Palindrome Program In Java
In this article, you will learn how to create a palindrome program in java using multiple different methods for both strings and numbers.
- What is Palindrome?
- String Palindrome Program In Java
- Number Palindrome Program In Java
- Conclusion
Table Of Contents

What is Palindrome?
A Palindrome is a word, number, phrase, or other sequence of characters that reads the same backward or forward. For example, "madam", "racecar", 12321, 2641462, "AToyota" are all palindromes.
The concept of a palindrome is used in computational biology to create and test new genetic sequences like proteins, DNA, and RNA. Palindromes are used to test the integrity of a sequence.
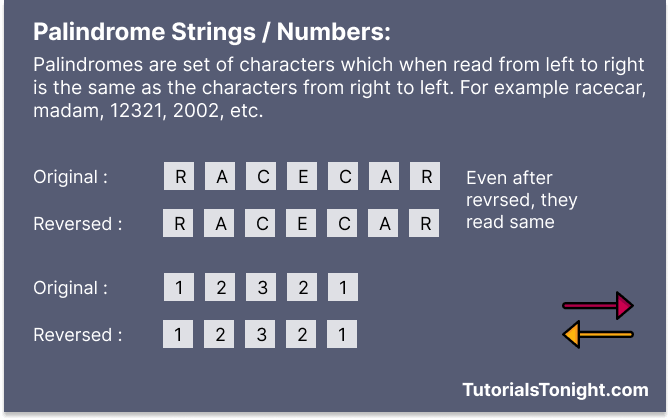
String Palindrome Program In Java
You know now what is a palindrome. Let's create Java programs that will check if a string is a palindrome or not.
There can be many different approaches to create such programs. We have discussed three approaches in this article.
Let's see them all one by one.
Method 1: Find String Palindrome
Any string that is palindrome must have the same characters in reverse order. This means, that the first character must be the same as the last character, the second character must be the same as the second last character, and so on.
So we can take 2 variables, one starts from 0, and the other starts from the last index of the string. We will compare each character of the string with its reverse counterpart. If they are the same, we will move to the next character. If they are not the same, means the string is not a palindrome.
Here is the Java program using this concept:
import java.util.Scanner;
public class palindrome {
static boolean isPalindrome(String s) {
int i = 0;
int j = s.length() - 1;
while (i < j) {
if (s.charAt(i) != s.charAt(j)) {
return false;
}
i++;
j--;
}
return true;
}
public static void main(String[] args) {
// take string as input
Scanner sc = new Scanner(System.in);
System.out.print("Enter the string: ");
String s = sc.nextLine();
// call isPalindrome method
if(isPalindrome(s)) {
System.out.println(s + " is a palindrome");
} else {
System.out.println(s + " is not a palindrome");
}
// close scanner
sc.close();
}
}
Output:
$ java palindrome Enter the string: racecar racecar is a palindrome Enter the string: hello hello is not a palindrome
Code Explanation:
- The program starts by taking input from the user.
- We call isPalindrome() method and pass a string as a parameter.
- The isPalindrome() method returns true if the string is palindrome otherwise false.
- Within the method, take 2 variables, one starts from 0, and the other starts from the last index of the string.
- Execute a while loop until i is less than j. Check if characters at index i and j are the same. If they are the same, increment i and decrement j. If they are not the same, return false.
- If the loop is executed completely, return true.
- Finally, print whether the string is a palindrome or not.
Method 2: Reverse String
In this method, we are going to reverse the string and then compare it with the original string using the equals() method. If they are the same, then the string is a palindrome.
import java.util.Scanner;
public class palindrome {
static boolean isPalindrome(String s) {
String reversed = "";
for (int i = s.length() - 1; i >= 0; i--) {
reversed += s.charAt(i);
}
return reversed.equals(s);
}
public static void main(String[] args) {
// take string as input
Scanner sc = new Scanner(System.in);
System.out.print("Enter the string: ");
String s = sc.nextLine();
// call isPalindrome method
if(isPalindrome(s)) {
System.out.println(s + " is a palindrome");
} else {
System.out.println(s + " is not a palindrome");
}
// close scanner
sc.close();
}
}
Output:
$ java palindrome Enter the string: racecar racecar is a palindrome Enter the string: hello hello is not a palindrome
Code Explanation:
- Start the program by taking user input.
