Hexadecimal to Decimal in Python
In this tutorial, you will learn 3 different ways to convert hexadecimal to decimal in python.
Hexadecimal to Decimal Conversion
Hexadecimal numbers are base 16 numbers, means they are made up of 16 different digits. The digits are 0-9 & A-F.
Decimal numbers are base 10 numbers, that we use in our daily life.
Any hexadecimal number can be converted to decimal number. To convert hexadecimal to decimal, we need to multiply each digit with its respective power of 16.
For example, let's convert 2A0 to decimal:
2A0 = 2 * 162 + 10 * 161 + 0 * 160
In the above example, you can see the decimal equivalent of 'A' (10) is used while converting to decimal.
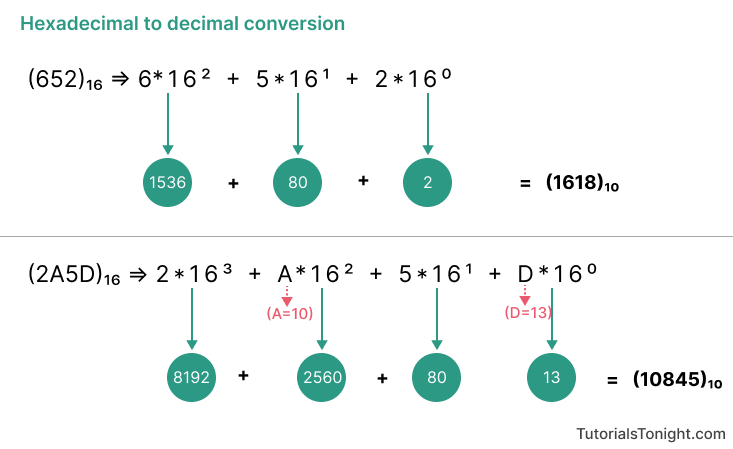
Method 1: Using int() function
The first and easiest way to convert hexadecimal to decimal is by using int() function.
The int() function can convert any number to decimal. It takes two arguments, the number to be converted (as string) and the base of the passing number.
# convert hexadecimal to decimal
hex_num = '65'
# using int() function
dec_num = int(hex_num, 16)
print(dec_num)
Output:
101
Method 2: Using custom function
We have discussed above how you can convert a hexadecimal number to a decimal.
What we did was, we multiplied each digit's decimal value with its respective power of 16 and added them.
Same thing we are going to do using Python programming.
Steps to convert hexadecimal to a decimal using the custom function:
- Create variable dec to store decimal value and multiplier to store power of 16 which will be used to multiply with each digit.
- Loop through each digit of the hexadecimal number in reverse order, or if you loop in the forward direction then get digits from the end.
- Convert each digit to a decimal value and multiply it with its respective power of 16.
- Add the result to dec variable.
- Return the dec variable.
# convert hexadecimal to decimal
# using custom function
def hex2dec(hex):
# create variable dec & multiplier
dec = 0
multiplier = 1
length = len(hex)
# loop through each digit
for i in range(length):
# get digit from end
digit = hex[length - i - 1]
digit = digit.upper()
# if digit is between 'A' and 'F'
if digit >= 'A' and digit <= 'F':
digit = ord(digit) - 55
else:
digit = int(digit)
dec += digit * multiplier
multiplier *= 16
return dec
# get hexadecimal number
hex_num = '7B2C'
# call function
print(hex2dec(hex_num))
Output:
31532
Method 3: Recursive function
Let's create a recursive function that converts hexadecimal to decimal in Python.
Steps to convert hexadecimal to a decimal using the recursive function:
- Get the last digit of the hexadecimal number.
- Convert the last digit to a decimal value.
- If the length of the hexadecimal number is 1, then return the decimal value of the last digit.
- Otherwise, return the decimal value of the last digit plus 16 times the function call with the hexadecimal number without the last digit.
# convert hexadecimal to decimal
# using recursive function
def hex2dec(hex):
digit = hex[-1]
digit = digit.upper()
# if digit is between 'A' and 'F'
if digit >= 'A' and digit <= 'F':
digit = ord(digit) - 55
else:
digit = int(digit)
if len(hex) == 1:
return digit
else:
return digit + 16 * hex2dec(hex[:-1])
# get hexadecimal number
hex_num = '642E'
# call function
print(hex2dec(hex_num))
Output:
25646
Conclusion
You have looked at 3 different methods to convert hexadecimal to decimal in Python. You can use any of the methods as per your requirement.
Using a built-in function is easy but creating a custom function is where you learn the logic behind the conversion.
Happy Coding!😊