Dictionary Comprehension in Python
In this tutorial, you are going to learn about Dictionary Comprehension in Python with various examples.
- Dictionary Comprehension
- Dictionary Comprehension with if condition
- Nested Dictionary Comprehension
- Conclusion
Table Of Contents
Dictionary Comprehension
Previously, you have learned about List Comprehension in Python. Just like list comprehension is a concise way to create a list, dictionary comprehension is a concise way to create a dictionary.
Dictionary comprehension is a way to create a dictionary in a single line. It is a combination of for loop and if-else statements.
Using this you can create a dictionary from another dictionary, list, tuple, set, etc.
Syntax
Let's see the syntax of dictionary comprehension.
{key:value for (key, value) in iterable}
# or
{key:value for (key, value) in iterable (if condition)}
Here, an iterable can be a dictionary, list, tuple, set, string, etc.
The first part key:value is the output expression producing key-value pairs. So it can be any expression involving key and value.
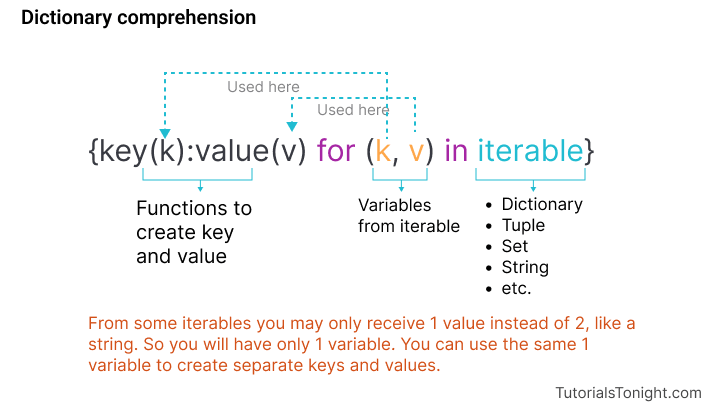
Let's see an example to understand it better with the help of examples.
Example 1: Create a dictionary from a list
Let's combine a list of fruits and their prices to create a dictionary.
fruits = ["apple", "banana", "cherry"]
prices = [100, 50, 150]
# create a dictionary using dictionary comprehension
fruits_price = {fruit:price for (fruit, price) in zip(fruits, prices)}
print(fruits_price)
{'apple': 100, 'banana': 50, 'cherry': 150}
Here, we have created a dictionary using dictionary comprehension. The output expression is fruit:price. The iterable is zip(fruits, prices). zip() is a built-in function that takes two or more iterables and returns an iterator of tuples.
Example 2: Create a dictionary from a string
Let's create a dictionary from a string. The example is to count the number of times each character appears in a string.
string = "banana"
# creating a dictionary that
# contains the count of each character
char_count = {char:string.count(char) for char in string}
print(char_count)
{'b': 1, 'a': 3, 'n': 2}
Here, you can see we are using the expression char:string.count(char) in the output expression.
Example 3: Create a dictionary from a dictionary
Let's create a dictionary from another dictionary.
The example includes a dictionary of fruits and their prices. We will create a new dictionary that contains the fruits and with 30% discount.
fruits_price = {"apple": 100, "banana": 50, "cherry": 150}
# create a new dictionary with 30% discount
discount = {fruit:price*0.7 for (fruit, price) in fruits_price.items()}
print(discount)
{'apple': 70.0, 'banana': 35.0, 'cherry': 105.0}
Here, we have used the items() method to get the key-value pairs from the dictionary.
Dictionary Comprehension with if condition
You can also use conditional statements in dictionary comprehension.
Let's see an example to understand it better.
Example 4: Create a dictionary from a list with if condition
Let's create a dictionary from a list with if condition.
The example includes a list of fruits and their prices. We will create a new dictionary that contains the fruits and their prices only if the price is greater than 100.
fruits = ["apple", "banana", "cherry"]
prices = [105, 50, 150]
# create a dictionary that contains
# fruits and their prices only if the price is greater than 100
fruits_price = {fruit:price for (fruit, price) in zip(fruits, prices) if price > 100}
print(fruits_price)
{'apple': 105, 'cherry': 150}
Here, we have used if price > 100 to filter the fruits and their prices.
Example 5: using if and else
Let's create a dictionary from a list of natural numbers. If the number is even, then we will use the number as the key and the square of the number as the value. If the number is odd, then we will use the number as the key and the cube of the number as the value.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9]
# create a dictionary that contains
# square of even numbers and cube of odd numbers
numbers_dict = {number:number**2 if number%2==0 else number**3 for number in numbers}
print(numbers_dict)
{1: 1, 2: 4, 3: 27, 4: 16, 5: 125, 6: 36, 7: 343, 8: 64, 9: 729}
Here, we have used number**2 if number%2==0 else number**3 in the output expression.
Nested Dictionary Comprehension
You can also use nested dictionary comprehension. This means you can use dictionary comprehension inside another dictionary comprehension.
Note: Nested dictionary comprehension sometimes makes the code difficult to understand. So, you should use it only when it is necessary.
Example 6: Nested dictionary comprehension
Let's create a nested dictionary from a string. The example contains a list of strings. We will create a dictionary that contains the count of each character in each string.
strings = ["apple", "banana", "cherry"]
# create a dictionary that contains
# count of each character in each string
char_count = {string:{char:string.count(char) for char in string} for string in strings}
print(char_count)
{'apple': {'a': 1, 'p': 2, 'l': 1, 'e': 1}, 'banana': {'b': 1, 'a': 3, 'n': 2}, 'cherry': {'c': 1, 'h': 1, 'e': 1, 'r': 2, 'y': 1}}
Here, we have used dictionary comprehension inside another dictionary comprehension.
Dictionary Comprehension with Functions
In the image of the syntax section above you can see that we can use the function in the output expression.
You can replace the output expression with a function that takes items of the iterable as arguments and returns the value for the key or value of the dictionary.
Let's look at some examples.
Example 7: Dictionary comprehension with function
In the following function, we iterate through the list of numbers and mark the numbers as prime or not prime.
from math import sqrt
def is_prime(number):
if number == 1:
return "Not prime"
for i in range(2, int(sqrt(number))+1):
if number % i == 0:
return "Not prime"
return "Prime"
numbers = [21, 43, 53, 87, 99, 101]
# create a dictionary that contains
# number and its prime status
prime_status = {number:is_prime(number) for number in numbers}
print(prime_status)
{21: 'Not prime', 43: 'Prime', 53: 'Prime', 87: 'Not prime', 99: 'Not prime', 101: 'Prime'}
Here, we have used is_prime(number) as the output expression.
Conclusion
Dictionary comprehension is a tool that you can use to create dictionaries in a single line. It is very useful when you want to create a dictionary from another iterable.
Some of the key points of dictionary comprehension are:
- It is a single-line expression.
- It is used to create dictionaries from other iterables.
- It is faster than for loop.
- It can be nested.
- It can be used with functions.