Diamond Star Pattern In Java
In this tutorial, we will learn to create Diamond Star Pattern In Java. We will see 6 different patterns with stars, numbers, and alphabets with a full explanation.
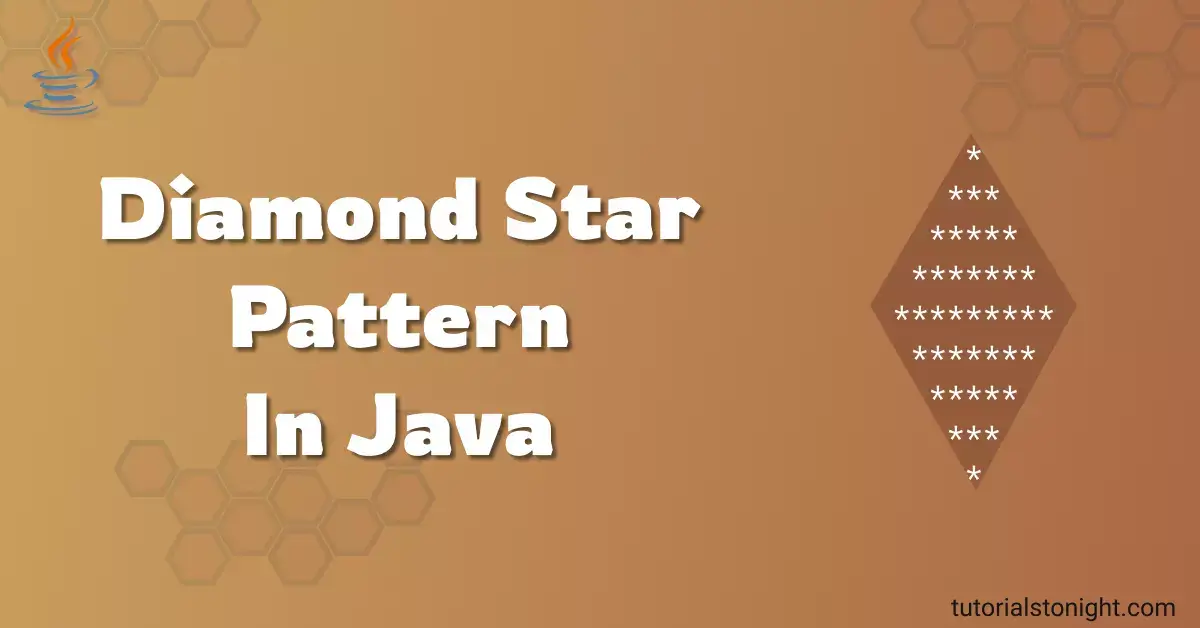
- Diamond star pattern in Java
- Hollow diamond star pattern in Java
- Number diamond pattern
- Hollow number diamond pattern
- Alphabet diamond pattern
- Hollow alphabet diamond pattern
Table Of Contents
1. Diamond star pattern in Java
The diamond star pattern is quite a famous pattern program that is asked in exams and interviews.
* *** ***** ******* ********* ******* ***** *** *
The above pattern is the diamond star pattern. If you look closely, you will notice that the pattern is symmetrical and is made up of two parts: Pyramid and reverse pyramid.
Here are the steps to create the diamond star pattern in Java:
- Take the size of the pattern. In this case, the size is 5.
- Generate the diamond pattern in 2 steps, first create an upper pyramid and then create a lower pyramid.
- To create an upper part, print spaces before printing the star in the upside pyramid then print the star such that it makes the shape of a pyramid.
- Create another loop to print the downside pyramid.
- Start with 1 and end with size-1. Again do the same thing but in the reverse order.
Complete Code:
class starDiamond {
public static void main(String[] args) {
// set a size
int size = 5;
// upper pyramid
for (int i = 1; i <= size; i++) {
// print spaces
for (int j = size; j > i; j--) {
System.out.print(" ");
}
// print star
for (int k = 0; k < i * 2 - 1; k++) {
System.out.print("*");
}
System.out.println();
}
// lower pyramid
for (int i = 1; i <= size - 1; i++) {
// print spaces
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// print star
for (int k = (size - i) * 2 - 1; k > 0; k--) {
System.out.print("*");
}
System.out.println();
}
}
}
Output:
* *** ***** ******* ********* ******* ***** *** *
2. Hollow diamond star pattern in Java
The hollow diamond star pattern is another version of the diamond star pattern. It is hollow from the inside.
* * * * * * * * * * * * * * * *
The above pattern shows the hollow diamond star pattern. You can see stars are only at boundaries.
Here are the steps to create the hollow diamond star pattern in Java:
- All the steps of the hollow diamond star pattern are the same as the diamond star pattern but you have to control the printing of stars and spaces here.
- In the loop in which you print star, use conditional statements to print only at boundaries by checking if it's the first or last iteration.
Complete code:
class hollowStarDiamond {
public static void main(String[] args) {
int size = 5;
// upper pyramid
for (int i = 1; i <= size; i++) {
// printing spaces
for (int j = size; j > i; j--) {
System.out.print(" ");
}
// printing star
for (int k = 0; k < i * 2 - 1; k++) {
if (k == 0 || k == 2 * i - 2) {
System.out.print("*");
}
else {
System.out.print(" ");
}
}
System.out.println();
}
// lower triangle
for (int i = 1; i < size; i++) {
// printing spaces
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// printing star
for (int k = (size - i) * 2 - 1; k >= 1; k--) {
if (k == 1 || k == (size - i) * 2 - 1) {
System.out.print("*");
}
else {
System.out.print(" ");
}
}
System.out.println();
}
}
}
Output:
* * * * * * * * * * * * * * * *
3. Number diamond pattern
The number diamond pattern is also a diamond pattern but it is made up of numbers.