- Call the isPalindrome() method by passing a string as a parameter.
- The method creates an empty string. Then run a for loop from the last index to 0 and append each character to the string.
- Finally, check if the string is equal to reversed string. If they are the same, then the string is a palindrome.
Method 3: Recursive Method
Here is a recursive method to check if the string is a palindrome or not.
import java.util.Scanner;
public class palindrome {
static boolean isPalindrome(String s) {
if (s.length() <= 1) {
return true;
}
if (s.charAt(0) != s.charAt(s.length() - 1)) {
return false;
}
// remove first and last char
s = s.substring(1, s.length() - 1);
return isPalindrome(s);
}
public static void main(String[] args) {
// take string as input
Scanner sc = new Scanner(System.in);
System.out.print("Enter the string: ");
String s = sc.nextLine();
// call isPalindrome method
if(isPalindrome(s)) {
System.out.println(s + " is a palindrome");
} else {
System.out.println(s + " is not a palindrome");
}
// close scanner
sc.close();
}
}
Output:
$ java palindrome Enter the string: madam madam is a palindrome Enter the string: world world is not a palindrome
Code Explanation:
- The program uses recursion to check if the string is a palindrome or not.
- If the string length is less than or equal to 1, then return true.
- If the first and last characters are not the same, then return false.
- Remove the first and the last character and call the method again.
Number Palindrome Program In Java
Unlike string, you can't access individual characters of a number. You can only access the whole number. So, we have to apply some logic to get individual characters of a number.
Here are a few methods to find if a number is palindrome or not:
Method 1: Number Palindrome
One of the simplest ways is to create another number by reversing the original number and then comparing it with the original number.
import java.util.Scanner;
public class palindrome {
static boolean isPalindrome(int n) {
int reversed = 0;
int temp = n;
while (temp > 0) {
reversed = reversed * 10 + temp % 10;
temp /= 10;
}
return reversed == n;
}
public static void main(String[] args) {
// take string as input
Scanner sc = new Scanner(System.in);
System.out.print("Enter the number: ");
int num = sc.nextInt();
// call isPalindrome method
if(isPalindrome(num)) {
System.out.println(num + " is a palindrome");
} else {
System.out.println(num + " is not a palindrome");
}
// close scanner
sc.close();
}
}
Output:
$ java palindrome Enter the number: 1221 1221 is a palindrome Enter the number: 1234 1234 is not a palindrome
Code Explanation:
- Start the program by taking user input.
- Logic is to create another number by reversing the original number and then comparing it with the original number.
- For this create a variable and assign 0 to it.
- Run a while loop and multiply the variable by 10 and add the remainder of the original number to it. Divide the original number by 10.
- Finally, check if the variable is equal to the original number. If they are the same, then the number is a palindrome.
Method 2: Number Palindrome
Another simple approach could be to convert the number to string and apply any of the above string palindrome methods.
import java.util.Scanner;
public class palindrome {
static boolean isPalindrome(int n) {
String s = String.valueOf(n);
for (int i = 0; i < s.length() / 2; i++) {
if (s.charAt(i) != s.charAt(s.length() - 1 - i)) {
return false;
}
}
return true;
}
public static void main(String[] args) {
// take string as input
Scanner sc = new Scanner(System.in);
System.out.print("Enter the number: ");
int num = sc.nextInt();
// call isPalindrome method
if(isPalindrome(num)) {
System.out.println(num + " is a palindrome");
} else {
System.out.println(num + " is not a palindrome");
}
// close scanner
sc.close();
}
}
Output:
$ java palindrome Enter the number: 1234321 1234321 is a palindrome Enter the number: 1234 1234 is not a palindrome
Conclusion
A palindrome is a simple pattern of a word or a number that reads the same backward as forward. You can imagine sitting in the middle of a string or number, both sides of the string or number will be same.
Above we have seen 5 palindrome program in Java using different approaches. Don't stop learning here check these out: Happy number in Java, Lucky number in java.