Python Octal to Decimal Conversion
In this article, we will look at 4 different ways to convert octal to decimal in Python.
Octal and Decimal Numbers
Octal Numbers
Octal numbers are base 8 numbers. They are represented by the digits 0 to 7. The digits 8 and 9 are not used in octal numbers. Octal numbers are used in computer programming and computer hardware.
8 will be represented as 10 in octal, 9 will be represented as 11 in octal, and so on.
Decimal Numbers
Decimal numbers are base 10 numbers. They are represented by the digits 0 to 9. Decimal numbers are used in everyday life.
Octal to Decimal Conversion
Octal to decimal conversion is the process of converting octal numbers to decimal numbers. The process of octal to decimal conversion is similar to binary to decimal conversion. The only difference is that the base is 8 instead of 2.
Multiply each digit of the octal number with the corresponding power of 8. Then add all the products to get the decimal number.
Look at the image below to understand the process of octal to decimal conversion.
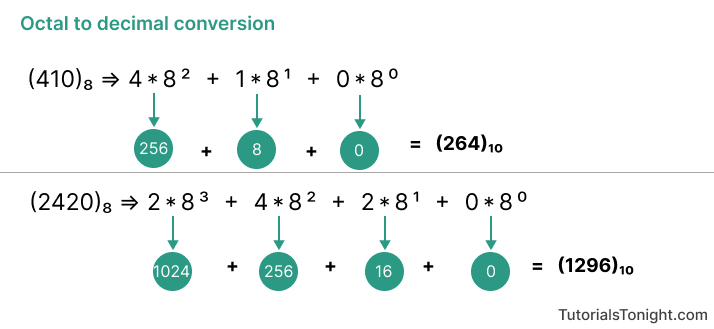
Method 1: Using while loop
To convert octal to decimal in Python, we can use a while loop and start from the rightmost digit. We can multiply each digit with the corresponding power of 8 and add all the products to get the decimal number.
Algorithm
- Initialize dec to 0 and i to 0. Here dec is the decimal number and i is the power of 8.
- Run a while loop until oct is greater than 0.
- Inside the while loop, add the product of oct%10 and 8**i to dec.
- Divide oct by 10 and increment i by 1.
- Return dec.
# function to convert octal to decimal
def oct2dec(oct):
dec = 0
i = 0
while oct > 0:
dec += oct%10 * (8**i)
oct //= 10
i += 1
return dec
num = input("Enter a octal number: ")
print(oct2dec(num))
Output:
Enter a octal number: 155 109
Method 2: Using for loop
We can also use for loop in a similar way.
To work with for loop we need to know the number of iterations in advance. Convert the octal number to a string using str() function. Then use len() function to get the number of digits in the octal number.
Algorithm
- Initialize dec to 0 and i to 0. Here dec is the decimal number and i is the power of 8.
- Convert oct to string using str() function.
- Get the number of digits in oct using len() function.
- Run a for loop from 0 to the number of digits in oct.
- Inside the for loop, add the product of oct[i] and 8**i to dec.
- Return dec.
# function to convert octal to decimal
def oct2dec(oct):
dec = 0
i = 0
oct = str(oct)
for i in range(len(oct)):
dec += int(oct[i]) * (8**i)
return dec
num = input("Enter a octal number: ")
print(oct2dec(num))
Output:
Enter a octal number: 215 141
Method 3: Recursive function
Using recursion you can solve a problem that can be solved by solving a smaller version of the same problem.
For octal to decimal conversion, we can use recursion to solve the problem.
Algorithm
- If oct is 0, return 0.
- Else, return the sum of oct%10 and 8 * oct2dec(oct // 10).
# recursive function to convert octal to decimal
def oct2dec(oct):
if oct == 0:
return 0
else:
return (oct%10) + 8 * oct2dec(oct // 10)
num = input("Enter a octal number: ")
print(oct2dec(num))
Output:
Enter a octal number: 65 53