1 123 12345 1234567 123456789 1234567 12345 123 1
You can see above the diamond pattern using numbers, the numbers start from 1 in each row and increase by 1.
Steps to create the number diamond pattern in Java:
- Follow the same logic as you used in the diamond star pattern.
- To print numbers we have to track the number, to do this create a variable called count and initialize it with 1. (you can name something else)
- Replace the star (as in diamond star pattern) with the number and increment the number by 1. (you can use count++)
- At the end of each internal loop reset the count to 1 and break the line
class numberDiamond {
public static void main(String[] args) {
int size = 5;
int count = 1;
// upper pyramid
for (int i = 1; i <= size; i++) {
// printing spaces
for (int j = size; j > i; j--) {
System.out.print(" ");
}
// printing star
for (int k = 0; k < i * 2 - 1; k++) {
System.out.print(count++);
}
// set the number to 1
count = 1;
System.out.println();
}
// lower pyramid
for (int i = 1; i <= size - 1; i++) {
// printing spaces
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// printing star
for (int k = (size - i) * 2 - 1; k > 0; k--) {
System.out.print(count++);
}
// set count to 1
count = 1;
System.out.println();
}
}
}
Output:
1 123 12345 1234567 123456789 1234567 12345 123 1
4. Hollow number diamond pattern
The hollow number diamond pattern is the same as the above pattern but hollow inside.
1 1 2 1 2 1 2 1 2 1 2 1 2 1 2 1
You can see above the hollow number diamond pattern. Since numbers are only at boundaries so it uses only 1 and 2 (if you reset the count to 1 in each row).
To create this follow the same logic as you used in the hollow diamond star pattern and replace the star with a count variable and increment it by 1.
class hollowDiamond {
public static void main(String[] args) {
int size = 5;
int count = 1;
// upper pyramid
for (int i = 1; i <= size; i++) {
// printing spaces
for (int j = size; j > i; j--) {
System.out.print(" ");
}
// printing star
for (int k = 0; k < i * 2 - 1; k++) {
if (k == 0 || k == 2 * i - 2) {
System.out.print(count++);
} else {
System.out.print(" ");
}
}
// set the number to 1
count = 1;
System.out.println();
}
// lower triangle
for (int i = 1; i < size; i++) {
// printing spaces
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// printing star
for (int k = (size - i) * 2 - 1; k >= 1; k--) {
if (k == 1 || k == (size - i) * 2 - 1) {
System.out.print(count++);
} else {
System.out.print(" ");
}
}
// set the number to 1
count = 1;
System.out.println();
}
}
}
Output:
1 1 2 1 2 1 2 1 2 1 2 1 2 1 2 1
5. Alphabet diamond pattern
The alphabet diamond pattern is diamond patterns made up of alphabets.
A ABC ABCDE ABCDEFG ABCDEFGHI ABCDEFG ABCDE ABC A
To create an alphabet diamond pattern take the number 65 as a base number and typecast it to char. Example (char)65 => A
.
Follow the same step as in the number diamond pattern but add the base number to 65 and typecast it to char.
Complete code:
class alphabetDiamond {
public static void main(String[] args) {
int size = 5;
int alpha = 65;
int count = 0;
// upper pyramid
for (int i = 1; i <= size; i++) {
// printing spaces
for (int j = size; j > i; j--) {
System.out.print(" ");
}
// printing star
for (int k = 0; k < i * 2 - 1; k++) {
System.out.print((char)(alpha+count++));
}
// set the number to 0
count = 0;
System.out.println();
}
// lower pyramid
for (int i = 1; i <= size - 1; i++) {
// printing spaces
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// printing star
for (int k = (size - i) * 2 - 1; k > 0; k--) {
System.out.print((char)(alpha+count++));
}
// set count to 0
count = 0;
System.out.println();
}
}
}
Output:
A ABC ABCDE ABCDEFG ABCDEFGHI ABCDEFG ABCDE ABC A
6. Hollow alphabet diamond pattern
The hollow alphabet diamond pattern is the same as the above pattern but hollow inside. It is made up of only 2 alphabets 'A' and 'B'.
A A B A B A B A B A B A B A B A
Complete code:
class hollowAlphabetDiamond {
public static void main(String[] args) {
int size = 5;
int alpha = 65;
int count = 0;
// upper pyramid
for (int i = 1; i <= size; i++) {
// printing spaces
for (int j = size; j > i; j--) {
System.out.print(" ");
}
// printing star
for (int k = 0; k < i * 2 - 1; k++) {
if (k == 0 || k == 2 * i - 2) {
System.out.print((char)(alpha+count++));
} else {
System.out.print(" ");
}
}
// set the number to 0
count = 0;
System.out.println();
}
// lower triangle
for (int i = 1; i < size; i++) {
// printing spaces
for (int j = 0; j < i; j++) {
System.out.print(" ");
}
// printing star
for (int k = (size - i) * 2 - 1; k >= 1; k--) {
if (k == 1 || k == (size - i) * 2 - 1) {
System.out.print((char)(alpha+count++));
} else {
System.out.print(" ");
}
}
// set the number to 0
count = 0;
System.out.println();
}
}
}
Output:
A A B A B A B A B A B A B A B A
Conclusion
In this article, we have seen the diamond star pattern in java. We have also seen the number diamond pattern and the alphabet diamond pattern.
You can also look at pattern program in java, star pattern in javascript, or star pattern in python.