Binary to Decimal in Java
In this tutorial, we will look at different Java programs that convert binary to decimal.
- Binary to Decimal Conversion
- Program 1: Using Integer.parseInt()
- Program 2: Using for loop
- Program 3: Using while loop
- Program 4: Using recursion
Example
Binary to Decimal Conversion
A binary number is a number expressed in the base-2 numeral system or binary numeral system, which uses only two symbols: typically "0" (zero) and "1" (one). Each digit is referred to as a bit.
A decimal number is a number expressed in the base-10 numeral system or decimal numeral system, which uses ten symbols: 0, 1, 2, 3, 4, 5, 6, 7, 8, and 9. Each digit is referred to as a place value.
Conversion
Binary to decimal conversion is a simple process. We just need to multiply each digit with its corresponding power of 2 and add them all.
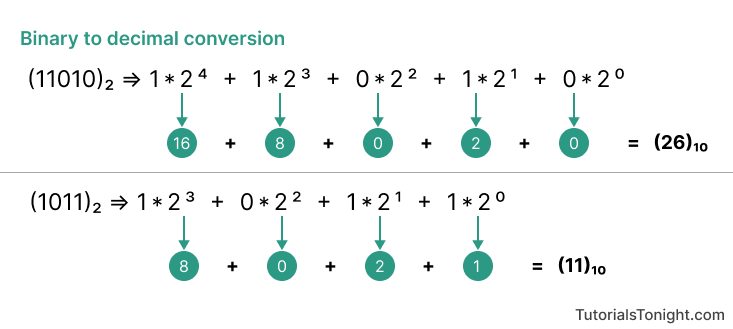
We can represent a binary number as a decimal system as follows:
(1010)2 = (1*23 + 0*22 + 1*21 + 0*20)10 = 1010 (1011)2 = (1*23 + 0*22 + 1*21 + 1*20)10 = 1110 (11001)2 = (1*24 + 1*23 + 0*22 + 0*21 + 1*20)10 = 2510
Program 1: Binary to Decimal Conversion using Integer.parseInt()
Integer.parseInt() method is used to convert a string to an integer. It takes two arguments, the string to be converted and the radix of the string.
The radix is the base of the number system. Here, we are converting a binary number to a decimal number. So, the radix is 2.
Let's see the program:
import java.util.Scanner;
public class main {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.print("Enter a binary number: ");
// input binary string
String binary = s.nextLine();
// convert binary to decimal
int decimal = Integer.parseInt(binary, 2);
System.out.println(decimal);
s.close();
}
}
Output:
Enter a binary number: 100101 37
Program 2: Binary to Decimal using for loop
In this program, we will create a function that takes a binary number as a string and returns its decimal equivalent.
Within the function, we use a for loop to iterate through the string from the right. We multiply each digit with its corresponding power of 2 and add them all.
Let's see the program:
import java.util.Scanner;
public class main {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.print("Enter a binary number: ");
// input binary string
String binary = s.nextLine();
// convert binary to decimal
System.out.println(bin2dec(binary));
s.close();
}
static int bin2dec(String binary) {
int decimal = 0;
int n = 0;
for (int i = binary.length() - 1; i >= 0; i--) {
// if current digit is 1
if (binary.charAt(i) == '1') {
decimal += Math.pow(2, n);
}
n++;
}
return decimal;
}
}
Output:
Enter a binary number: 10110 22
Program 3: Binary to Decimal using while loop
Here we will use the same approach as in the previous program. But, we will use a while loop instead of a for loop.
To work with this we create a variable that keeps track of the current digit. We multiply this variable with its corresponding power of 2 and add them all.
Let's see the program:
import java.util.Scanner;
public class main {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.print("Enter a binary number: ");
// input binary string
String binary = s.nextLine();
// convert binary to decimal
System.out.println(bin2dec(binary));
s.close();
}
static int bin2dec(String binary) {
int decimal = 0;
int n = 0;
int index = binary.length() - 1;
while (index >= 0) {
// if current digit is 1
if (binary.charAt(index) == '1') {
decimal += Math.pow(2, n);
}
n++;
index--;
}
return decimal;
}
}
Output:
Enter a binary number: 10100 20
Getting binary as an integer:
You can also get binary input as an integer. In that case, you can use the following program:
import java.util.Scanner;
public class main {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.print("Enter a binary number: ");
// input binary string
int binary = s.nextInt();
// convert binary to decimal
System.out.println(bin2dec(binary));
s.close();
}
static int bin2dec(int binary) {
int decimal = 0;
int n = 0;
while (binary != 0) {
// if current digit is 1
if (binary % 10 == 1) {
decimal += Math.pow(2, n);
}
n++;
binary /= 10;
}
return decimal;
}
}
Output:
Enter a binary number: 10100 20
Program 4: Binary to Decimal using recursion
In this program, we will use recursion to convert binary to decimal.
import java.util.Scanner;
public class main {
public static void main(String[] args) {
Scanner s = new Scanner(System.in);
System.out.print("Enter a binary number: ");
// input binary integer
int binary = s.nextInt();
// convert binary to decimal
System.out.println(bin2dec(binary));
s.close();
}
static int bin2dec(int binary) {
// base case
if (binary == 0) {
return 0;
}
return (binary % 10) + 2 * bin2dec(binary / 10);
}
}
Output:
Enter a binary number: 110101 53