Anagram Program In Python
In this article, we are going to anagram program in Python. The programs will compare two words or phrases and check if they are anagrams or not.
- What is Anagram?
- Check if Two Strings are Anagrams
- Conclusion
Table Of Contents
What is Anagram?
An anagram is a word or phrase created by rearranging the letters of another word or phrase.
For example, the word "heart" can be rearranged to form the word "earth". So, "heart" and "earth" are anagrams of each other.
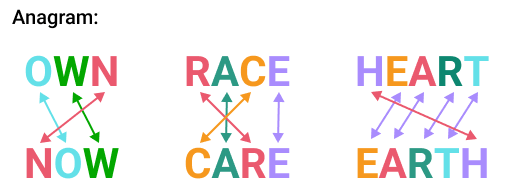
Check if Two Strings are Anagrams
There can be many different ways to check if two strings are anagrams. Here, we are going to see 4 different ways and their implementations.
1. By Sorting
In this method, we will sort the letters of both strings and then compare them. If both the strings are anagrams, then both the strings will be equal after sorting.
For example:
"heart" => sorted => "aehrt" "earth" => sorted => "aehrt" Both strings are equal after sorting. So, they are anagrams of each other.
Let's see the implementation of this method in Python.
def check_anagram(str1, str2):
# Remove all spaces from both the strings
# and convert them to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if the length of both the strings are equal
if len(str1) != len(str2):
return False
else:
# Sort both the strings
str1 = sorted(str1)
str2 = sorted(str2)
# Compare both the strings
for i in range(len(str1)):
if str1[i] != str2[i]:
return False
return True
# Driver code
str1 = "heart"
str2 = "earth"
if check_anagram(str1, str2):
print("The strings are anagrams.")
else:
print("The strings aren't anagrams.")
Output:
The strings are anagrams.
2. By Counting Characters
In this method, we will count the number of times each character appears in both strings. If both the strings are anagrams, then the number of times each character appears in both strings will be equal.
For example:
"heart" => count => {'h': 1, 'e': 1, 'a': 1, 'r': 1, 't': 1} "earth" => count => {'e': 1, 'a': 1, 'r': 1, 't': 1, 'h': 1} Both the strings have the same number of times each character appears. So, they are anagrams of each other.
Algorithm:
- Remove all spaces from both strings.
- Check if the length of both strings is equal. If not, then they are not anagrams.
- Create empty dictionaries to store the count of each character in both strings.
- Compare both dictionaries. If both dictionaries are equal, then the strings are anagrams.
Execute a for loop for each character in both the strings and create entries in the dictionaries.
Let's see the implementation of this method in Python.
def check_anagram(str1, str2):
# Remove all spaces from both the strings
# and convert them to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if the length of both the strings are equal
if len(str1) != len(str2):
return False
else:
# Count the number of times each character appears in both the strings
count1 = {}
count2 = {}
for i in str1:
if i in count1:
count1[i] += 1
else:
count1[i] = 1
for i in str2:
if i in count2:
count2[i] += 1
else:
count2[i] = 1
# Compare both dictionaries
if count1 == count2:
return True
else:
return False
# Driver code
str1 = "listen"
str2 = "silent"
if check_anagram(str1, str2):
print("The strings are anagrams.")
else:
print("The strings aren't anagrams.")
Output:
The strings are anagrams.
3. By Using Dictionary
In this method, we will create a dictionary to store the count of each character in the first string. Then, we will traverse the second string and check if the character is present in the dictionary. If the character is present, then we will decrease the count of that character by 1. If the character is not present, then we will return False.
Finally, we will check if all the values in the dictionary are 0. If yes, then the strings are anagrams.
Let's see the Python program for this method.
def check_anagram(str1, str2):
# Remove all spaces from both the strings
# and convert them to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if the length of both the strings are equal
if len(str1) != len(str2):
return False
# Create a dictionary to store the count
# of each character in the first string
count = {}
for i in str1:
if i in count:
count[i] += 1
else:
count[i] = 1
# Traverse the second string and
# check if all characters have 0 count
for i in str2:
if i in count:
count[i] -= 1
else:
return False
# Check if the dictionary is empty
for i in count:
if count[i] != 0:
return False
return True
# Driver code
str1 = "binary"
str2 = "brainy"
if check_anagram(str1, str2):
print("The strings are anagrams.")
else:
print("The strings aren't anagrams.")
Output:
The strings are anagrams.
4. Replace Each Character with Empty String
Another way to find if two strings are anagrams is to replace each character of the first string with an empty string in the second string. If the second string is empty, then the strings are anagrams.
For example:
1st string: "own" 2nd string: "now" Replace "o" with "" in the 2nd string => "nw" Replace "w" with "" in the 2nd string => "n" Replace "n" with "" in the 2nd string => "" Since the 2nd string is empty, the strings are anagrams.
Let's see the implementation of this method in Python.
def check_anagram(str1, str2):
# Remove all spaces from both the strings
# and convert them to lowercase
str1 = str1.replace(" ", "").lower()
str2 = str2.replace(" ", "").lower()
# Check if the length of both the strings are equal
if len(str1) != len(str2):
return False
# Replace each character of the first string
# with an empty string in the second string
for i in str1:
str2 = str2.replace(i, "", 1)
# Check if the second string is empty
if str2 == "":
return True
else:
return False
# Driver code
str1 = "own"
str2 = "now"
if check_anagram(str1, str2):
print("The strings are anagrams.")
else:
print("The strings aren't anagrams.")
Output:
The strings are anagrams.
Conclusion
We have seen 4 different ways to tell if two strings are anagrams in Python. You can use any of these methods as per your requirement.
Reference: Wikipedia