Random Color Generator JavaScript
Random Color Generator JavaScript is a simple project for beginners to learn JavaScript. In this project, we will learn how to generate random colors using JavaScript.
Once we generate the random colors, we will use them as background colors for the page, and also as the text color for the page. This will look really cool😎.
We also have a tool to generate random colors when you generate random colors and can copy these colors in different formats like HEX, RGB, and HSL.
Prerequisites
Here is what our project will look like:
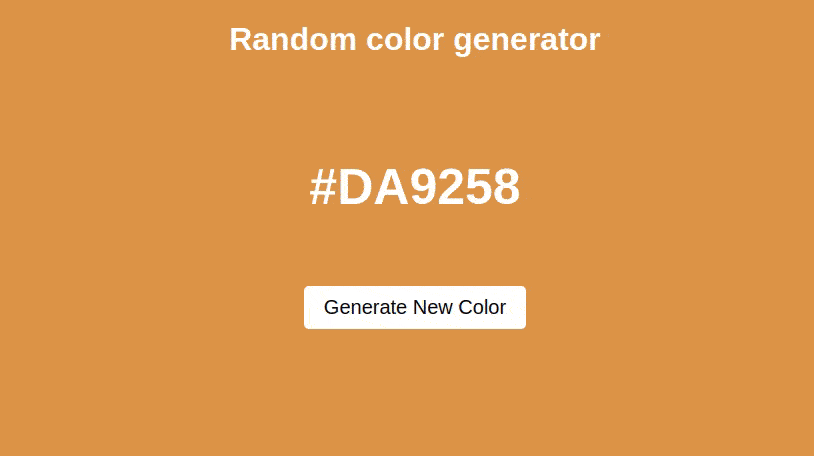
You can also use this color format in the project.
Random Color Generator Project
Let's start with the random color generator project.
The interface of our project is going to be very simple. It will have a button and text. The button will be used to generate the random colors, and the text will be used to display the random colors.
Browser support different color formats like HEX, RGB, and HSL.
1. HTML
Let's start with the HTML part of our project.
Use <h1> tag and give a heading to our project.
Create a div with the class color-generator. This div will contain the button and the text.
Add an onclick event to the button. This event will call the generateColor() function.
<h1>Random color generator</h1>
<div class="color-generator">
<p id="color"></p>
<button onclick="randomColor()">Generate New Color</button>
</div>
2. CSS
Let's style our project.
Target elements in the HTML part and style them using CSS properties like background, margin, padding, colors, transitions, etc.
Explore different CSS properties and draw your imagination on the screen.
Meanwhile here is the CSS style used by us.
body {
background-color: #000000;
color: #ffffff;
font-family: sans-serif;
text-align: center;
}
.color-generator {
margin-top: 100px;
}
#color {
font-size: 50px;
font-weight: bold;
}
button {
background-color: #ffffff;
color: #000000;
font-size: 20px;
padding: 10px 20px;
border: none;
border-radius: 5px;
margin-top: 20px;
transition: 0.5s ease-in-out;
cursor: pointer;
}
button:hover {
transform: scale(1.1)
}
button:active {
transform: scale(.9)
}
3. JavaScript
Here comes the main part of the project. Let's see how to generate random colors.
Before you generate a random number, first know how to generate random number in JavaScript. Use the Math.random() function, it returns a random number between 0 and 1.
Here is the generateColor() function that generates a random color in HEX format.
function generateColor() {
let color = '#';
let digits = '0123456789ABCDEF';
for (let i = 0; i < 6; i++) {
// generate a random number between 0 and 15
let randomDigit = Math.floor(Math.random() * 16);
// append the random number to the color string
color += digits[randomDigit];
}
console.log(color);
}
generateColor();
In the above function, we start with a string with the value '#' (for HEX color). Then we create a string with all the digits and letters that can be used in a HEX color code.
Then we use a for loop to generate a random number between 0 and 15. And then used this random number to get a random digit or letter from the digits string.
Then we append this random digit or letter to the color string. And finally, we have a random color in HEX format.
