jQuery Add Class
Adding class programmatically give us the power to manipulate the HTML elements and create interactive web experiences, animations, and responsive designs.
In this tutorial, you will learn how to add class using jQuery and anything around it.
- jQuery addClass() Method
- Add Multiple Classes
- Add Class if not Exists
- Add Class on Click
- Add Class with Delay
- Conclusion
Table of Contents
1. jQuery addClass() Method
To add class using jQuery you can use the addClass() method.
Call the addClass() method on the element you want to add the class to and pass the class name as an argument to the method.
For example, if you want to add the class active to the element with id elementID, you can do it like this:
Example
let element = $("#elementID");
// Add the class "active" to the element
element.addClass("active");
2. Add Multiple Classes
The addClass() method can also be used to add multiple classes to an element. Just pass the multiple class names separated by space as an argument to the method.
For example, if you want to add the classes active and big to the element with id elementID, you can do it like this:
Example
let element = $("#elementID");
// Add the classes "active" and "big" to the element
element.addClass("active big");
3. Add Class if not Exists
jQuery addClass() method adds the class to the element even if the element already has the class. If you want to add the class only if the element doesn't have the class, you can use the hasClass() method to check if the element has the class or not.
Here is an example for the same:
Example
let element = $("#elementID");
// Check if the element has the class "active"
if(!element.hasClass("active")) {
// Add the class "active" to the element
element.addClass("active");
}
4. Add Class on Click
Dynamically changing class is most used case of why we add class programmatically. Let's see how we can add class on click of a button.
- Select the element on which you want to add the class.
- Use the click() method to add a click event listener to the element.
- Inside the click event listener, add the class to the element using the addClass() method.
Example
let element = $("#elementID");
let button = $("#buttonID");
// Add click event listener to the button
button.click(function() {
// Add the class "active" to the element
element.addClass("active");
});
Output:
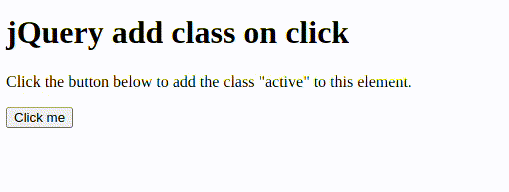
5. Add Class with Delay
Adding class with delay is useful when you want to perform some animation or transition on the element.
Let's see how we can add class with delay.
Here is an example for the same:
Example
let element = $("#elementID");
let button = $("#buttonID");
// Add click event listener to the button
button.click(function() {
// Add the class "active" to the element after 1 second
setTimeout(function() {
element.addClass("active");
}, 1000);
});
Output:

Conclusion
Summing up, use the addClass() method to add class. Pass the class name as an argument to the method.
To add multiple classes, pass the multiple class names separated by space as an argument to the method.
Happy Learning!