Python String to Binary
You must be knowing that computers only understand binary numbers (0 and 1). So, whenever you store a string in a computer, it actually stores the binary code of that string.
In this tutorial, you will learn how to convert a string to binary in Python in 3 different ways.
At the end you will also see a speed comparison between these 2 methods to find out which one is the fastest.
1. Using bin() function
The bin() function converts a given integer to its binary equivalent. For example, bin(10)
will return '0b1010'
.
So, to convert a string to binary, we need to convert each character to its integer equivalent and then convert that integer to binary.
To get the integer equivalent of a character, we can use the ord() function.
Here is a program to convert a string to binary.
str = "Hello"
# loop over each character in string
# and convert them to binary
for char in str:
# convert character to integer
ascii_val = ord(char)
# convert integer to binary
binary_val = bin(ascii_val)
# print the binary value
print(binary_val, end=" ")
Output:
0b1001000 0b1100101 0b1101100 0b1101100 0b1101111
Note: The bin() function returns a string with '0b'
as prefix. So, we have to remove this prefix from the binary value.
2. Using format() function
Issue with the above method is that it returns a string with '0b'
as prefix. You have to manually remove this prefix from the binary value.
However, the format() function can be used to convert an integer to binary without the prefix.
Here is a program to convert a string to binary using format() function.
str = "Hello"
binary_str = ""
# loop over each character in string
# and convert them to binary
for char in str:
# convert character to integer
ascii_val = ord(char)
# convert integer to binary
binary_val = format(ascii_val, "b")
# append the binary value
binary_str += binary_val + " "
print(binary_str)
Output:
1001000 1100101 1101100 1101100 1101111
Here, we have used "b"
as the second argument to the format() function. This tells the function to convert the integer to binary. To get consistent 8-bit binary values, you can use "08b"
as the second argument.
Conclusions
Both the methods discussed above are easy to understand and implement. You can choose any of them to convert a string to binary.
The following image show the speed comparison between these 2 methods.
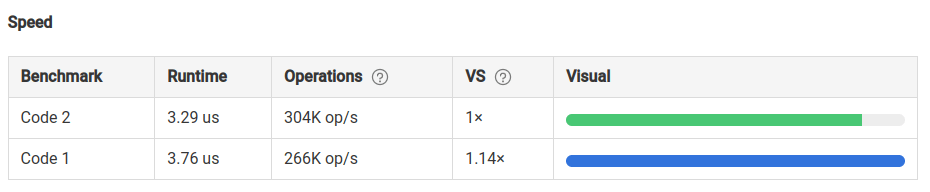
You can see that the format() function is faster than the bin() function.