In this function, we need to add 2 more lines of code to display the random color on the screen and change the background color of the button.
document.getElementById('color').innerHTML = color;
document.querySelector('button').style.backgroundColor = color;
Here is the complete code of our project.
Complete Project Code
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Random color generator</title>
<style>
body {
background-color: #000000;
color: #ffffff;
font-family: sans-serif;
text-align: center;
}
.color-generator {
margin-top: 100px;
}
#color {
font-size: 50px;
font-weight: bold;
}
button {
background-color: #ffffff;
color: #000000;
font-size: 20px;
padding: 10px 20px;
border: none;
border-radius: 5px;
margin-top: 20px;
transition: 0.5s ease-in-out;
cursor: pointer;
}
button:hover {
transform: scale(1.1)
}
button:active {
transform: scale(.9)
}
</style>
</head>
<body>
<h1>Random color generator</h1>
<div class="color-generator">
<p id="color"></p>
<button onclick="generateColor()">Generate New Color</button>
</div>
<script>
function generateColor() {
let color = '#';
let digits = '0123456789ABCDEF';
for (let i = 0; i < 6; i++) {
// generate a random number between 0 and 15
let randomDigit = Math.floor(Math.random() * 16);
// append the random number to the color string
color += digits[randomDigit];
}
// set the text value and background color to the random color
document.getElementById('color').innerHTML = color;
document.body.style.backgroundColor = color;
}
// call the function when the page loads
randomColor();
</script>
</body>
</html>
Generating Random Colors in RGB Format
Now let's see how to generate random colors in RGB format
The RGB format is used to define colors in CSS. It is a combination of 3 numbers between 0 and 255. Each number represents the amount of red, green, and blue in the color. For example, the color rgb(255, 0, 0) is red, because it has 255 red, 0 green, and 0 blue.
Here is the generateColor() function that generates a random colors in RGB format.
function generateColor() {
let red, green, blue;
red = Math.floor(Math.random() * 256);
green = Math.floor(Math.random() * 256);
blue = Math.floor(Math.random() * 256);
let color = `rgb(${red}, ${green}, ${blue})`;
console.log(color);
}
generateColor();
Converting HEX to RGB
To convert HEX to RGB, we need to use the following formula:
RGB = HEX * 16 + HEX
For example, if the HEX value is FF, the RGB value will be 255. i.e FF * 16 = 240, and 240 + FF = 255.
Here is the hexToRgb() function that converts HEX to RGB.
function hexToRgb(hex) {
let red, green, blue;
red = parseInt(hex[1] + hex[2], 16);
green = parseInt(hex[3] + hex[4], 16);
blue = parseInt(hex[5] + hex[6], 16);
let color = `rgb(${red}, ${green}, ${blue})`;
console.log(color);
}
hexToRgb('#FF0000');
Converting RGB to HEX
To convert RGB to HEX, we need to use the following formula:
HEX = RGB / 16
Here is the rgbToHex() function that converts RGB to HEX.
function rgbToHex(rgb) {
let red, green, blue;
// rgb color values are separated by commas
// looks like rgb(255, 0, 0)
// first we need to remove the rgb( and )
rgb = rgb.replace('rgb(', '');
rgb = rgb.replace(')', '');
// then we need to split the string by commas
rgb = rgb.split(',');
red = parseInt(rgb[0]);
green = parseInt(rgb[1]);
blue = parseInt(rgb[2]);
// convert the RGB values to hex
red = red.toString(16);
green = green.toString(16);
blue = blue.toString(16);
// make sure the hex values are 2 digits
if (red.length == 1) {
red = '0' + red;
}
if (green.length == 1) {
green = '0' + green;
}
if (blue.length == 1) {
blue = '0' + blue;
}
let color = `#${red}${green}${blue}`;
console.log(color);
}
rgbToHex('rgb(45, 185, 50)');
Conclusion
The main takeaway from this tutorial is that you can generate random colors in HEX or RGB format using JavaScript. You can also convert HEX to RGB and RGB to HEX using JavaScript.
In this tutorial, we have generated random colors and used them to change the page's background color. You can use this technique to create a random color generator.