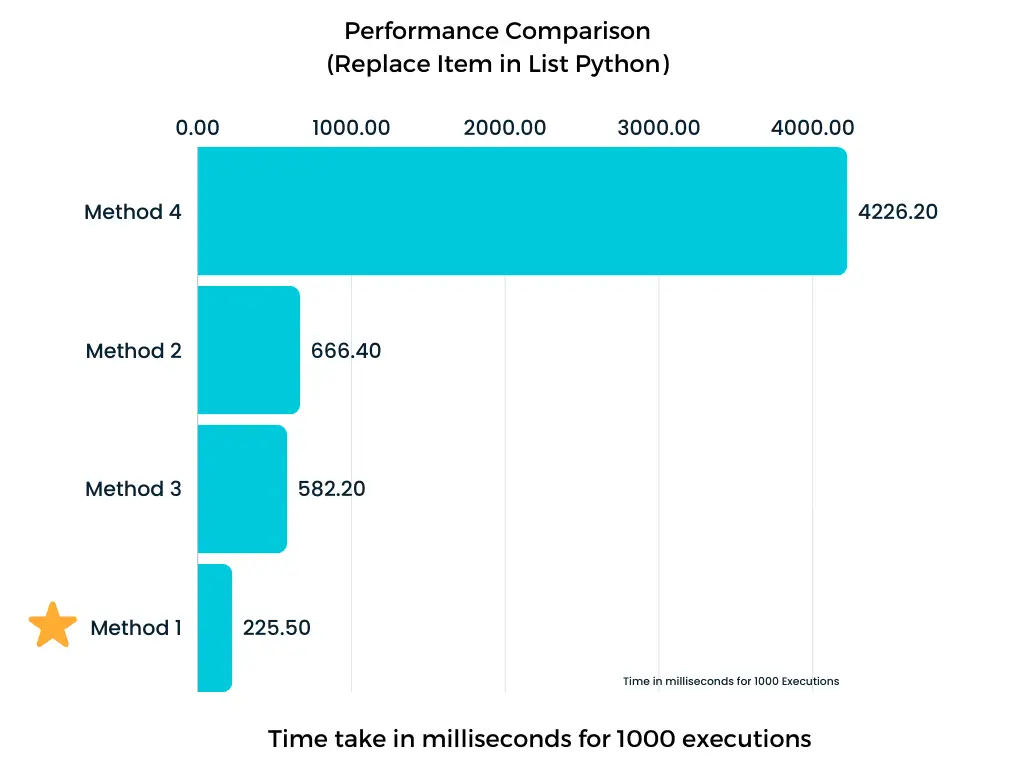
As you can see, the index assignment method is the fastest, followed by the list comprehension method.