Python While Loop with Examples
In this tutorial, we are going to extend our knowledge of Python by learning about the while
loop. It is another loop that python has apart from for
loop. Let's look at it in detail with various examples.
- While Loop Introduction
- Break While Loop
- Continue While Loop
- Else in While Loop
- Nested While Loop
- When to Use While Loop
- Conclusion
Table Of Contents
Introduction To Python While Loop
The while loop is another loop that python has apart from for loop.
The while loop is used to execute a block of code again and again until the condition is false.
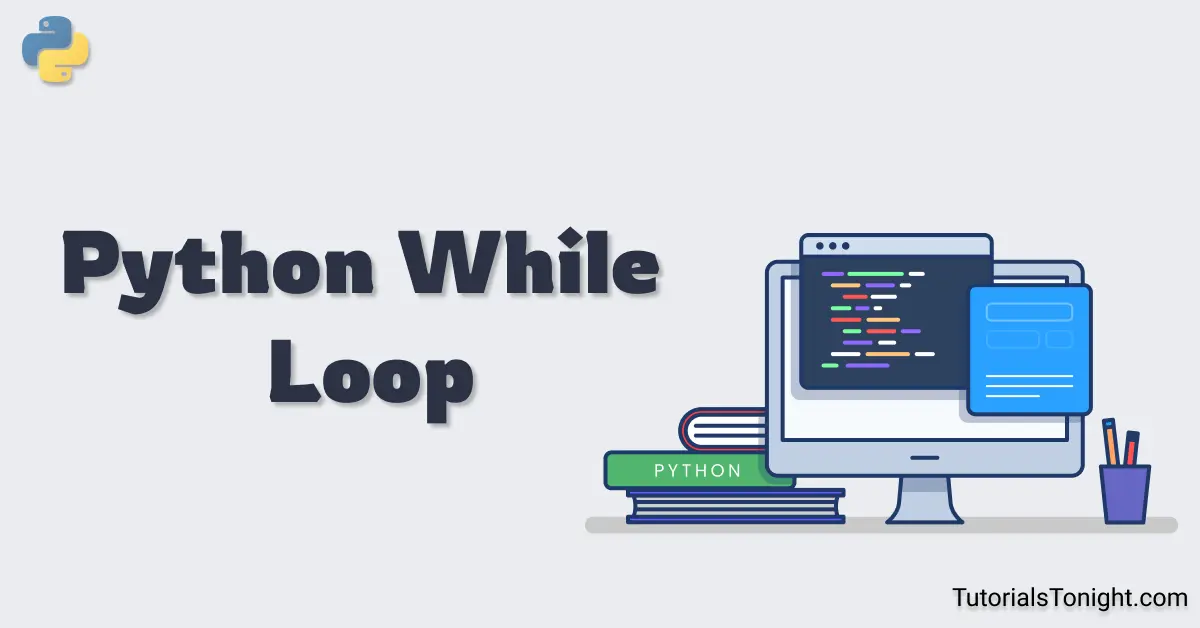
The while loop is very useful when you want to repeatedly execute a block of code until a certain condition is met. The condition may be that the sum becomes greater than 100, the string becomes empty, prime value is found, etc.
Syntax
The syntax of python while loop is as follows:
while condition:
# block of code
The condition is checked at the start of each iteration. If the condition is true, the block of code is executed. If the condition is false, the loop is terminated.
The condition can be any expression that evaluates to True or False.
Flowchart
The flowchart below shows the while loop in action.
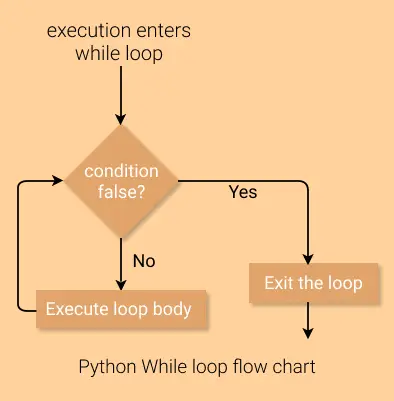
Note: The most important part of the while loop is the increment, decrement, or change of some variable within the block of code so that loop moves toward the finish line. Otherwise, the loop will never terminate.
While Loop Example
Example 1: Print the numbers from 1 to 10.
i = 1
while i <= 10:
print(i, end=" ")
# important: increment i
i += 1
Output:
1 2 3 4 5 6 7 8 9 10
Example 2: Calculate the sum of natural numbers until the sum becomes greater than 100.
sum = 0
i = 1
while sum <= 100:
sum += i
i += 1
print(f"Loop executed {i} times and the sum is {sum}")
Output:
Loop executed 15 times and the sum is 105
Example 3: Execute loop until list becomes empty.
list = [1, 2, 3, 4, 5]
while list:
print(list.pop())
Output:
5 4 3 2 1
Break While Loop
The break statement is used to terminate the loop. You can use it with an if-else statement to terminate the loop when the condition is satisfied.
Using the break statement you can start an infinite loop and terminate it on your desired condition.
while True: # infinite loop
print("Hello World!")
break # terminate the loop
Output:
Hello World!
The above loop is an infinite loop but as soon as the break statement is executed, the loop is terminated.
Let's see another example of the break statement.
i = 1
sum = 0
while i <= 10:
print(i, end=" ")
sum += i
# break loop when the sum is greater than 25
if sum > 25:
break
i += 1
Output:
1 2 3 4 5 6 7
The above loop is terminated when the sum becomes greater than 25.
Continue While Loop
The continue statement is used to skip the current iteration of the loop. This means while the loop is executing, it will skip the current iteration if the program says continue for a certain condition.
This is not commonly used, but you can use this for the values for which you don't want your loop to execute.
The example below skips the current iteration if the value is even.
i = 1
while i <= 10:
# skip the current iteration if i is even
if i % 2 == 0:
continue
print(i, end=" ")
i += 1
Output:
1 3 5 7 9
Else in While Loop
Python provides an else statement to execute with the while loop.
The else statement is executed once the condition of the while loop is no longer true. This is useful when you want to execute a block of code when the condition becomes false.
Let's see an example of an else statement in the while loop.
i = 1
while i <= 5:
print(i, end=" ")
i += 1
else:
print("i is greater than 5")
Output:
1 2 3 4 5 i is greater than 5
The above example prints the numbers from 1 to 5 and then prints the message "i is greater than 5" when the condition of loop becomes false.
Nested While Loop
You can use a while loop inside another while loop. This is called a nested loop.
A nested loop is used when a problem is unable to be solved by a single loop. For example, sorting a list of numbers using a while loop.
Let's see an example of a nested loop.
# print pattern of numbers 1 to 5
i = 1
n = 5
while i <= n:
j = 1
while j <= i:
print(j, end=" ")
j += 1
print()
i += 1
Output:
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5
When to use While Loop
You can use while loop in the following situations:
- When you don't know the number of iterations of the loop. Use a while loop, when the number of iterations is either unknown or, has nothing to do with the condition of the loop.
- When you want to execute a block infinitely and terminate it on your desired condition.
Conclusion
This amazing journey taught you everything about python while loop. You learned how to use the while loop, when to use it, its key elements, etc with various examples.
Now use this concept and solve some awesome problems to boost learning.