Python Binary to String Conversion
Computers understand only binary numbers. All the data that we store in a computer is in binary format. Whether it is a text file, image, video, or any other file, it is stored in binary format.
Sometimes we need to convert binary data into a string. For example, network communication, reading a binary file, cryptography, etc.
Here you will learn 3 different ways to convert binary data into a string in Python.
Later in this tutorial, we will also compare the speed of all the methods to find the fastest one.
1. Using int() and chr() function
Most straightforward way to convert binary data into a string is to use int() and chr() function.
First, you can convert binary data into an integer using int() function and then this integer can be treated as an ASCII value of a character and can be converted into a string using chr() function.
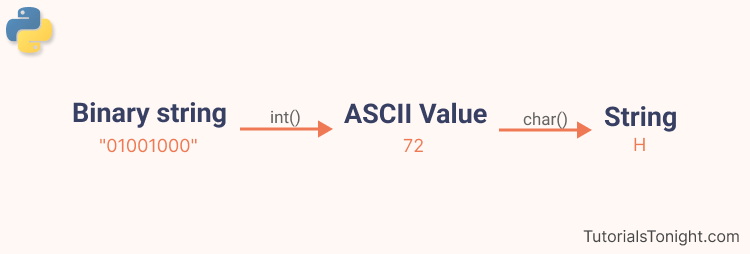
Here is a program that does the same.
# convert binary data into a string
binary_string = "01001000 01100101 01101100 01101100 01101111"
# split string into a list of binary values
binary_values = binary_string.split()
ascii_string = ""
# loop over binary values
for binary_value in binary_values:
# convert binary value to an integer
an_integer = int(binary_value, 2)
# convert integer to an ascii character
ascii_character = chr(an_integer)
ascii_string += ascii_character
print(ascii_string)
Output:
Hello
2. Using int() and bytes() function
Another way to convert binary data into a string is to use int() and bytes() function.
The bytes() function takes an array of integers as an argument and returns a byte object. This byte object can be converted into a string using decode() function.
# convert binary data into a string
binary_string = "01001000 01100101 01101100 01101100 01101111"
# split string into a list of binary values
binary_values = binary_string.split()
str = ""
# loop over binary values
for binary_value in binary_values:
# convert binary value to an integer
an_integer = int(binary_value, 2)
# convert integer to a byte
a_byte = bytes([an_integer])
# decode byte to string
ascii_character = a_byte.decode()
str += ascii_character
print(str)
Output:
Hello
3. Using binascii module
Python provides a module named binascii. It contains various functions to convert binary data into different formats.
One of such functions is unhexlify() function. It takes a string of hexadecimal values as an argument and returns a byte object.
Once you have a byte object, you can convert it into a string using decode() function.
import binascii
# convert binary data into a string
binary_string = "01001000 01100101 01101100 01101100 01101111"
# split string into a list of binary values
binary_values = binary_string.split()
str = ""
# loop over binary values
for binary_value in binary_values:
# convert binary value to a byte
a_byte = binascii.unhexlify('%x' % int(binary_value, 2))
# decode byte to string
ascii_character = a_byte.decode()
str += ascii_character
print(str)
Output:
Hello
Speed Comparison
You have seen 3 different ways to convert binary data into a string. Now let's compare the speed of all the methods to find the fastest one.
We run each method and find following results.
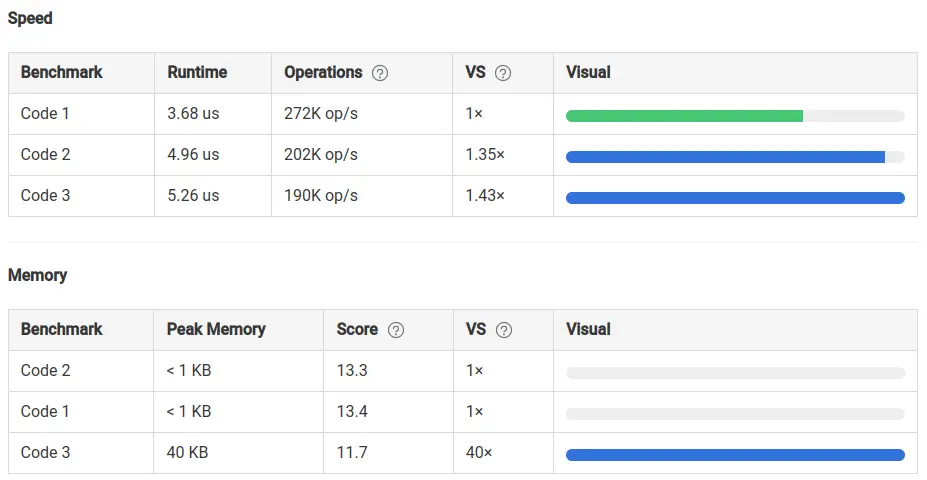
As you can see, the first method is the fastest one and the third method is the slowest one.